Why Am I Seeing the ‘Tuple Object is Not Callable’ Error in Python?
In the world of Python programming, few errors can be as perplexing as the dreaded “`tuple’ object is not callable” message. This seemingly cryptic notification often leaves developers scratching their heads, wondering how a simple tuple—a fundamental data structure—could lead to such confusion. Whether you’re a seasoned coder or a newcomer to the language, encountering this error can be a frustrating experience that disrupts your workflow. But fear not! Understanding the root causes of this error can not only help you resolve it but also enhance your overall coding skills.
At its core, the “`tuple’ object is not callable” error typically arises from a misunderstanding of how Python handles different data types and their associated methods. When you mistakenly attempt to invoke a tuple as if it were a function, Python throws this error as a safeguard against such misuse. This situation often stems from naming conflicts or incorrect syntax, which can easily trip up even the most vigilant programmers. By exploring the common scenarios that lead to this error, you can develop a clearer understanding of how to avoid it in your own code.
As we delve deeper into the intricacies of this error, we will unravel the common pitfalls that lead to its occurrence and provide practical strategies for troubleshooting and prevention. From clarifying the nature of tuples
Understanding the ‘tuple’ Object is Not Callable Error
The error message `’tuple’ object is not callable` typically occurs in Python when an attempt is made to call a tuple as if it were a function. This error arises due to a misunderstanding of how tuples work and how they differ from callable objects like functions.
When you define a tuple in Python, you create an immutable sequence of elements. If you mistakenly use parentheses in a way that Python interprets your tuple as a function, you will encounter this error. For example, if you have a tuple defined and later try to call it with parentheses, Python raises this error.
Common Causes of the Error
Several scenarios can lead to this error, including:
- Variable Name Conflicts: If a variable that is supposed to reference a function is later overwritten by a tuple, calling that variable will lead to this error.
- Incorrect Parentheses Usage: Using parentheses when trying to access tuple elements can mistakenly imply a function call.
- Returning Tuples from Functions: If a function is intended to return a callable but actually returns a tuple, any attempt to call it will result in this error.
Example Scenarios
To illustrate the error, consider the following examples:
“`python
Scenario 1: Variable name conflict
def my_function():
return “Hello, World!”
my_function = (1, 2, 3) Overwrites the function with a tuple
print(my_function()) Raises: ‘tuple’ object is not callable
Scenario 2: Incorrect parentheses usage
my_tuple = (1, 2, 3)
print(my_tuple(0)) Raises: ‘tuple’ object is not callable
“`
How to Fix the Error
To resolve the `’tuple’ object is not callable` error, consider the following approaches:
- Check Variable Names: Ensure that no variable names clash with function names. Always use distinct names for functions and tuples.
- Use Square Brackets for Indexing: When accessing elements in a tuple, always use square brackets `[]` instead of parentheses `()`.
Best Practices
To avoid encountering this error in your code:
- Use descriptive variable names that clarify their purpose (e.g., `my_tuple` instead of `tuple`).
- Regularly review your code for any variable shadowing or overwriting that could lead to conflicts.
- Utilize type hints and comments to maintain clarity and avoid ambiguity in your code structure.
Error Tracking Table
Scenario | Error Trigger | Resolution |
---|---|---|
Variable Name Conflict | Overwriting a function with a tuple | Rename variable or restore function reference |
Incorrect Parentheses Usage | Using () instead of [] for indexing | Change to square brackets for indexing |
Returning Tuples from Functions | Returning a tuple instead of a callable | Ensure the function returns a callable type |
By implementing these strategies, you can efficiently troubleshoot and prevent the `’tuple’ object is not callable` error in your Python projects.
Understanding the Error Message
The error message `’tuple’ object is not callable` typically occurs in Python when you attempt to call a tuple as if it were a function. This can happen due to various reasons, such as variable name conflicts or misusing parentheses.
Common Causes of the Error
- Variable Shadowing: If you assign a tuple to a variable name that is the same as a built-in function or a previously defined function, you may inadvertently overwrite that reference.
- Improper Use of Parentheses: Using parentheses when you meant to use brackets can lead to treating a tuple like a function.
Example Scenarios
- Variable Shadowing Example
“`python
def my_function():
return “Hello, World!”
my_function = (1, 2, 3) Overwriting the function with a tuple
result = my_function() This will raise the error
“`
- Improper Parentheses Use
“`python
my_tuple = (4, 5, 6)
result = my_tuple() This will raise the error
“`
How to Fix the Error
Resolving this error often involves identifying where the conflict or misuse occurs and making the appropriate changes.
