What Does a Colon Do in Python? Understanding Its Role in Code Syntax
In the world of Python programming, every character has its purpose, but few are as pivotal as the humble colon. Often overlooked by beginners, this small yet mighty symbol plays a crucial role in defining the structure and flow of Python code. Whether you’re crafting a simple function, setting up a conditional statement, or organizing your code into loops, the colon is an essential component that helps to delineate blocks of code and clarify their relationships. Understanding its significance can elevate your coding skills and enhance your ability to write clear, efficient Python scripts.
At its core, the colon serves as a syntactical marker that indicates the start of an indented block of code. This indented block is where the magic happens—it’s where you define what should occur under specific conditions or within particular structures. For instance, when you define a function or an if statement, the colon signals that the subsequent lines will be part of that logical grouping. This not only aids in readability but also enforces a clear hierarchy within your code, allowing Python to interpret your intentions accurately.
Moreover, the use of colons extends beyond just functions and conditionals; they are integral to loops, class definitions, and even dictionary comprehensions. By mastering the role of the colon in Python, you can unlock a deeper understanding of how
Understanding the Role of Colons in Python
Colons in Python serve multiple purposes, primarily indicating the start of an indented block of code. They are essential in defining the structure of control flow statements, functions, classes, and more.
Control Flow Statements
In control flow statements, colons are used to signify the beginning of a block of code that will be executed conditionally. Common statements include `if`, `elif`, and `else`. The colon follows the condition or expression and precedes the indented block of code.
For example:
“`python
if condition:
code block to execute if condition is true
“`
The use of colons ensures that the subsequent indented lines are associated with the control structure.
Defining Functions and Classes
When defining a function or a class, the colon indicates that the following indented lines will contain the body of the function or class.
For example:
“`python
def function_name(parameters):
function body
“`
In the case of classes:
“`python
class ClassName:
class body
“`
Here, the colon clearly separates the definition of the function or class from its implementation.
Data Structures and Dictionary Comprehensions
Colons are also used in defining dictionaries, where they separate keys from values. In dictionary comprehensions, colons play a role similar to that in standard dictionary definitions.
Example of a dictionary:
“`python
my_dict = {
‘key1’: ‘value1’,
‘key2’: ‘value2’
}
“`
In dictionary comprehensions:
“`python
squared = {x: x**2 for x in range(5)} Creates a dictionary of squares
“`
Table of Colons Usage in Python
Context | Example | Description |
---|---|---|
Control Flow | if condition: | Begins a block of code that executes if the condition is true. |
Function Definition | def my_function(): | Indicates the start of the function body. |
Class Definition | class MyClass: | Starts the class body. |
Dictionary | {‘key’: ‘value’} | Separates key and value in a dictionary. |
Conclusion on Colons
The colon is a critical syntax element in Python, helping to define the structure and flow of the code. Understanding its use is essential for writing clean, readable, and functional Python code.
Understanding the Role of Colons in Python
In Python, colons play a pivotal role in defining the structure and flow of the code. They are used in various contexts, primarily to denote the beginning of an indented block of code. Here are the key usages of colons:
Control Structures
Colons are essential in control structures, such as `if` statements, `for` and `while` loops, and `try` blocks. The colon signifies that the subsequent lines will be part of the block that executes conditionally or iteratively.
- If Statement:
“`python
if condition:
Code block executed if condition is True
“`
- For Loop:
“`python
for item in iterable:
Code block executed for each item
“`
- While Loop:
“`python
while condition:
Code block executed while condition is True
“`
- Try Block:
“`python
try:
Code that may raise an exception
“`
Function and Class Definitions
When defining functions and classes, colons are used to indicate that a block of code will follow, which will define the function’s or class’s behavior.
- Function Definition:
“`python
def function_name(parameters):
Function body
“`
- Class Definition:
“`python
class ClassName:
Class body
“`
Dictionaries and Slices
Colons also appear in dictionaries and slicing operations, serving different purposes.
- Dictionaries: In dictionaries, colons separate keys from values.
