Why Are Control Structures Essential in JavaScript?
### Introduction
In the dynamic world of programming, control structures serve as the backbone of logical flow and decision-making. When it comes to JavaScript, a language that powers the majority of the web, understanding control structures is essential for crafting efficient and effective code. These structures allow developers to dictate how their programs respond to various conditions, enabling a level of interactivity and functionality that is crucial in modern web applications. Whether you’re building a simple webpage or a complex web application, mastering control structures will empower you to create more sophisticated and user-friendly experiences.
Control structures in JavaScript encompass a variety of tools that dictate the flow of execution within a program. From conditional statements that allow your code to make decisions based on specific criteria, to loops that enable repetitive tasks, these structures are fundamental to writing logical and efficient code. They help programmers manage the complexity of their applications by providing a clear pathway for execution, making it easier to implement features like user interactions, data processing, and error handling.
By leveraging control structures, developers can create programs that not only respond to user input but also adapt to changing conditions in real time. This adaptability is what makes JavaScript a powerful language for web development. As we delve deeper into the various types of control structures available in JavaScript, you’ll discover how they
Understanding Control Structures in JavaScript
Control structures in JavaScript are fundamental programming constructs that dictate the flow of execution of code blocks. They allow developers to create dynamic and responsive applications by enabling decision-making, looping, and branching based on conditions. The main types of control structures include conditional statements, loops, and control flow statements.
Conditional Statements
Conditional statements execute different code paths based on specified conditions. The primary types of conditional statements in JavaScript include:
– **if statement**: Evaluates a condition and executes a block of code if the condition is true.
– **else statement**: Follows an if statement and executes a block of code if the condition is .
– **else if statement**: Tests additional conditions if the previous if statement evaluates to .
– **switch statement**: Evaluates an expression and executes code blocks based on matching case values.
Example of an if-else statement:
javascript
let score = 85;
if (score >= 90) {
console.log(“Grade: A”);
} else if (score >= 80) {
console.log(“Grade: B”);
} else {
console.log(“Grade: C”);
}
Loops
Loops are control structures that allow code to be executed repeatedly based on a condition. JavaScript provides several types of loops:
- for loop: Repeats a block of code a specified number of times.
- while loop: Repeats a block of code as long as a specified condition is true.
- do…while loop: Similar to the while loop, but the block of code is executed at least once before the condition is tested.
Example of a for loop:
javascript
for (let i = 0; i < 5; i++) {
console.log(i);
}
Control Flow Statements
Control flow statements manage the execution order of code. They help in altering the flow based on specific conditions. Key control flow statements include:
- break: Exits a loop or switch statement.
- continue: Skips the current iteration of a loop and moves to the next iteration.
- return: Exits a function and optionally returns a value.
Comparison of Control Structures
The following table summarizes the primary control structures in JavaScript along with their purposes:
Control Structure | Purpose |
---|---|
if | Executes a block of code if a condition is true. |
else | Executes a block of code if the preceding if condition is . |
switch | Executes code blocks based on matching case values. |
for | Repeats a block of code a set number of times. |
while | Repeats a block of code as long as the condition is true. |
do…while | Executes a block of code at least once, then continues based on a condition. |
The effective use of control structures enhances code readability, maintainability, and logic flow, enabling developers to create complex applications that respond accurately to user input and system states.
Purpose of Control Structures in JavaScript
Control structures in JavaScript are essential for managing the flow of execution in a program. They enable developers to dictate how and when certain blocks of code are executed, allowing for dynamic behavior based on conditions or iterations.
Types of Control Structures
JavaScript primarily utilizes three types of control structures:
- Conditional Statements: These allow code to execute only if certain conditions are met.
- `if` statement
- `else` statement
- `else if` statement
- `switch` statement
- Looping Structures: These enable repeated execution of a block of code while a condition remains true or for a specific number of iterations.
- `for` loop
- `while` loop
- `do…while` loop
- `for…in` loop (for iterating over object properties)
- `for…of` loop (for iterating over iterable objects)
- Jump Statements: These alter the flow of control within loops and functions.
- `break`: Exits a loop or switch statement.
- `continue`: Skips the current iteration of a loop and proceeds to the next iteration.
- `return`: Exits a function and optionally returns a value.
Use Cases for Control Structures
Control structures are utilized in various scenarios, including:
- Decision Making: To execute different blocks of code based on specific conditions.
- Data Handling: To process arrays or collections of data, applying operations like filtering or transforming.
