Is Your Code at Risk? Understanding the Dangers of Unsafe Assignment of Error-Typed Values
In the ever-evolving landscape of programming, developers often face a myriad of challenges that can lead to unexpected behaviors in their code. One such challenge is the “unsafe assignment of an error typed value,” a concept that can perplex even seasoned programmers. This phenomenon not only raises questions about code reliability and safety but also highlights the importance of understanding type systems and error handling in modern programming languages. As software becomes increasingly complex, grasping the implications of such assignments is crucial for maintaining robust and error-free applications.
The notion of unsafe assignment revolves around the potential pitfalls that arise when error types are improperly handled or assigned within a program. This can lead to scenarios where errors are masked, mismanaged, or worse, cause runtime failures that could have been avoided with proper type checks and error handling strategies. Understanding the nuances of error types and their assignments is essential for developers who aim to write clean, maintainable, and secure code.
As we delve deeper into this topic, we will explore the common scenarios that lead to unsafe assignments, the implications of such practices, and best practices for managing error types effectively. By equipping ourselves with this knowledge, we can enhance our coding practices and contribute to the creation of more reliable software solutions. Join us as we unravel the intricacies of error
Understanding Unsafe Assignments
Unsafe assignment of an error typed value typically arises in programming contexts where variables may inadvertently hold error states, leading to unexpected behavior in applications. This phenomenon is particularly pertinent in languages with strict typing systems, such as TypeScript or C.
When an error type is assigned to a variable that is expected to hold a different type, it can lead to runtime errors, data inconsistencies, or application crashes. The core issue lies in the assumption that a variable can safely contain multiple types without proper checks or safeguards in place.
Types of Error Assignments
There are several common scenarios where unsafe assignments can occur:
- Implicit Type Casting: When a programming language allows automatic conversion between types, it can lead to errors being assigned to non-error types.
- Error Handling Mechanisms: Inadequate handling of exceptions can result in errors being captured and assigned incorrectly.
- Data Structures: When using collections or maps, assigning an error object where a value of a different type is expected can lead to unsafe conditions.
Best Practices for Safe Assignments
To mitigate the risks associated with unsafe assignments, consider the following best practices:
- Explicit Type Checks: Always check the type of a value before assigning it to a variable. This ensures that only the expected types are used.
- Use of Type Guards: In languages like TypeScript, utilizing type guards can help narrow down types and prevent unsafe assignments.
- Error Handling Standards: Implement standardized error handling mechanisms to ensure that errors are processed correctly and do not propagate unintentionally.
Example of Unsafe Assignment
The following code snippet illustrates an unsafe assignment scenario in TypeScript:
“`typescript
function processValue(value: number | Error) {
let result: number;
// Unsafe assignment: no type check before assignment
result = value; // This can be an Error
}
“`
In this example, if `value` is an instance of `Error`, the assignment to `result` will lead to a type error at runtime.
Table of Safeguards Against Unsafe Assignments
Safeguard | Description |
---|---|
Type Assertions | Forcefully asserting the type of a variable to avoid runtime errors. |
Try-Catch Blocks | Catching exceptions to prevent error propagation and mishandling. |
Strict Type Definitions | Defining types explicitly to prevent unintended assignments. |
Code Reviews | Peer reviews of code to catch unsafe assignments during development. |
By implementing these safeguards, developers can enhance the safety and reliability of their code, reducing the likelihood of encountering unsafe assignments of error-typed values.
Understanding Unsafe Assignment of Error Typed Values
The term “unsafe assignment of an error typed value” typically arises in programming languages with strict typing systems, particularly in languages like TypeScript and Rust. This scenario often occurs when a developer attempts to assign a value of an error type to a variable that expects a different type. Such practices can lead to runtime errors and behaviors, compromising the integrity of the application.
Common Causes
Several factors can contribute to this issue:
- Type Mismatch: Attempting to assign a variable that is expected to hold a specific type with an error object or any incompatible type.
- Improper Error Handling: Failing to handle errors appropriately can lead to untyped values being passed around in the code.
- Incorrect Function Return Types: Functions that return error types need to be clearly defined; misalignment can result in unsafe assignments.
- Lack of Type Assertion: In languages that support type assertions, failing to assert the correct type can lead to assignment issues.
Examples of Unsafe Assignments
Here are some common programming scenarios demonstrating unsafe assignments:
“`typescript
let errorValue: Error;
let result: string;
// Unsafe assignment
result = errorValue; // Type ‘Error’ is not assignable to type ‘string’.
