Why Am I Seeing ‘The String Did Not Match The Expected Pattern’ Error?
In the world of programming and data processing, the phrase “the string did not match the expected pattern” can evoke a mix of frustration and curiosity. This common error message serves as a critical reminder of the importance of data integrity and validation in software development. Whether you’re a seasoned coder or a novice just starting your journey, understanding the nuances behind this message can significantly enhance your coding skills and troubleshooting abilities. Join us as we delve into the intricacies of string patterns, explore the reasons behind mismatches, and learn how to effectively handle these situations in your projects.
Overview
At its core, the issue of string mismatches often arises from the need for specific formats in data entry or processing. Developers frequently rely on regular expressions and validation rules to ensure that input data adheres to expected patterns, whether it be for email addresses, phone numbers, or other structured formats. When a string fails to meet these criteria, it triggers an error message that can halt processes and necessitate debugging. Understanding the underlying principles of pattern matching is essential for anyone looking to create robust applications.
Moreover, the implications of a string not matching the expected pattern extend beyond mere error messages. They can impact user experience, data integrity, and overall application performance. By learning to anticipate and
Understanding the Error Message
The error message “the string did not match the expected pattern” typically arises in programming and data processing contexts, particularly when working with regular expressions or data validation mechanisms. This message indicates a failure to conform to a specified format or pattern that the system anticipates.
Common scenarios where this error may occur include:
- Input Validation: When user input does not align with the required format, such as a phone number or email address.
- Data Parsing: In cases where data is extracted from files or databases, and the format of the data does not match what the application expects.
- Regular Expressions: When a string does not satisfy the conditions set by a regex pattern.
To troubleshoot this error effectively, it is essential to understand the expected pattern and the nature of the string being validated.
Common Causes of the Error
Several factors can contribute to this error message:
- Incorrect Pattern Definition: The regular expression may be incorrectly defined or too strict.
- Leading/Trailing Spaces: Extra spaces in the input string that are not accounted for can lead to mismatches.
- Data Type Issues: Attempting to validate a string that contains non-string data types, such as numbers or special characters.
- Locale Differences: Variations in formatting based on locale, such as date formats (MM/DD/YYYY vs. DD/MM/YYYY).
Best Practices for Avoiding This Error
To mitigate the occurrence of this error, follow these best practices:
- Thoroughly Test Regular Expressions: Use tools to validate regex patterns against sample data before implementation.
- Trim Inputs: Always trim leading and trailing whitespace from user inputs.
- Utilize Error Handling: Implement robust error handling to capture and address mismatches gracefully.
- Define Clear Patterns: Ensure that patterns are well-documented and adequately reflect the expected input formats.
Example of Validating Input
When validating a phone number format, for instance, you may define a pattern that looks for digits and specific formatting characters:
“`regex
^\(\d{3}\) \d{3}-\d{4}$
“`
This regex matches a phone number in the format (XXX) XXX-XXXX. Here’s how you can apply it in practice:
Input | Valid? |
---|---|
(123) 456-7890 | Yes |
123-456-7890 | No |
(123)456-7890 | No |
(123) 456 7890 | No |
This table illustrates examples of strings being validated against the defined pattern, indicating which inputs are acceptable and which are not. By adhering to clear patterns and validation rules, developers can significantly reduce the likelihood of encountering the “string did not match the expected pattern” error.
Understanding the Error Message
The error message “the string did not match the expected pattern” typically arises in programming and data validation contexts. It indicates that a given input string does not conform to a predefined format or structure. This message is common in languages such as C, JavaScript, and others when dealing with regular expressions, string parsing, or data input validation.
Common Causes of the Error
Identifying the root causes of this error can help in troubleshooting and resolving the issue effectively. Some frequent reasons include:
- Incorrect Regular Expression: The pattern defined may not accurately reflect the intended structure.
- Invalid Input Data: The data being validated does not meet the criteria established by the regular expression.
- Formatting Issues: Differences in expected formatting, such as extra spaces, missing characters, or incorrect delimiters.
- Locale Settings: Variations in locale might affect the expected format, particularly for dates and numbers.
Regular Expressions and Their Importance
Regular expressions (regex) are powerful tools for string matching and validation. They allow developers to define specific patterns that input strings must match. A basic understanding of regex can prevent many errors.
