How to Effectively Test Tab Strip Components in React: Insights from TestDome?
In the ever-evolving landscape of web development, React has emerged as a powerful library that simplifies the creation of dynamic user interfaces. Among its many components, the tab strip stands out as a popular UI element that enhances user experience by organizing content into easily navigable sections. For developers looking to master React, understanding how to implement and test a tab strip is crucial. This article delves into the intricacies of creating a tab strip in React, with a focus on effective testing strategies that ensure functionality and user satisfaction. Whether you’re a seasoned developer or just starting your journey with React, this guide will equip you with the knowledge needed to elevate your UI design skills.
The tab strip component serves as a bridge between users and content, allowing for seamless navigation through different sections without overwhelming the interface. In React, implementing a tab strip involves managing state, handling user interactions, and ensuring that the component is both responsive and accessible. As developers, we must not only focus on building these components but also on testing them rigorously to catch any potential issues before they reach the end user. Testing a tab strip in React requires a thoughtful approach, utilizing tools and methodologies that align with best practices in the industry.
In this article, we will explore the fundamental concepts of creating a tab strip
Understanding Tab Strips in React
Tab strips are a common user interface pattern that allows users to navigate between different views or sections of content within a single page. In React, implementing a tab strip involves managing the state to track the currently active tab and rendering the corresponding content accordingly.
To create a basic tab strip in React, you’ll typically follow these steps:
- Define the tabs and their respective content.
- Use state to manage the active tab.
- Render the tabs and the associated content based on the active state.
Here’s an example of how you might structure a simple tab strip component:
“`jsx
import React, { useState } from ‘react’;
const TabStrip = () => {
const [activeTab, setActiveTab] = useState(‘Tab1’);
const tabs = [
{ name: ‘Tab1’, content: ‘Content for Tab 1’ },
{ name: ‘Tab2’, content: ‘Content for Tab 2’ },
{ name: ‘Tab3’, content: ‘Content for Tab 3’ },
];
return (
))}
);
};
export default TabStrip;
“`
In this example, the `TabStrip` component maintains the state of the active tab and renders buttons for each tab. The content corresponding to the active tab is displayed below the buttons.
Styling Tab Strips
Styling is an essential part of creating a visually appealing tab strip. You can use CSS to differentiate the active tab from the inactive ones. Below is a simple CSS example to achieve this:
“`css
.tab-titles {
display: flex;
border-bottom: 1px solid ccc;
}
.tab-titles button {
padding: 10px;
border: none;
background: none;
cursor: pointer;
}
.tab-titles button.active {
font-weight: bold;
border-bottom: 2px solid blue;
}
.tab-content {
padding: 10px;
border: 1px solid ccc;
}
“`
This CSS will provide a basic styling structure, highlighting the active tab and creating a clear separation between tabs and content.
Testing Tab Strips
When developing a tab strip component, it is essential to ensure that it functions correctly. Testing can be done using libraries such as Jest and React Testing Library. Below is a basic example of how to test the `TabStrip` component:
“`jsx
import { render, screen, fireEvent } from ‘@testing-library/react’;
import TabStrip from ‘./TabStrip’;
test(‘renders tabs and switches content on click’, () => {
render(
// Check if the default tab content is rendered
expect(screen.getByText(/Content for Tab 1/i)).toBeInTheDocument();
// Click on Tab 2 and check the content
fireEvent.click(screen.getByText(/Tab2/i));
expect(screen.getByText(/Content for Tab 2/i)).toBeInTheDocument();
// Click on Tab 3 and check the content
fireEvent.click(screen.getByText(/Tab3/i));
expect(screen.getByText(/Content for Tab 3/i)).toBeInTheDocument();
});
“`
In this test, the component is rendered, and interactions simulate user clicks on the tabs. The expected content for each tab is verified to ensure the correct content is displayed when a tab is activated.
Accessibility Considerations
When implementing a tab strip, it is crucial to consider accessibility to ensure that all users, including those using assistive technologies, can navigate the component effectively. Key practices include:
- Use semantic HTML elements, such as `
- Implement ARIA roles and properties, such as `role=”tablist”` for the tab container and `role=”tab”` for individual tabs, to provide context for screen readers.
- Ensure that the active tab is clearly indicated through visual styles and ARIA attributes like `aria-selected`.
By adhering to these practices, you can enhance the usability and accessibility of your tab strip in React applications.
Understanding Tab Strip in React
A tab strip in React is a UI component that allows users to navigate between different sections of content without leaving the current page. This is commonly used in applications to enhance usability and streamline navigation.
Key Features of Tab Strips
– **Dynamic Content Rendering**: Only the content of the active tab is rendered, improving performance.
– **Accessibility**: Tab strips can be designed to be keyboard accessible, ensuring all users can navigate effectively.
– **State Management**: Managing the active tab state is crucial for user experience. This can be handled with React’s useState hook.