“`
“`rust
fn process(value: Result
let error: String;
// Unsafe assignment
error = value; // Mismatched types: Result
}
“`
Best Practices for Safe Assignments
To mitigate the risks of unsafe assignments, consider the following practices:
- Use Type Guards: Implement type guards to ensure that the value being assigned matches the expected type.
“`typescript
if (isError(value)) {
// Handle error
} else {
// Safe assignment
result = value;
}
“`
- Explicit Type Annotations: Clearly define types in functions and variables to avoid implicit any types.
- Error Handling Mechanisms: Utilize try-catch blocks or equivalent constructs to manage errors effectively.
- Compiler Warnings: Pay attention to compiler warnings or errors regarding type assignments to catch potential issues early.
Tools for Identifying Unsafe Assignments
Utilize various tools and practices to identify and resolve unsafe assignments:
Tool | Description |
---|---|
TypeScript Compiler | Provides strict type-checking and error identification. |
Rust Compiler | Enforces strict type rules and provides error messages. |
Linters | Tools like ESLint can identify unsafe coding practices. |
IDE Features | Many IDEs offer real-time type-checking and suggestions. |
Recognizing and addressing unsafe assignments of error typed values is crucial for maintaining robust and reliable code. Employing best practices and utilizing available tools can significantly minimize the risks associated with type mismatches. By adhering to these guidelines, developers can enhance the safety and reliability of their applications.
Understanding the Risks of Unsafe Assignment in Error Handling
Dr. Emily Tran (Software Security Analyst, CyberSafe Institute). “The unsafe assignment of an error typed value can lead to significant vulnerabilities in software applications. When error values are improperly handled, they may propagate through the system, potentially exposing sensitive data or causing unexpected behavior that can be exploited by malicious actors.”
Michael Chen (Lead Developer, TechGuard Solutions). “In programming, assigning error values without proper validation can introduce critical bugs. It is essential to implement strict type checking and error handling mechanisms to ensure that only valid data is processed, thus maintaining the integrity of the application and preventing runtime errors.”
Laura Patel (Senior Software Engineer, CodeSecure Labs). “Developers must be vigilant about the implications of unsafe assignments in error handling. By adopting best practices such as using explicit error types and employing robust logging strategies, teams can mitigate the risks associated with incorrect error assignments and enhance overall software reliability.”
Frequently Asked Questions (FAQs)
What does “unsafe assignment of an error typed value” mean?
This phrase typically refers to a programming scenario where a value of an error type is assigned to a variable that does not expect or handle error types, potentially leading to runtime errors or unexpected behavior.
In which programming languages is this issue commonly encountered?
This issue is commonly encountered in languages with strict type systems, such as TypeScript, Swift, and Rust, where type safety is enforced to prevent errors at compile time.
How can developers avoid unsafe assignments of error typed values?
Developers can avoid unsafe assignments by implementing proper type checks, using union types, or employing error handling mechanisms such as try-catch blocks to ensure that only valid types are assigned to variables.
What are the potential consequences of unsafe assignments?
The potential consequences include runtime errors, application crashes, data corruption, and security vulnerabilities, which can severely impact the reliability and stability of the software.
Are there tools or practices to detect unsafe assignments in code?
Yes, many integrated development environments (IDEs) and static analysis tools can detect unsafe assignments. Practices such as code reviews, type annotations, and unit testing also help identify potential issues early in the development process.
What should a developer do if they encounter an unsafe assignment warning?
A developer should investigate the warning, review the code to understand the context, and refactor the code to ensure type safety, possibly by changing variable types or implementing error handling strategies.
The concept of “unsafe assignment of an error typed value” primarily pertains to programming practices where an error type is improperly assigned or handled within code. This situation often arises in languages that utilize strict type systems, where assigning an error type to a variable that expects a different type can lead to unpredictable behavior, runtime errors, or compromised application stability. Such practices can undermine the robustness of software, making it crucial for developers to adhere to type safety principles to ensure that error handling is both effective and predictable.
One of the key takeaways from the discussion surrounding this topic is the importance of type safety in programming. By ensuring that values assigned to variables are of the expected type, developers can prevent a range of issues that might arise from type mismatches. This includes not only runtime errors but also potential security vulnerabilities that could be exploited by malicious actors. Therefore, adopting strict type-checking practices and utilizing comprehensive error handling mechanisms is essential for maintaining code integrity.
Moreover, the implications of unsafe assignments extend beyond immediate errors; they can also affect long-term maintainability and readability of code. When error types are mishandled, it can lead to confusion among developers who may struggle to understand the flow of data and error management within the application. As a result
Author Profile
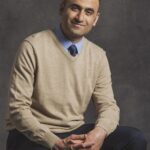
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?