Regex Component | Description | Example |
---|---|---|
`^` | Asserts position at the start of a string | `^abc` |
`$` | Asserts position at the end of a string | `abc$` |
`\d` | Matches any digit | `\d{3}` |
`\w` | Matches any word character | `\w+` |
`.` | Matches any character except line breaks | `a.b` |
`*` | Matches 0 or more occurrences of the preceding element | `a*` |
`+` | Matches 1 or more occurrences of the preceding element | `a+` |
`?` | Matches 0 or 1 occurrence of the preceding element | `a?` |
Troubleshooting Steps
When encountering the error, follow these troubleshooting steps to identify and fix the issue:
- Review the Input String: Check if the input string adheres to the expected format.
- Validate the Regular Expression: Use online tools to test and validate the regex pattern against sample inputs.
- Check for Escape Characters: Ensure special characters are properly escaped in the regex.
- Examine Data Sources: If the input string comes from an external source, verify that the source data is correct and consistent.
- Debugging: Implement logging to capture the input strings and patterns used, which can aid in identifying discrepancies.
Example Scenarios
To illustrate the error, consider the following scenarios:
- Email Validation: An email input might fail validation due to missing “@” or domain parts. The regex for a basic email might look like:
“`
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
“`
- Date Format: If a date is expected in the format `YYYY-MM-DD` but the input is in `MM/DD/YYYY`, the error will occur. The regex could be:
“`
^\d{4}-\d{2}-\d{2}$
“`
By understanding the underlying causes and employing effective troubleshooting strategies, developers can efficiently resolve instances of the “the string did not match the expected pattern” error.
Understanding String Pattern Mismatches in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘the string did not match the expected pattern’ typically indicates that the input data does not conform to the predefined format. This can often be resolved by validating the input against the expected regular expression or format specification before processing.”
Michael Thompson (Lead Data Scientist, Data Solutions Group). “In data processing, encountering a mismatch in string patterns can lead to significant disruptions. It is crucial to implement robust error handling and logging mechanisms to identify the exact point of failure and rectify the input data accordingly.”
Sarah Lee (Technical Consultant, Code Quality Experts). “When developers face the issue of a string not matching the expected pattern, it is often beneficial to conduct a thorough review of the string manipulation functions used in the code. Ensuring that the functions are properly configured to handle various input scenarios can prevent such errors.”
Frequently Asked Questions (FAQs)
What does the error “the string did not match the expected pattern” mean?
This error typically indicates that the input string does not conform to the predefined format or structure expected by the application or function processing it.
What are common causes of the “the string did not match the expected pattern” error?
Common causes include incorrect formatting, missing required elements, or the use of invalid characters in the string.
How can I troubleshoot this error?
To troubleshoot, verify the expected format, check for typos, ensure all required fields are filled correctly, and validate the string against the expected pattern using regular expressions if applicable.
Are there specific programming languages where this error frequently occurs?
Yes, this error can occur in various programming languages, particularly those that utilize regular expressions or strict data validation, such as C, Java, and JavaScript.
Can I prevent this error from occurring in my application?
Yes, you can prevent this error by implementing robust input validation, providing clear user instructions, and using error handling mechanisms to catch and manage invalid inputs effectively.
What should I do if I encounter this error in a production environment?
In a production environment, log the error details for analysis, notify the relevant development team, and consider implementing a user-friendly error message to guide users in correcting their input.
The phrase “the string did not match the expected pattern” often arises in programming and data processing contexts, indicating a failure to conform to predefined formats or structures. This message typically signals that an input string does not align with the expected syntax, which can lead to errors in data validation, parsing, or processing. Understanding the implications of this error is crucial for developers and data analysts, as it can hinder the functionality of applications and lead to significant debugging efforts.
One of the primary reasons for encountering this error is the use of regular expressions or specific formatting rules that are not met by the input data. For instance, when validating user inputs such as email addresses, phone numbers, or dates, any deviation from the expected format will trigger this error. Consequently, it is essential to implement thorough validation checks and provide clear feedback to users, ensuring that they understand the required format and can correct their inputs accordingly.
Key takeaways from the discussion surrounding this error include the importance of robust input validation mechanisms and the need for comprehensive error handling strategies. Developers should prioritize creating user-friendly interfaces that guide users toward providing the correct data formats. Additionally, implementing logging and debugging tools can facilitate the identification of the source of the error, allowing for quicker resolution and improved user
Author Profile
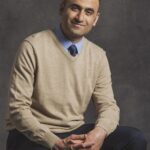
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?