What Are Libraries in Python and How Do They Enhance Your Coding Experience?
In the vast landscape of programming, Python stands out as a versatile and user-friendly language, beloved by beginners and seasoned developers alike. One of the key features that contribute to Python’s popularity is its extensive collection of libraries. But what exactly are these libraries, and how do they transform the way we write code? Libraries in Python are more than just collections of pre-written code; they are powerful tools that enable developers to streamline their workflows, enhance functionality, and tackle complex tasks with ease. As we delve deeper into the world of Python libraries, we will uncover their significance, explore different types, and demonstrate how they can elevate your programming experience.
At their core, Python libraries are bundles of modules and functions that provide specific functionalities, allowing programmers to avoid reinventing the wheel. With thousands of libraries available, ranging from data analysis and machine learning to web development and automation, Python empowers developers to leverage existing solutions and focus on innovation. This not only accelerates the development process but also encourages collaboration and knowledge sharing within the programming community.
Moreover, the modular nature of libraries means that they can be easily integrated into projects, enabling developers to build robust applications without extensive groundwork. Whether you are looking to visualize data, implement machine learning algorithms, or create web applications, Python libraries offer
Understanding Python Libraries
Python libraries are collections of pre-written code that facilitate various programming tasks, allowing developers to implement functionality without having to write everything from scratch. These libraries can encompass a wide range of functionalities, from data manipulation to web development, scientific computing, and more.
Libraries in Python are typically structured as packages, which can be imported into a Python script using the `import` statement. This modularity promotes code reuse and enhances productivity, making it easier for developers to focus on core logic rather than boilerplate code.
Types of Python Libraries
Python libraries can be categorized based on their applications. Here are some common types:
- Standard Libraries: These are included with Python and provide basic functionalities. Examples include:
- `math`: Mathematical functions
- `datetime`: Manipulating dates and times
- `os`: Operating system interactions
- Third-Party Libraries: These are developed by the community and can be installed via package managers like `pip`. Examples include:
- `NumPy`: Numerical computing
- `Pandas`: Data analysis and manipulation
- `Flask`: Web development framework
- Frameworks: While technically libraries, frameworks provide a robust structure for building applications. They often dictate the architecture of your code. Examples include:
- `Django`: High-level web framework
- `TensorFlow`: Machine learning framework
Popular Python Libraries
Below is a table highlighting some of the most popular Python libraries along with their primary use cases:
Library | Use Case |
---|---|
NumPy | Numerical computing and array manipulation |
Pandas | Data manipulation and analysis |
Matplotlib | Data visualization |
Requests | HTTP requests and web interactions |
Scikit-learn | Machine learning algorithms |
Flask | Lightweight web framework |
How to Install and Use Python Libraries
To make use of third-party libraries, you generally start by installing them using `pip`, Python’s package manager. The command is straightforward:
“`
pip install library_name
“`
After installation, you can import the library in your code as follows:
“`python
import library_name
“`
This allows you to access the functionalities provided by the library, enabling you to integrate them seamlessly into your projects.
In addition to importing entire libraries, you can also import specific functions or classes, which can help reduce memory usage and improve code clarity:
“`python
from library_name import specific_function
“`
By leveraging libraries, Python developers can significantly enhance their coding efficiency and minimize the time required for development.
Understanding Libraries in Python
In Python, libraries are collections of pre-written code that provide various functionalities, enabling developers to implement complex tasks without having to write extensive code from scratch. Libraries serve as modules that can be imported into Python programs, allowing for code reuse and modularity.
Types of Libraries
Python libraries can be categorized into several types based on their functionalities:
- Standard Libraries: Included with Python installations, these libraries provide essential tools and functionalities for everyday programming tasks.
- Third-Party Libraries: Developed by the community, these libraries can be installed via package managers like pip. They extend Python’s capabilities significantly.
- Custom Libraries: Developers can create their own libraries to encapsulate specific functionality, which can then be reused across multiple projects.