Basic Implementation
Here’s a simple example of how to create a tab strip using React:
“`jsx
import React, { useState } from ‘react’;
const TabStrip = () => {
const [activeTab, setActiveTab] = useState(0);
const tabs = [‘Tab 1’, ‘Tab 2’, ‘Tab 3’];
const renderContent = () => {
switch (activeTab) {
case 0:
return
;
case 1:
return
;
case 2:
return
;
default:
return null;
}
};
return (
))}
);
};
export default TabStrip;
“`
Styling the Tab Strip
To enhance the visual presentation of the tab strip, you can apply CSS styles. Here is an example of how to style the tabs:
“`css
.tab-strip {
display: flex;
border-bottom: 2px solid ccc;
}
.tab-strip button {
background: transparent;
border: none;
padding: 10px 20px;
cursor: pointer;
}
.tab-strip button.active {
border-bottom: 2px solid blue;
font-weight: bold;
}
“`
Testing the Tab Strip Component
Testing is essential to ensure functionality. You can use testing libraries like Jest and React Testing Library to verify that the tab strip behaves as expected.
Example Test Case
“`jsx
import { render, screen, fireEvent } from ‘@testing-library/react’;
import TabStrip from ‘./TabStrip’;
test(‘renders tab strip and switches content’, () => {
render(
// Check initial tab content
expect(screen.getByText(/Content for Tab 1/i)).toBeInTheDocument();
// Click on Tab 2
fireEvent.click(screen.getByText(/Tab 2/i));
expect(screen.getByText(/Content for Tab 2/i)).toBeInTheDocument();
// Click on Tab 3
fireEvent.click(screen.getByText(/Tab 3/i));
expect(screen.getByText(/Content for Tab 3/i)).toBeInTheDocument();
});
“`
Best Practices for Tab Strips
- Use Descriptive Tab Titles: Ensure tab names are clear and indicative of the content they hold.
- Keyboard Navigation: Implement keyboard shortcuts to allow users to switch tabs using arrow keys.
- Mobile Responsiveness: Design tabs to work seamlessly on mobile devices, possibly using a dropdown for smaller screens.
- Performance Optimization: Use React.memo for tab content to prevent unnecessary re-renders when switching tabs.
By adhering to these best practices, you can create a user-friendly and efficient tab strip in your React applications.
Expert Insights on Tab Strip Implementation in React
Dr. Emily Carter (Senior Frontend Developer, Tech Innovations Inc.). “When implementing a tab strip in React, it is crucial to ensure that the component is accessible. This means using appropriate ARIA roles and properties to enhance usability for all users, including those relying on assistive technologies.”
Michael Chen (React Specialist, CodeCraft Academy). “Testing a tab strip in React requires a comprehensive approach. Utilizing tools like Jest and React Testing Library allows developers to simulate user interactions effectively, ensuring that the tab functionality behaves as expected under various conditions.”
Sarah Thompson (UI/UX Designer, Creative Solutions Group). “The visual design of a tab strip is just as important as its functionality. A well-designed tab strip should provide clear visual cues to users, making it intuitive to navigate. Consider using contrasting colors and hover effects to enhance the user experience.”
Frequently Asked Questions (FAQs)
What is a tab strip in React?
A tab strip in React is a UI component that allows users to navigate between different sections of content by clicking on tabs. Each tab represents a separate view or functionality within the application.
How do I implement a tab strip in React?
To implement a tab strip in React, you can create a stateful component that manages the active tab. Use conditional rendering to display content based on the selected tab. Libraries like Material-UI or React Tabs can simplify this process.
What are the benefits of using a tab strip in my application?
Using a tab strip enhances user experience by organizing content into manageable sections, reducing clutter, and allowing for quick navigation. It also improves accessibility by providing clear navigation paths.
Can I customize the appearance of a tab strip in React?
Yes, you can customize the appearance of a tab strip in React using CSS or styled-components. Many UI libraries also offer props for styling, allowing for easy adjustments to colors, sizes, and layouts.
What testing strategies should I use for a tab strip component in React?
Testing strategies for a tab strip component should include unit tests to verify functionality, integration tests to ensure it works with other components, and end-to-end tests to validate user interactions. Tools like Jest and React Testing Library are recommended.
Are there any common issues when using a tab strip in React?
Common issues include managing state effectively, ensuring proper accessibility, and handling dynamic content changes. It’s essential to test the component across different browsers and devices to identify any inconsistencies.
The topic of “tab strip” in the context of React, particularly in relation to TestDome, highlights the importance of user interface components that allow for efficient navigation and organization of content. A tab strip is a common UI element that enables users to switch between different views or sections without leaving the current page. In React, implementing a tab strip involves understanding state management, component lifecycle, and event handling, which are essential for creating a seamless user experience.
Key insights from the discussion include the significance of accessibility and responsiveness in tab strip design. It is crucial for developers to ensure that tab strips are not only visually appealing but also functional across various devices and screen sizes. Additionally, incorporating keyboard navigation and screen reader support enhances usability for all users, aligning with best practices in web development.
Furthermore, testing is a vital aspect of developing a tab strip in React. Utilizing tools and frameworks such as Jest and React Testing Library can help ensure that the component behaves as expected under different scenarios. This emphasis on testing reinforces the reliability and robustness of the tab strip, ultimately contributing to a better user experience.
mastering the implementation of a tab strip in React requires a blend of technical skills, attention to detail, and a commitment
Author Profile
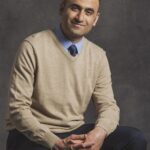
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?