Why Am I Getting a ‘TypeError: Cannot Read Properties of _history’ in Vue Tests?
In the ever-evolving landscape of web development, Vue.js has emerged as a powerful framework, enabling developers to create dynamic and interactive user interfaces with ease. However, as with any technology, the journey is not without its bumps. One common issue that developers encounter is the dreaded `TypeError: cannot read properties of ‘_history’?`, which can arise during testing. This error can be particularly frustrating, especially when it disrupts the flow of development and testing processes. Understanding the root causes and solutions to this problem is essential for any Vue developer looking to maintain a smooth workflow and ensure their applications run seamlessly.
This article delves into the intricacies of this TypeError, exploring its common triggers and implications within the context of Vue.js applications. We will examine the scenarios in which this error typically surfaces, particularly during unit testing or when integrating Vue Router. By gaining insight into the underlying mechanics of Vue’s reactivity system and the router’s history management, developers can better navigate these challenges and enhance their debugging skills.
Furthermore, we will highlight best practices and preventive measures to help you avoid encountering this error in the first place. Whether you are a seasoned Vue developer or just starting your journey, understanding how to tackle this TypeError will empower you to write more robust tests and
Understanding the Error
The error message `TypeError: Cannot read properties of ‘_history’` typically indicates that the code is attempting to access a property of an object that is either or null. In the context of Vue applications, this commonly arises when dealing with the Vue Router or state management libraries like Vuex.
When this error occurs, it can disrupt the application’s flow and lead to unexpected behavior. The `_history` property is often part of the internal implementation of Vue Router, which manages the navigation state of the application. Understanding the root cause of this error requires investigating several potential issues.
Common Causes
There are several common causes for this error in a Vue application:
- Incorrect Router Configuration: If the Vue Router is not properly initialized or if the routes are misconfigured, the application may fail to recognize the `_history` property.
- Lifecycle Issues: Accessing the router instance before it is fully initialized can lead to this error. This often occurs when trying to access router properties in the wrong lifecycle hook.
- State Management Problems: In applications using Vuex, an incorrect state management setup could lead to properties being accessed, resulting in this error.
- Version Mismatches: Using incompatible versions of Vue, Vue Router, or other dependencies can lead to unexpected behavior and errors.
Troubleshooting Steps
To resolve the `TypeError: Cannot read properties of ‘_history’`, consider the following troubleshooting steps:
- Check Router Initialization: Ensure that the Vue Router is correctly initialized in your application. Review your main entry file (usually `main.js` or `app.js`) and confirm that the router is passed as an option to the Vue instance.
- Validate Route Definitions: Review your route definitions for typos or structural issues. Ensure that all routes are properly defined and that their components are imported correctly.
- Examine Lifecycle Hooks: If you’re accessing router properties in your components, ensure you’re doing so in the appropriate lifecycle hook, such as `mounted` or `created`, after the router has been fully initialized.
- Inspect Dependencies: Ensure that your Vue, Vue Router, and other related dependencies are compatible. Check the version numbers in your `package.json` and update them if necessary.
- Debugging: Use console logs to debug the state of the router before the error occurs. This can help identify whether the router instance is defined at the point of access.
Example Code Snippet
Here’s an example of how to correctly initialize the Vue Router in your application:
“`javascript
import Vue from ‘vue’;
import App from ‘./App.vue’;
import router from ‘./router’;
new Vue({
router, // Ensure the router is passed here
render: h => h(App),
}).$mount(‘app’);
“`
If you attempt to access the router in a component, do so as follows:
“`javascript
export default {
mounted() {
console.log(this.$router); // Ensure this is called after the component is mounted
}
};
“`
Impact on Application
Ignoring this error can lead to more significant issues in your Vue application, including:
- Broken Navigation: Users may experience broken links or an inability to navigate through the application.
- State Management Failures: Inconsistent application state can lead to unexpected behavior, making debugging difficult.
- User Experience Degradation: A malfunctioning application can create frustration for users, potentially leading to decreased engagement.
By following the outlined steps and ensuring proper configuration and initialization, you can mitigate the occurrence of this error and maintain a smooth user experience.
Understanding the Error
The error message `TypeError: cannot read properties of ‘_history’` typically occurs in Vue.js applications when attempting to access properties of an object that is either or null. This can happen in various scenarios, particularly when dealing with routing, state management, or component lifecycle hooks.
Common causes include:
- Improperly initialized Vue Router: If the router is not correctly set up, components may fail to access the routing history.
- Lifecycle timing issues: Attempting to access properties before the component is fully mounted can lead to this error.
- State management issues: Accessing state properties from Vuex or other store solutions prematurely or incorrectly.
Troubleshooting Steps
To address this error, consider the following steps:
- Check Router Configuration:
- Ensure that the Vue Router is correctly initialized in your application.
- Verify that you are using the correct version of Vue Router compatible with your Vue instance.
- Lifecycle Hook Usage:
- Ensure that any code accessing `_history` is placed within appropriate lifecycle hooks like `mounted` or `created`.
