Why Am I Getting a TypeError: ‘Function’ Object is Not Subscriptable?
In the world of programming, particularly when working with Python, encountering errors is an inevitable part of the development journey. One such error that often leaves developers scratching their heads is the infamous `TypeError: ‘function’ object is not subscriptable`. This seemingly cryptic message can halt your progress and prompt a flurry of questions about what went wrong and how to fix it. Whether you’re a seasoned coder or a novice just dipping your toes into the vast ocean of programming, understanding this error is crucial for effective debugging and writing robust code.
As you delve deeper into the intricacies of Python, you’ll find that this error typically arises when you mistakenly attempt to access elements of a function as if it were a list or dictionary. This misunderstanding can stem from a variety of coding practices, such as improper variable assignments or misusing function calls. By unraveling the nuances of this error, you’ll not only enhance your coding skills but also gain insights into the importance of data types and structures in Python.
In this article, we will explore the common scenarios that lead to the `TypeError: ‘function’ object is not subscriptable`, providing you with the tools to diagnose and resolve the issue effectively. We will also discuss best practices to avoid such pitfalls in the future
Understanding the TypeError
A `TypeError` indicating that a ‘function’ object is not subscriptable arises when you attempt to index or slice a function as if it were a list or dictionary. In Python, functions are callable objects and do not support indexing. This error commonly occurs in the following scenarios:
- Attempting to access a specific element of a function like a list.
- Misnaming a variable or function, leading to confusion between a function and a data structure.
- Forgetting to call a function when trying to access its return value.
Consider the following code snippet that triggers this error:
“`python
def my_function():
return [1, 2, 3]
Incorrect usage
value = my_function[0] Raises TypeError
“`
In the above example, `my_function` should be called with parentheses to retrieve its return value. The correct line of code is:
“`python
value = my_function()[0] Correct usage
“`
Common Causes of the Error
Several common mistakes can lead to this specific TypeError:
- Accidental Function Reference: Referring to a function directly instead of its return value.
- Variable Shadowing: Naming a variable the same as a function, causing confusion.
- Improper Data Handling: Assuming a function returns a data structure when it does not.
Here’s a table illustrating common causes and their solutions:
Cause | Example | Solution |
---|---|---|
Accidental Function Reference | my_function[0] | Use my_function()[0] |
Variable Shadowing | def my_function(): …; my_function = 5 | Rename the variable or function |
Improper Data Handling | result = my_function; value = result[0] | Ensure result is called: result = my_function(); |
Debugging Steps
To effectively debug this error, follow these steps:
- Check the Function Call: Ensure that you are calling the function with parentheses when attempting to access its return value.
- Review Variable Names: Look for any variable names that may be overshadowing your function.
- Print Debugging: Use print statements to display the types of your variables to confirm they are what you expect.
- Utilize Type Checking: Implement type checking with `isinstance()` to verify the type of the object before attempting to index it.
By adhering to these practices, you can prevent the ‘function’ object is not subscriptable error and ensure your code runs smoothly.
Understanding the Error
The error `TypeError: ‘function’ object is not subscriptable` occurs in Python when you attempt to index or slice a function object as if it were a list, dictionary, or other subscriptable types. This typically happens when you mistakenly reference a function instead of the data structure you intended to access.
Common Causes
- Mistakenly using parentheses: When you call a function but forget to include the parentheses, Python treats the function itself as an object.
- Variable shadowing: A variable name can overshadow a built-in function or a previously defined function, leading to confusion.
- Incorrect return type: A function might return another function instead of a data structure.
Examples of the Error
Example 1: Function Call Mistake
“`python
def my_function():
return [1, 2, 3]
Incorrect usage
result = my_function[0] Raises TypeError
“`
In this example, `my_function` is called without parentheses, leading to an attempt to index the function object itself.
Example 2: Variable Shadowing
“`python
def list_func():
return [1, 2, 3]
list_func = 5 Overwrites the function with an integer
print(list_func[0]) Raises TypeError
“`
The variable `list_func` now holds an integer, which is not subscriptable.
How to Fix the Error
To resolve the `TypeError: ‘function’ object is not subscriptable`, follow these strategies:
- Ensure Correct Function Call: Always verify that functions are called correctly with parentheses.