“`python
my_dict = {
‘key1’: ‘value1’,
‘key2’: ‘value2’
}
“`
- Slicing: In slicing operations, colons define the start and end of the slice.
“`python
my_list = [0, 1, 2, 3, 4, 5]
sliced_list = my_list[1:4] Results in [1, 2, 3]
“`
Lambda Functions
In lambda functions, colons separate the parameters from the expression.
- Lambda Function Example:
“`python
lambda x: x + 1 A function that adds 1 to x
“`
Syntax and Indentation Requirements
The presence of a colon necessitates that the subsequent lines are indented. Python relies on indentation to define blocks of code, making colons crucial for maintaining the correct structure.
Context | Example |
---|---|
If Statement | `if x > 0:\n print(“Positive”)` |
For Loop | `for i in range(5):\n print(i)` |
Function Definition | `def greet():\n print(“Hello”)` |
Class Definition | `class Dog:\n def bark(self):\n print(“Woof”)` |
Using colons properly is essential for the correct execution of Python programs, as they indicate where a new block begins, contributing to code readability and structure.
Understanding the Role of Colons in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Colons in Python serve as a crucial syntactical element that indicates the start of an indented block of code. This is particularly important for defining functions, loops, and conditional statements, as it establishes the scope of the code that follows.
Michael Thompson (Lead Python Developer, CodeCraft Solutions). The use of colons in Python is not merely a stylistic choice; it enforces readability and helps maintain the structure of the code. By clearly delineating blocks, colons allow developers to write cleaner and more maintainable code.
Sarah Lee (Python Educator and Author, LearnPythonToday). In Python, colons are essential for control flow statements such as if, for, and while. They signal the interpreter to expect a block of code that will execute based on the preceding condition, making them integral to the language’s design philosophy of simplicity and clarity.
Frequently Asked Questions (FAQs)
What does a colon do in Python?
A colon in Python is used to indicate the start of an indented block of code. It typically follows control flow statements such as `if`, `for`, `while`, and function definitions.
How is a colon used in defining functions?
In function definitions, a colon is placed at the end of the function header. It signifies the beginning of the function’s body, which must be indented.
Can you give an example of a colon in a conditional statement?
Certainly. In an `if` statement, the syntax looks like this:
“`python
if condition:
code block
“`
The colon indicates that the following indented lines are executed if the condition is true.
What is the significance of colons in dictionaries?
In Python dictionaries, colons are used to separate keys from their corresponding values. For example:
“`python
my_dict = {‘key’: ‘value’}
“`
Are colons used in any other contexts in Python?
Yes, colons are also used in list slicing and in defining classes. For example, in slicing:
“`python
my_list[1:5] This retrieves elements from index 1 to 4.
“`
Do colons affect the readability of Python code?
Yes, colons enhance the readability of Python code by clearly indicating the structure of control flow statements and function definitions, making it easier for developers to understand the code’s logic.
In Python, the colon (:) serves several critical functions that are fundamental to the language’s syntax and structure. Primarily, it is used to denote the beginning of an indented block of code that follows control flow statements such as if, for, while, and function definitions. This use of the colon helps to establish clear and organized code, making it easier to read and maintain. Without the colon, Python would not be able to differentiate between a statement and the block of code that it controls.
Additionally, the colon is employed in defining slices for lists, tuples, and strings. By using a colon within square brackets, programmers can extract specific portions of data, enhancing data manipulation capabilities. This versatility of the colon extends to dictionary comprehensions and lambda functions as well, where it separates keys from values and defines anonymous functions, respectively. Thus, the colon is integral to both control structures and data handling in Python.
Overall, the colon is a vital component of Python’s syntax that contributes to the language’s readability and functionality. Understanding its various applications is essential for anyone looking to write effective Python code. By mastering the use of the colon, programmers can leverage its capabilities to create more structured and efficient programs.
Author Profile
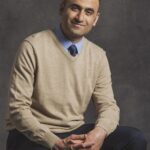
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?