- User Interaction: To respond dynamically to user inputs, such as validating form submissions.
- Error Handling: To implement logic for managing exceptions and errors in code execution.
Examples of Control Structures
Below are examples illustrating the use of different control structures:
**Conditional Statement Example**:
javascript
let temperature = 30;
if (temperature > 25) {
console.log(“It’s a hot day.”);
} else if (temperature < 15) {
console.log("It's a cold day.");
} else {
console.log("It's a pleasant day.");
}
Looping Structure Example:
javascript
let fruits = [“apple”, “banana”, “cherry”];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
Jump Statement Example:
javascript
for (let i = 0; i < 10; i++) {
if (i === 5) {
continue; // Skip the iteration when i is 5
}
console.log(i);
}
Best Practices for Control Structures
To ensure clarity and maintainability in code, consider the following best practices:
- Keep conditions simple: Complex conditions can be hard to read. Break them down into smaller functions if necessary.
- Avoid deep nesting: Use early returns in functions to minimize nesting and enhance readability.
- Use descriptive variable names: This aids in understanding the purpose of each control structure.
- Comment your logic: Provide context for complex logic to assist future developers in understanding your code.
By leveraging control structures effectively, developers can create responsive, efficient, and manageable JavaScript applications.
The Role of Control Structures in JavaScript Development
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). Control structures are fundamental in JavaScript as they dictate the flow of execution based on conditions. They enable developers to create dynamic and responsive applications by allowing for decision-making processes within the code, such as executing different blocks based on user input or application state.
Michael Thompson (JavaScript Framework Specialist, CodeCraft Academy). The use of control structures in JavaScript is essential for managing complex logic. They provide the necessary tools for looping through data, making conditional decisions, and handling asynchronous operations, which are critical in modern web development to ensure a seamless user experience.
Sarah Patel (Lead Frontend Developer, Web Solutions Group). Control structures not only enhance code readability but also improve maintainability. By structuring code with clear conditional statements and loops, developers can easily modify and extend functionality, which is vital in collaborative environments where multiple developers work on the same codebase.
Frequently Asked Questions (FAQs)
What are control structures in JavaScript?
Control structures in JavaScript are constructs that dictate the flow of execution in a program. They allow developers to control the order in which statements are executed based on certain conditions.
What do we use control structures for in JavaScript?
Control structures are used to implement decision-making, looping, and branching logic in JavaScript. They enable the execution of specific code blocks based on conditions, repetition of code, and managing the flow of the program.
What are the main types of control structures in JavaScript?
The main types of control structures in JavaScript include conditional statements (like `if`, `else`, and `switch`), loops (such as `for`, `while`, and `do…while`), and branching statements (like `break` and `continue`).
How do conditional statements work in JavaScript?
Conditional statements evaluate boolean expressions and execute specific code blocks based on whether the condition is true or . For instance, an `if` statement runs a block of code if the condition is met, while an `else` statement provides an alternative execution path.
What is the purpose of loops in JavaScript?
Loops in JavaScript are used to execute a block of code repeatedly as long as a specified condition is true. They are essential for tasks that require iteration, such as processing items in an array or performing repetitive calculations.
Can you explain the difference between `break` and `continue` statements?
The `break` statement terminates the current loop or switch statement, causing execution to jump to the next statement following the loop. In contrast, the `continue` statement skips the current iteration of a loop and proceeds to the next iteration, allowing the loop to continue executing.
Control structures in JavaScript are essential tools that allow developers to dictate the flow of execution within their programs. These structures enable the implementation of conditional logic, iteration, and branching, which are fundamental for creating dynamic and responsive applications. By utilizing control structures such as if-else statements, switch cases, loops (for, while, and do-while), and try-catch blocks, developers can manage how their code executes based on varying conditions and inputs.
One of the primary purposes of control structures is to facilitate decision-making in code. They allow developers to execute specific blocks of code based on certain criteria, enabling the application to respond appropriately to user input or other variables. This capability is vital for creating interactive web applications that require real-time responses to user actions.
Moreover, control structures enhance code efficiency and readability. By employing loops, developers can perform repetitive tasks without the need for redundant code, thereby reducing the likelihood of errors and improving maintainability. Additionally, the use of structured control flow promotes clearer logic, making it easier for developers to understand and modify their code in the future.
In summary, control structures are a cornerstone of JavaScript programming, providing the necessary framework for managing the execution flow of applications. Their ability
Author Profile
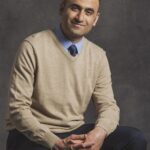
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?