“`python
result = my_function() Correct way to call
print(result[0]) Accessing the first element
“`
- Avoid Variable Name Conflicts: Use unique names for variables and functions to prevent shadowing.
“`python
my_list = [1, 2, 3]
print(my_list[0]) Use a different name for the variable
“`
- Check Function Returns: If a function is expected to return a list or dictionary, ensure it does so.
“`python
def get_list():
return [1, 2, 3]
my_list = get_list() Correctly call the function
print(my_list[0]) Access the first element
“`
Debugging Tips
When encountering this error, consider the following debugging tips:
Tip | Description |
---|---|
Use print statements | Print the types of variables to see if they match expectations. |
Check function definitions | Ensure functions are returning the intended types. |
Utilize IDE tools | Many IDEs provide error highlighting and suggestions. |
By following these guidelines, you can effectively troubleshoot and resolve the `TypeError: ‘function’ object is not subscriptable` in your Python code.
Understanding the TypeError: ‘function’ Object is Not Subscriptable
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The TypeError ‘function’ object is not subscriptable typically arises when a programmer attempts to index or slice a function as if it were a list or dictionary. This mistake often occurs due to a naming conflict between a function and a variable, leading to confusion in the code.”
Michael Chen (Python Developer and Educator, CodeMaster Academy). “To resolve the TypeError ‘function’ object is not subscriptable, developers should carefully review their code for any instances where a function name is mistakenly used in place of a data structure. Ensuring that variable names are distinct from function names is crucial for preventing such errors.”
Sarah Thompson (Lead Data Scientist, Analytics Solutions Group). “In data analysis and manipulation tasks, encountering a TypeError ‘function’ object is not subscriptable can be particularly frustrating. It is essential to verify that the object being accessed is indeed a list, tuple, or dictionary, rather than a callable function. Utilizing debugging tools can aid in identifying the source of the error.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: ‘function’ object is not subscriptable” mean?
This error indicates that you are trying to use indexing or slicing on a function object, which is not allowed in Python. Functions cannot be accessed like lists or dictionaries.
What are common causes of this TypeError?
Common causes include mistakenly trying to index a function instead of a list or dictionary, or using parentheses instead of brackets when attempting to access elements of a data structure.
How can I resolve the “function object is not subscriptable” error?
To resolve this error, check your code for instances where you are trying to index a function. Ensure that you are calling the function correctly and accessing the appropriate data structure that it returns.
Can this error occur with lambda functions?
Yes, this error can occur with lambda functions if you mistakenly attempt to index the lambda itself instead of the data it returns. Always ensure you are working with the correct data type.
Is there a way to debug this error effectively?
To debug this error, use print statements or a debugger to trace the variable types in your code. Confirm that you are working with the intended objects and not inadvertently referencing a function.
Are there any specific scenarios where this error frequently occurs?
This error frequently occurs in scenarios involving callbacks, higher-order functions, or when misusing built-in functions. Pay close attention to variable assignments and function calls to avoid this mistake.
The TypeError ‘function’ object is not subscriptable typically occurs in Python when an attempt is made to index or slice a function object as if it were a list, tuple, or dictionary. This error highlights a fundamental misunderstanding of the data types being used in the code. Functions in Python are callable objects and cannot be accessed using indexing syntax, which is reserved for subscriptable types like lists or dictionaries. Understanding the distinction between these types is crucial for effective programming in Python.
To resolve this error, developers should carefully review their code to ensure that they are not mistakenly trying to index a function. Common scenarios that lead to this error include mistakenly using parentheses when defining a function or inadvertently naming a variable the same as a function. By ensuring that variable names are distinct and that functions are called correctly, programmers can avoid this common pitfall.
Furthermore, this error serves as a reminder of the importance of clear and consistent naming conventions in programming. By adopting meaningful names for functions and variables, developers can reduce the likelihood of confusion and enhance the readability of their code. Overall, understanding the nature of Python objects and adhering to best practices in naming and code structure can significantly mitigate the occurrence of TypeError ‘function’ object is not subscript
Author Profile
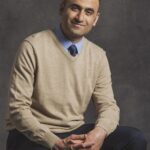
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?