What Does iloc Do in Python? A Deep Dive into DataFrame Indexing!
In the world of data manipulation and analysis, Python has emerged as a powerhouse, particularly with the advent of libraries like Pandas. Among the myriad of functions that Pandas offers, `iloc` stands out as a vital tool for those looking to navigate and extract data efficiently from DataFrames. Whether you’re a seasoned data scientist or a novice programmer, understanding how to leverage `iloc` can significantly enhance your ability to work with structured data. This article will delve into the functionality of `iloc`, providing you with the insights needed to harness its full potential.
At its core, `iloc` is an indexer for Pandas DataFrames that allows users to access rows and columns by their integer positions. This feature is particularly useful when you need to slice and dice your data without relying on the actual labels of the rows or columns. By using simple integer-based indexing, `iloc` empowers users to retrieve specific subsets of data, making it an essential function for data cleaning, transformation, and analysis.
Moreover, `iloc` supports a variety of indexing techniques, including single-value access, slicing, and even boolean indexing. This versatility makes it an indispensable tool for anyone working with large datasets, as it provides a straightforward way to manipulate data structures with precision. As we explore the intricacies of
Understanding iloc in Python
The `iloc` indexer in pandas is a powerful tool for selecting data by integer-location based indexing. It allows for selecting rows and columns from a DataFrame based on their numerical index positions. This is particularly useful when the exact labels of the rows or columns are unknown or when you want to access data programmatically.
Basic Usage of iloc
The general syntax for using `iloc` is as follows:
python
DataFrame.iloc[
Where:
- `
`: an integer index or a list of integer indices specifying which rows to select. - `
`: an integer index or a list of integer indices specifying which columns to select.
For example, if you have a DataFrame `df`, you can select the first row and first column like this:
python
value = df.iloc[0, 0]
This would return the value located at the first row and first column.
Selecting Rows and Columns
`iloc` can be used for various types of selections:
- Single Row Selection:
python
single_row = df.iloc[2] # Selects the third row
- Multiple Rows Selection:
python
multiple_rows = df.iloc[[0, 1, 2]] # Selects the first three rows
- Row Range Selection:
python
row_range = df.iloc[1:4] # Selects rows from index 1 to index 3
- Single Column Selection:
python
single_column = df.iloc[:, 1] # Selects the second column
- Multiple Columns Selection:
python
multiple_columns = df.iloc[:, [0, 2]] # Selects the first and third columns
- Column Range Selection:
python
column_range = df.iloc[:, 1:3] # Selects columns from index 1 to index 2
Advanced Indexing Techniques
In addition to basic indexing, `iloc` supports more complex selections:
- Boolean Indexing with iloc: You can combine boolean arrays with `iloc` for more refined selections.
- Negative Indexing: Similar to Python lists, you can use negative integers to count from the end of the DataFrame.
Example DataFrame
To illustrate the use of `iloc`, consider the following example DataFrame:
python
import pandas as pd
data = {
‘A’: [1, 2, 3, 4],
‘B’: [5, 6, 7, 8],
‘C’: [9, 10, 11, 12]
}
df = pd.DataFrame(data)
Index | A | B | C |
---|---|---|---|
0 | 1 | 5 | 9 |
1 | 2 | 6 | 10 |
2 | 3 | 7 | 11 |
3 | 4 | 8 | 12 |
Using `iloc`, various selections can be made:
- To get the value in the third row, second column:
python
value = df.iloc[2, 1] # Returns 7
- To get the first two rows of the DataFrame:
python
rows = df.iloc[:2] # Returns rows 0 and 1
Thus, `iloc` serves as a versatile tool for data manipulation and retrieval in pandas, enhancing the efficiency of data analysis workflows.
Understanding iloc in Python
The `iloc` attribute in pandas is a powerful tool for data manipulation, specifically used for integer-location based indexing. It allows users to select rows and columns from a DataFrame or Series by their integer positions.
Basic Functionality of iloc
`iloc` operates primarily on two dimensions: rows and columns. You can access data in the following ways:
- Selecting a single row:
python
df.iloc[0] # Selects the first row of the DataFrame
- Selecting a single column:
python
df.iloc[:, 1] # Selects the second column of the DataFrame
- Selecting multiple rows:
python
df.iloc[[0, 2, 4]] # Selects the first, third, and fifth rows
- Selecting multiple columns:
python
df.iloc[:, [0, 2]] # Selects the first and third columns
- Selecting a specific range of rows and columns:
python
df.iloc[1:4, 0:2] # Selects rows 1 to 3 and columns 0 to 1
Advanced Usage of iloc
In addition to basic indexing, `iloc` supports more complex operations:
– **Conditional selection**: You can combine boolean indexing with `iloc` for filtering.
python
df.iloc[(df[‘column_name’] > value).values] # Selects rows based on a condition
- Slicing: You can utilize slicing to extract parts of DataFrames or Series.
python
df.iloc[0:5, 0:3] # Selects the first five rows and the first three columns
- Negative indexing: Similar to Python lists, negative indices can be employed to access elements from the end.
python
df.iloc[-1] # Selects the last row
Common Use Cases
The `iloc` function is particularly useful in the following scenarios:
- Data cleaning: Quickly access and modify specific rows or columns.