Steps to Resolve
- Rename Variables: Change the name of the tuple to avoid shadowing built-in functions or other callable objects.
“`python
my_tuple = (1, 2, 3) Use a unique name
“`
- Check Function Definitions: Ensure that you haven’t unintentionally assigned a tuple to a variable that was previously a function.
- Use the Correct Syntax: If you need to access elements in a tuple, use square brackets instead of parentheses.
“`python
first_element = my_tuple[0] Correct way to access elements
“`
Diagnostic Tips
- Print Variable Types: Use the `type()` function to identify the type of a variable before calling it.
“`python
print(type(my_function)) Helps determine if it’s a tuple
“`
- Code Review: Review your code for any potential overwrites or misuses of parentheses.
Preventing Future Errors
Adopting best practices can significantly reduce the likelihood of encountering the `’tuple’ object is not callable` error.
Best Practices
- Use Descriptive Variable Names: Choose variable names that clearly describe their purpose. This reduces the chance of accidental shadowing.
- Keep Tuples Immutable: Remember that tuples are immutable. Use them only for fixed collections of items.
- Consistent Coding Style: Maintain a consistent coding style to help identify mistakes quickly.
Good Practice | Description |
---|---|
Descriptive Names | Use clear names to avoid confusion |
Code Reviews | Regularly review code to catch potential errors |
Use of Linters | Employ linting tools to catch common issues early |
By following these guidelines, you can minimize the potential for this error and improve the overall quality of your Python code.
Understanding the ‘tuple’ Object is Not Callable Error in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘tuple’ object is not callable error often arises when a programmer inadvertently attempts to use a tuple as if it were a function. This typically occurs when a variable name is reused, overshadowing a built-in function, leading to confusion in the code’s execution.”
Michael Tran (Python Developer and Educator, Code Academy). “To prevent the ‘tuple’ object is not callable error, it is essential to maintain clear and distinct variable names. By avoiding the reuse of names for both tuples and callable functions, developers can significantly reduce the likelihood of encountering this issue.”
Sarah Johnson (Lead Data Scientist, Data Solutions Corp.). “Debugging the ‘tuple’ object is not callable error requires careful examination of the code. Utilizing tools such as linters and debuggers can help identify where the tuple is mistakenly being treated as a function, allowing for more efficient troubleshooting.”
Frequently Asked Questions (FAQs)
What does the error message ‘tuple’ object is not callable mean?
The error message indicates that you are trying to call a tuple as if it were a function. This typically occurs when parentheses are mistakenly used after a tuple variable.
How can I resolve the ‘tuple’ object is not callable error?
To resolve this error, check your code for instances where you may have used parentheses after a tuple variable. Ensure that you are not attempting to invoke a tuple as a function.
What are common scenarios that lead to this error?
Common scenarios include assigning a tuple to a variable name that shadows a function, or mistakenly using parentheses instead of square brackets when accessing tuple elements.
Can this error occur with other data types?
Yes, this error can occur with any data type if you attempt to call it as a function. However, it is most commonly associated with tuples due to their syntax.
How can I identify the source of the error in my code?
To identify the source, review the traceback provided by Python. Look for the line number where the error occurs and examine the variables involved to determine if a tuple is being incorrectly called.
Is it possible to convert a tuple to a callable function?
No, a tuple cannot be converted to a callable function. However, you can create a function that returns a tuple or use a list or dictionary if you need a mutable or associative data structure.
The error message “‘tuple’ object is not callable” typically arises in Python programming when an attempt is made to call a tuple as if it were a function. This situation often occurs due to naming conflicts, where a variable is inadvertently assigned a tuple, overshadowing a previously defined function with the same name. As a result, when the code attempts to invoke the function, Python raises this error, indicating that it is encountering a tuple instead of a callable function.
To resolve this issue, developers should carefully review their code to identify any naming conflicts. It is advisable to avoid using the same name for both functions and variables to prevent such confusion. Additionally, utilizing clear and descriptive naming conventions can significantly reduce the likelihood of encountering this error. When debugging, checking the type of the variable before calling it can also help identify the source of the problem.
In summary, the “‘tuple’ object is not callable” error serves as a reminder of the importance of maintaining clarity in variable and function naming. By adhering to best practices in coding, such as avoiding name collisions and employing descriptive identifiers, programmers can mitigate the risk of encountering this error. Ultimately, fostering a disciplined approach to coding will enhance code readability and maintainability, leading to more efficient debugging and
Author Profile
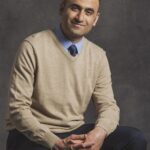
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?