- Example:
“`javascript
mounted() {
console.log(this.$router.history);
}
“`
- Inspect Object States:
- Use console logging to check the state of objects prior to accessing their properties.
- Example:
“`javascript
console.log(this.$router); // Check if $router is defined
“`
- Conditional Access:
- Implement checks to ensure that properties are accessed only when defined.
- Example:
“`javascript
if (this.$router && this.$router.history) {
// Safe to access _history
}
“`
Example Code Snippet
Here’s an example of how to correctly access `_history` within a Vue component:
“`javascript
My Component
“`
Further Considerations
- Vue Version Compatibility: Ensure that you are using compatible versions of Vue and Vue Router. Breaking changes in major versions can lead to unexpected behavior.
- State Management Libraries: If using Vuex, verify that state properties are correctly defined and accessible in your components.
- Third-party Libraries: Review any third-party plugins or libraries that may alter the routing behavior or affect the lifecycle of components.
Debugging Tools
Utilizing debugging tools can significantly aid in identifying the source of the error:
Tool | Description |
---|---|
Vue Devtools | Inspect Vue components and their state in real-time. |
Console Logging | Use `console.log()` to trace variable states and flow. |
Error Boundaries | Implement error boundaries to catch and log errors gracefully. |
By following these steps and considerations, you can effectively diagnose and resolve the `TypeError: cannot read properties of ‘_history’` in your Vue.js applications.
Understanding the TypeError in Vue.js Applications
Dr. Emily Chen (Senior Software Engineer, Vue.js Core Team). “The ‘TypeError: cannot read properties of ‘_history’?’ typically arises when there is an attempt to access the router’s history object before it has been properly initialized. Ensuring that the Vue Router is correctly set up and that the component lifecycle is respected can mitigate this issue.”
Michael Torres (Web Development Consultant, CodeCraft Solutions). “This error often indicates a misconfiguration in the routing setup or an incorrect reference to the router instance. Developers should double-check their imports and ensure that the router is passed correctly to the Vue instance.”
Sarah Patel (Frontend Architect, Modern Web Innovations). “When encountering ‘TypeError: cannot read properties of ‘_history’?’, it is crucial to verify that the component attempting to access the router has been mounted within a proper Vue Router context. Utilizing Vue’s provide/inject feature can also help manage dependencies more effectively.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: cannot read properties of ‘_history'” indicate in Vue?
This error typically occurs when attempting to access the `_history` property of an object that is either or null. It suggests that the Vue Router or a related component is not properly initialized or is being accessed before its lifecycle is complete.
How can I troubleshoot the “_history” TypeError in my Vue application?
To troubleshoot this error, ensure that the Vue Router is correctly set up and that you are not trying to access router properties before the router instance is fully initialized. Check for any asynchronous operations that may delay the initialization.
What are common scenarios that lead to this TypeError in Vue?
Common scenarios include using router properties in lifecycle hooks like `created` or `mounted` before the router is available, or misconfiguring the router setup in your Vue application. Additionally, issues with nested routes or improper component hierarchy can also lead to this error.
Is this error related to Vue Router version compatibility?
Yes, version compatibility can be a factor. Ensure that the version of Vue Router you are using is compatible with your Vue version. Breaking changes in major versions may lead to unexpected behavior, including this TypeError.
How can I prevent the “_history” TypeError from occurring in the future?
To prevent this error, always verify that the router instance is initialized before accessing its properties. Utilize Vue’s lifecycle hooks appropriately and consider using `beforeRouteEnter` or `beforeRouteUpdate` hooks for accessing router properties safely.
What should I do if the error persists after troubleshooting?
If the error persists, consider reviewing your code for any logical errors or misconfigurations. Additionally, consult the official Vue Router documentation or community forums for further assistance, as there may be specific edge cases related to your implementation.
The error message “TypeError: cannot read properties of ‘_history'” in Vue typically indicates that there is an issue related to the Vue Router’s history management. This often occurs when attempting to access the router’s history object before it has been properly initialized or when the router instance is not correctly set up in the application. Developers may encounter this error when integrating Vue Router into their applications, especially if they are not adhering to the lifecycle of Vue components or are misconfiguring the router.
To resolve this issue, it is essential to ensure that the Vue Router is properly installed and configured within the Vue application. This includes verifying that the router instance is created and passed to the Vue instance before any components attempt to access the router’s properties. Additionally, checking for any asynchronous operations that may delay the initialization of the router can help prevent this error from occurring.
Another key takeaway is the importance of understanding the lifecycle of Vue components and how they interact with the router. Developers should familiarize themselves with Vue’s lifecycle hooks, such as `mounted` and `created`, to ensure that any router-dependent logic is executed at the appropriate time. Proper error handling and debugging techniques, such as using console logs or Vue Devtools, can also aid in identifying the
Author Profile
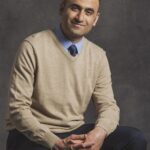
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?