How Can Unsatisfied Dependencies Be Expressed Through Fields in Your Data Model?
In the intricate world of software development, dependencies are the lifeblood of efficient coding practices. However, navigating these dependencies can sometimes lead to frustrating roadblocks, particularly when developers encounter the dreaded “unsatisfied dependency expressed through field” error. This message often signals a deeper issue within the codebase, hinting at misconfigurations or missing components that can derail even the most well-planned projects. Understanding this error is crucial for developers who wish to maintain smooth workflows and ensure their applications run seamlessly.
When a developer faces an unsatisfied dependency, it typically indicates that a required component or service is not available or properly configured within the application context. This can arise from various factors, such as incorrect annotations, mismanaged configurations, or simply forgetting to include a necessary library. The implications of this error are significant, as they can lead to application failures or unexpected behavior, making it essential for developers to grasp the underlying principles of dependency management.
Moreover, addressing unsatisfied dependencies goes beyond mere troubleshooting; it involves a proactive approach to software architecture and design. By understanding how dependencies are expressed and managed within a system, developers can create more resilient applications that are easier to maintain and scale. In the following sections, we will delve deeper into the causes, implications, and best
Understanding Unsatisfied Dependencies
Unsatisfied dependency issues arise when a component or service cannot locate one or more required dependencies to function correctly. In software development, especially within dependency injection frameworks, this can manifest as an error stating that a dependency was not satisfied through its declared field. This typically occurs due to misconfigurations, missing dependencies, or incorrect annotations.
Common Causes of Unsatisfied Dependencies
Several factors contribute to unsatisfied dependencies. Recognizing these can aid in troubleshooting:
- Misconfiguration: Incorrect setup in the configuration files or classes can lead to dependencies not being found.
- Component Scanning Issues: If a component is not picked up during the scanning process, it may not be available for injection.
- Scope Mismatches: When the scope of a dependency does not match the required scope in the class needing the dependency, it can lead to unsatisfied dependencies.
- Missing Dependencies: If a required library or service is not present, the application cannot resolve the dependency.
- Circular Dependencies: When two or more components depend on each other, it can cause a deadlock in dependency resolution.
Resolving Unsatisfied Dependencies
To address unsatisfied dependency issues, developers can follow several best practices:
- Review Configuration: Ensure all components are correctly defined and configured.
- Check Component Scanning: Verify that the necessary packages are included in the component scanning configuration.
- Validate Dependency Availability: Confirm that all required dependencies are correctly included in the project and available in the classpath.
- Analyze Scope Definitions: Make sure that the scope of the dependency matches the expected scope in the dependent component.
- Refactor Circular Dependencies: Modify the design to eliminate circular dependencies, possibly by using setter injection or introducing an intermediary component.
Example of Dependency Injection Configuration
The following table illustrates a basic dependency injection configuration that might lead to unsatisfied dependencies if not set up correctly.
Component | Dependency | Configuration Method | Potential Issue |
---|---|---|---|
ServiceA | ServiceB | Constructor Injection | ServiceB not found |
ServiceC | ServiceA | Setter Injection | Circular dependency |
ServiceD | ServiceE | Field Injection | ServiceE misconfigured |
By ensuring each of these components is properly defined and the dependencies are correctly injected, developers can minimize the occurrence of unsatisfied dependency errors.
Understanding Unsatisfied Dependency Expressed Through Field
Unsatisfied dependency expressed through field is a term often encountered in software development, particularly in the context of dependency injection frameworks. This term typically indicates that a component or service within an application requires a specific dependency to function correctly, but this dependency is either not provided or cannot be resolved.
Common Causes of Unsatisfied Dependencies
Several factors can lead to unsatisfied dependencies in an application:
- Misconfiguration: Incorrect configuration settings can prevent the dependency injection framework from recognizing or supplying the required dependency.
- Missing Beans or Services: In frameworks like Spring, if a required bean is not defined in the application context, it leads to unsatisfied dependencies.
- Incorrect Scoping: When the scope of a dependency does not match the required scope of the dependent component, it may cause resolution failures.
- Circular Dependencies: If two or more components depend on each other, it can create a scenario where neither can be instantiated, resulting in unsatisfied dependencies.
Identifying Unsatisfied Dependencies
To identify unsatisfied dependencies in your application, consider the following approaches:
- Error Logs: Review application logs for error messages indicating unsatisfied dependencies. Frameworks typically provide detailed error messages that specify which dependencies could not be resolved.
- Dependency Graphs: Utilize tools that generate dependency graphs to visualize how components depend on each other, making it easier to spot issues.
- Unit Testing: Implement unit tests that check for the presence of required dependencies, allowing you to catch unsatisfied dependencies early in the development process.
