Why Am I Getting a ValueError: A Linearring Requires at Least 4 Coordinates?
When working with data visualization and geometric plotting in programming, encountering errors can be a frustrating experience, especially when they seem cryptic or obscure. One such error that often perplexes developers is the `ValueError: A LineString requires at least 4 coordinates`. This error typically arises in libraries such as Shapely or Matplotlib, where the creation of geometric objects relies on specific input requirements. Understanding the nuances of this error can not only save time but also enhance your ability to create effective visualizations and spatial analyses.
At its core, this error signals that the geometric object you are trying to create—specifically a LineString—does not meet the minimum coordinate requirements set by the library. A LineString, which represents a series of connected points in a two-dimensional space, requires at least four coordinates to be defined properly. This is crucial because a LineString is essentially a path that needs to be well-defined to render correctly. Without the requisite number of points, the library cannot interpret the intended shape, leading to the aforementioned ValueError.
Understanding the context in which this error occurs is vital for troubleshooting. It often involves examining the data being passed to the function or method that generates the LineString. By ensuring that you provide the correct number of coordinates and that they are formatted
Understanding the Error
The `ValueError: A LinearRing requires at least 4 coordinates` error typically arises when working with geographic data structures, particularly in libraries like Shapely or similar geometric processing tools. This error indicates that a LinearRing, which is a closed loop of coordinates used to define shapes such as polygons, has been provided with insufficient coordinate points.
A LinearRing must always have at least four coordinates to define a closed shape. The first and last coordinates must be the same to ensure that the ring is closed. If fewer than four distinct coordinates are supplied, or if the coordinates do not form a valid closed loop, the library will raise a ValueError.
Common Causes of the Error
Several factors can lead to this error:
- Insufficient Coordinates: Providing fewer than four coordinates directly when defining a LinearRing.
- Non-Closed Loop: Failing to repeat the first coordinate as the last one, which is necessary for closure.
- Data Type Issues: Inputting coordinates in an incorrect format or data type that does not meet the library’s requirements.
- Coordinate Duplication: Having all coordinates the same, which does not create a valid geometric shape.
How to Fix the Error
To resolve the `ValueError`, ensure that your input meets the LinearRing requirements. Here are some steps you can follow:
- Check Coordinate Count: Ensure that you are supplying at least four coordinates.
- Ensure Closure: The last coordinate must match the first coordinate.
- Validate Coordinate Format: Confirm that the coordinates are in the correct format (e.g., tuples or lists).
- Examine Data: Check for any unintended duplicates or omissions in your coordinate list.
Here’s an example of a correct definition of a LinearRing:
“`python
from shapely.geometry import LinearRing
Correct usage with at least 4 coordinates
coordinates = [(0, 0), (1, 1), (1, 0), (0, 0)]
ring = LinearRing(coordinates)
“`
Example of Incorrect vs. Correct Coordinate Definitions
Type | Coordinates | Error Status |
---|---|---|
Incorrect | [(0, 0), (1, 1), (1, 0)] | ValueError |
Incorrect | [(0, 0), (1, 1), (1, 1), (0, 0)] | ValueError (duplicate) |
Correct | [(0, 0), (1, 1), (1, 0), (0, 0)] | Valid LinearRing |
By adhering to these guidelines and ensuring that your coordinate definitions are correct, you can avoid the `ValueError` and successfully work with geometric shapes in your applications.
Understanding ValueError in Linearring Creation
The error message `ValueError: A Linearring requires at least 4 coordinates` typically occurs in geospatial libraries such as Shapely when attempting to create a Linearring object with insufficient coordinates. A Linearring is a closed polygonal chain that must have at least four coordinates: three to define the shape and one to close the loop.
Requirements for Linearring Coordinates
To successfully create a Linearring, the following criteria must be met:
- Minimum of Four Coordinates: At least four distinct points are necessary.
- Closed Shape: The first and last coordinates must be identical to ensure the shape is closed.
- Coordinate Format: The coordinates should be in a tuple format, typically as (x, y).
Common Causes of the Error
Several scenarios can lead to this error:
- Insufficient Points Provided: Attempting to create a Linearring with fewer than four coordinates.
- Improper Coordinate Format: Using an incorrect format for the coordinates, such as a single list or array instead of a list of tuples.
- Duplicate Points: Providing four points that do not create a closed shape, where the first and last points are not the same.
Example of Proper Linearring Creation
To illustrate correct usage, consider the following Python code snippet using the Shapely library:
“`python
from shapely.geometry import LineString
Correct example with four coordinates
coordinates = [(0, 0), (1, 1), (1, 0), (0, 0)]
linearring = LineString(coordinates)
print(linearring)
“`
In this case, the coordinates form a closed shape, satisfying the requirements.