Popular Python Libraries
The following table highlights some of the most widely used Python libraries along with their primary purposes:
Library | Purpose |
---|---|
NumPy | Numerical computing and array manipulation |
Pandas | Data manipulation and analysis |
Matplotlib | Data visualization and plotting |
Scikit-learn | Machine learning and data mining |
TensorFlow | Deep learning and neural network training |
Flask | Web development and microservices |
Requests | HTTP requests handling |
Beautiful Soup | Web scraping and parsing HTML/XML documents |
Installing Libraries
To use third-party libraries, they must first be installed. The most common method for installation is using pip, Python’s package manager. The command syntax is as follows:
“`
pip install library_name
“`
For instance, to install the Pandas library, one would use:
“`
pip install pandas
“`
Importing Libraries
Once installed, libraries can be imported into a Python script using the `import` statement. Here are some common ways to import libraries:
- Import the entire library:
“`python
import numpy
“`
- Import with an alias for convenience:
“`python
import pandas as pd
“`
- Import specific functions or classes:
“`python
from math import sqrt
“`
Using Libraries
After importing, the functions and classes from the libraries can be utilized in your code. For example:
“`python
import numpy as np
Creating an array using NumPy
array = np.array([1, 2, 3, 4])
print(array)
“`
This code snippet demonstrates how to leverage the NumPy library to create an array, showcasing the simplicity and power of utilizing libraries in Python programming.
Benefits of Using Libraries
The advantages of using libraries in Python include:
- Time Efficiency: Reduces the amount of code that needs to be written.
- Community Support: Libraries often come with extensive documentation and community support.
- Enhanced Functionality: Provides advanced features that may not be feasible to implement independently.
- Consistency: Encourages best practices and standardized methods across different projects.
By understanding and utilizing libraries, developers can significantly enhance their productivity and the quality of their code in Python.
Understanding Python Libraries: Insights from Experts
Dr. Emily Chen (Senior Data Scientist, Tech Innovations Inc.). “Python libraries are essential tools that provide pre-written code to simplify complex programming tasks. They allow developers to leverage existing solutions, thereby accelerating the development process and enhancing productivity.”
Michael Thompson (Software Engineer, Open Source Advocate). “In Python, libraries serve as building blocks for software development. They encapsulate functionality that can be reused across different projects, which not only saves time but also promotes code maintainability and collaboration among developers.”
Sarah Patel (Python Educator, Code Academy). “Understanding how to effectively utilize Python libraries is crucial for any aspiring programmer. These libraries cover a wide range of applications, from data analysis to web development, making Python a versatile language for various domains.”
Frequently Asked Questions (FAQs)
What are libraries in Python?
Libraries in Python are collections of pre-written code that provide specific functionality, allowing developers to perform tasks without having to write code from scratch. They can include modules, packages, and functions that simplify complex programming tasks.
How do I install a library in Python?
You can install a library in Python using the package manager `pip`. For example, you can run the command `pip install library_name` in your terminal or command prompt to download and install the desired library.
What are some popular libraries in Python?
Some popular libraries in Python include NumPy for numerical computations, Pandas for data manipulation and analysis, Matplotlib for data visualization, and TensorFlow for machine learning. Each library serves specific purposes and enhances Python’s capabilities.
Can I create my own library in Python?
Yes, you can create your own library in Python by organizing your code into modules and packages. You can then distribute your library using tools like `setuptools` or `distutils`, allowing others to install and use it.
What is the difference between a module and a library in Python?
A module is a single file containing Python code, while a library is a collection of modules packaged together. Libraries provide a broader range of functionalities and can include multiple modules that serve related purposes.
How do I use a library in my Python project?
To use a library in your Python project, you first need to install it. After installation, you can import the library or specific modules/functions from it into your script using the `import` statement, allowing you to access its functionalities.
In summary, libraries in Python are pre-written code modules that provide a wide range of functionalities, enabling developers to perform various tasks without having to write code from scratch. These libraries can be categorized into standard libraries, which come bundled with Python, and third-party libraries, which can be installed via package managers like pip. The extensive ecosystem of Python libraries supports diverse applications, from web development and data analysis to machine learning and scientific computing.
One of the key takeaways is the importance of leveraging libraries to enhance productivity and efficiency in programming. By utilizing existing libraries, developers can save time and reduce the likelihood of errors, allowing them to focus on higher-level application logic rather than low-level implementation details. This practice not only accelerates development cycles but also encourages best practices through the use of well-tested and community-supported code.
Additionally, the Python community actively contributes to the growth of its library ecosystem. With thousands of libraries available, developers can find specialized tools tailored to their specific needs. This vast selection fosters innovation and collaboration, as developers can share their own libraries or contribute to existing ones, further enriching the Python programming landscape.
Author Profile
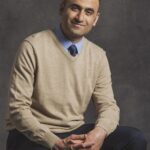
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?