Resolving Unsatisfied Dependencies
To resolve unsatisfied dependencies, you can take the following steps:
- Check Configuration: Verify that your configuration files (e.g., XML, annotations) correctly define all required beans or services.
- Define Missing Beans: If a required bean is missing, define it in the appropriate configuration file or class.
- Adjust Scopes: Ensure that the scopes of your components are compatible. For instance, a singleton bean should not depend on a prototype bean directly.
- Refactor Code: In cases of circular dependencies, consider refactoring the code to eliminate the circular references or introduce an intermediary service.
Example Scenario
Consider a Spring application where a service `UserService` depends on a repository `UserRepository`. If `UserRepository` is not defined, the following error might be thrown:
“`
UnsatisfiedDependencyException: Error creating bean with name ‘userService’: Unsatisfied dependency expressed through field ‘userRepository’;
“`
To resolve this:
- Ensure `UserRepository` is defined as a Spring bean.
- Confirm that there are no scope mismatches between `UserService` and `UserRepository`.
Issue | Resolution |
---|---|
Misconfigured Bean | Correct the configuration settings. |
Missing Bean Definition | Define the missing bean. |
Scope Mismatch | Adjust bean scopes accordingly. |
Circular Dependency | Refactor to break the cycle. |
Best Practices
To minimize the occurrence of unsatisfied dependencies, consider the following best practices:
- Use Dependency Injection: Utilize dependency injection frameworks properly to manage dependencies effectively.
- Maintain Clear Separation of Concerns: Ensure that components have clear responsibilities to reduce interdependencies.
- Regularly Review Dependency Configuration: Periodically check your dependency configurations for accuracy and completeness.
By implementing these strategies, developers can effectively manage dependencies, reducing the likelihood of encountering unsatisfied dependency issues in their applications.
Understanding Unsatisfied Dependency in Software Development
Dr. Emily Chen (Software Architect, Tech Innovations Inc.). “Unsatisfied dependency expressed through field often indicates a misalignment between the expected and actual state of a software component. It is crucial for developers to implement robust dependency management practices to mitigate these issues.”
Mark Thompson (Lead Systems Analyst, FutureTech Solutions). “When encountering an unsatisfied dependency expressed through field, it is essential to perform a thorough analysis of the data models involved. This can reveal underlying issues that may not be immediately apparent and help in formulating effective solutions.”
Linda Garcia (DevOps Engineer, CloudSync Technologies). “In many cases, unsatisfied dependency expressed through field can lead to significant bottlenecks in the deployment pipeline. Addressing these dependencies early in the development cycle can streamline processes and enhance overall software quality.”
Frequently Asked Questions (FAQs)
What does “unsatisfied dependency expressed through field” mean?
This phrase typically refers to a situation in software development where a required component or service is not available or properly configured, resulting in a failure to fulfill a dependency needed for a specific field or operation.
How can I identify unsatisfied dependencies in my application?
You can identify unsatisfied dependencies by examining error logs, utilizing dependency management tools, or performing static code analysis to detect missing or improperly configured components.
What are common causes of unsatisfied dependencies?
Common causes include misconfigured application settings, missing libraries or modules, version mismatches between dependencies, and changes in the environment that affect component availability.
How do I resolve unsatisfied dependencies?
To resolve unsatisfied dependencies, ensure that all required components are correctly installed and configured, update any outdated libraries, and verify that the application environment matches the expected configuration.
Can unsatisfied dependencies impact application performance?
Yes, unsatisfied dependencies can severely impact application performance, leading to errors, crashes, or degraded functionality, which can ultimately affect user experience and system reliability.
Are there tools available to help manage dependencies?
Yes, there are several tools available, such as Maven, Gradle, and npm, which can help manage dependencies, automate updates, and ensure that all required components are correctly integrated into your application.
The term “unsatisfied dependency expressed through field” typically refers to a situation in software development, particularly in dependency management systems. It highlights instances where a required component or library is not available or cannot be resolved within the specified context of an application. This situation can lead to significant issues in the build process, runtime errors, or even application failures, thus impacting overall project stability and performance.
Understanding the implications of unsatisfied dependencies is crucial for developers and project managers. It emphasizes the need for thorough dependency management practices, including regular updates, proper versioning, and comprehensive testing. By addressing these dependencies proactively, teams can mitigate risks associated with application failures and ensure smoother integration of various components within their software projects.
Furthermore, the identification and resolution of unsatisfied dependencies can lead to improved collaboration among team members. By fostering a culture of clear communication regarding dependencies, teams can work more effectively together, ensuring that all necessary components are accounted for and that potential conflicts are addressed early in the development process. This proactive approach not only enhances the quality of the final product but also streamlines the development lifecycle.
Author Profile
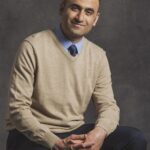
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?