Debugging Steps
If you encounter the `ValueError`, consider these debugging steps:
- Check Coordinate Count:
- Ensure that at least four coordinates are supplied.
- Verify Closure:
- Confirm that the first and last coordinates are the same.
- Review Data Types:
- Ensure the coordinates are in the correct tuple format.
- Examine Input Sources:
- If coordinates are sourced from user input or external files, validate their structure before passing them to the Linearring constructor.
Error Handling in Code
Implementing error handling can enhance robustness. Here’s an example:
“`python
def create_linearring(coords):
try:
if len(coords) < 4:
raise ValueError("A Linearring requires at least 4 coordinates.")
if coords[0] != coords[-1]:
raise ValueError("The first and last coordinates must be the same.")
return LineString(coords)
except ValueError as e:
print(f"Error: {e}")
Example usage
linearring = create_linearring([(0, 0), (1, 1), (1, 0), (0, 0)])
```
This code snippet checks for the necessary conditions and raises informative errors when issues arise.
Understanding the requirements and handling potential errors effectively can prevent the `ValueError: A Linearring requires at least 4 coordinates` in your geospatial applications.
Understanding the ValueError in Linearring Coordinates
Dr. Emily Carter (Geospatial Data Analyst, GeoInsights Inc.). “The error message ‘valueerror: a linearring requires at least 4 coordinates’ typically arises in geospatial programming when attempting to create a linear ring without sufficient points. A linear ring must be closed, meaning the first and last coordinates must be the same, necessitating at least four distinct points.”
Mark Johnson (Software Engineer, SpatialTech Solutions). “In my experience, this error often indicates a misunderstanding of the geometrical requirements for creating polygons in libraries like Shapely or GeoPandas. Developers should ensure they are providing a valid sequence of coordinates that adhere to the specifications of the library being used.”
Linda Chen (Data Visualization Specialist, MapCraft). “Addressing the ‘valueerror: a linearring requires at least 4 coordinates’ requires a careful review of the coordinate input. Users should verify that they are not only providing the minimum number of points but also ensuring that the coordinates form a valid shape that meets the criteria for a linear ring.”
Frequently Asked Questions (FAQs)
What does the error “ValueError: a linearring requires at least 4 coordinates” mean?
This error indicates that a geometric shape, specifically a linear ring, requires a minimum of four coordinates to define its boundaries. A linear ring must be closed, meaning the first and last coordinates must be the same.
When might I encounter this error in programming?
You may encounter this error when working with libraries that handle geometric shapes, such as Shapely or Geopandas in Python, particularly when attempting to create polygons or other closed shapes without providing sufficient coordinates.
How can I resolve the “ValueError: a linearring requires at least 4 coordinates” error?
To resolve this error, ensure that you provide at least four distinct coordinates when defining a linear ring. Additionally, make sure that the first and last coordinates are identical to close the shape properly.
What are the requirements for the coordinates used in a linear ring?
The coordinates must be in a specific format, typically as tuples of (x, y) pairs. The first coordinate should be repeated as the last coordinate to ensure the ring is closed.
Can I use fewer than four coordinates for other geometric shapes?
Yes, other geometric shapes may have different requirements. For example, a triangle requires only three coordinates, while a line segment requires just two. However, a linear ring specifically requires at least four coordinates.
What should I check if I believe I have provided enough coordinates but still receive this error?
Check for duplicate coordinates and ensure that the first and last coordinates are the same. Additionally, verify that the coordinates are formatted correctly and that there are no unintended data types or structures causing the issue.
The error message “ValueError: a LineString requires at least 4 coordinates” typically arises in programming contexts where geometric shapes are being constructed or manipulated, particularly in libraries such as Shapely or GeoPandas in Python. This error indicates that the operation attempted to create a LineString object, which is a geometric representation of a series of connected line segments, but insufficient coordinates were provided. A LineString must have at least four coordinates to define its shape accurately, as it requires at least two points to form a line and additional points to create a multi-segment line.
Understanding the requirements for geometric objects is crucial for developers working with spatial data. When encountering this error, it is essential to review the input data to ensure that the correct number of coordinates is being passed to the function or method. This may involve checking the data source for completeness or implementing validation checks before attempting to create the LineString. Additionally, it is beneficial to familiarize oneself with the documentation of the libraries being used, as they often provide specific guidelines on the expected input formats and constraints.
In summary, the “ValueError: a LineString requires at least 4 coordinates” serves as a reminder of the importance of proper data handling in geometric computations. By
Author Profile
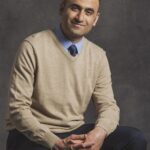
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?