- Data analysis: Extract subsets of data for analysis or visualization.
- Feature selection: Select specific features from a dataset when preparing machine learning models.
Examples of iloc in Action
The following table provides examples of how `iloc` can be applied in different contexts:
Operation | Code Example | Description |
---|---|---|
Select first row | `df.iloc[0]` | Retrieves the first row of the DataFrame. |
Select last two rows | `df.iloc[-2:]` | Retrieves the last two rows. |
Select specific cells | `df.iloc[1, 2]` | Retrieves the value at row 1, column 2. |
Select multiple non-consecutive rows | `df.iloc[[0, 2, 4]]` | Retrieves the first, third, and fifth rows. |
Select all rows for specific columns | `df.iloc[:, [0, 1]]` | Retrieves all rows for the first two columns. |
Performance Considerations
While using `iloc` is generally efficient, consider the following:
- Avoid excessive slicing: Large datasets may lead to performance degradation if slicing is overused.
- Memory management: Keep an eye on memory usage when dealing with large DataFrames, especially when creating copies.
- Use boolean indexing wisely: Combine `iloc` with boolean conditions judiciously to maintain performance.
By leveraging the capabilities of `iloc`, users can effectively navigate and manipulate data within pandas DataFrames, enhancing their data analysis workflows.
Understanding the Functionality of iloc in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The iloc function in Python is a powerful tool within the pandas library that allows users to access rows and columns by integer-location based indexing. This is particularly useful for data manipulation and analysis, as it provides a straightforward way to retrieve data without needing to reference labels.”
Michael Chen (Software Engineer, Data Solutions Corp.). “By utilizing iloc, developers can efficiently slice data frames, enabling them to extract specific subsets of data. This integer-based indexing is essential for tasks such as filtering and reshaping data sets, making it a fundamental skill for anyone working with pandas.”
Sarah Patel (Machine Learning Researcher, AI Analytics Group). “Understanding how iloc operates is crucial for effective data analysis in Python. It allows for precise control over data selection, which is vital when preparing datasets for machine learning models, ensuring that only the relevant features and samples are utilized.”
Frequently Asked Questions (FAQs)
What does iloc do in Python?
iloc is a Pandas method used for integer-location based indexing, allowing users to select rows and columns by their integer positions in a DataFrame or Series.
How do you use iloc to select a specific row?
To select a specific row using iloc, you can use the syntax `dataframe.iloc[row_index]`, where `row_index` is the integer position of the desired row.
Can iloc be used to select multiple rows at once?
Yes, iloc can select multiple rows by passing a list of indices or a slice. For example, `dataframe.iloc[[0, 2, 4]]` selects the first, third, and fifth rows.
How can you use iloc to select specific columns?
To select specific columns with iloc, use the syntax `dataframe.iloc[:, column_indices]`, where `column_indices` specifies the integer positions of the desired columns.
Is iloc inclusive of the endpoint when slicing?
No, iloc is exclusive of the endpoint when slicing. For example, `dataframe.iloc[0:3]` will return rows at index 0, 1, and 2, but not 3.
What happens if you use an index that is out of bounds with iloc?
Using an out-of-bounds index with iloc will raise an `IndexError`, indicating that the index is not valid for the DataFrame or Series.
The `iloc` function in Python, particularly within the pandas library, is a powerful tool for data manipulation and analysis. It allows users to access and modify data in a DataFrame by using integer-based indexing. This means that users can select rows and columns based on their numerical positions rather than their labels, which is particularly useful when the structure of the DataFrame is not known or when working with large datasets where labels may be cumbersome to use.
One of the key features of `iloc` is its ability to perform slicing operations, enabling users to extract specific subsets of data efficiently. For instance, users can retrieve a single row, multiple rows, or even specific ranges of rows and columns by specifying the appropriate index values. This flexibility makes `iloc` an essential function for data analysis tasks, as it streamlines the process of accessing and manipulating data.
In summary, the `iloc` function is a fundamental component of the pandas library that enhances data accessibility through integer-based indexing. Its versatility in slicing and selecting data positions makes it an invaluable resource for data scientists and analysts alike. Understanding how to effectively use `iloc` can significantly improve one’s ability to work with data in Python, facilitating more efficient data analysis and manipulation workflows.
Author Profile
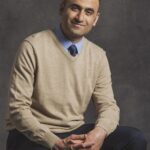
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?