What Does the Colon (:) Mean in Python? Understanding Its Role and Usage
In the world of Python programming, every character holds significance, and among them, the colon (`:`) stands out as a pivotal symbol. Whether you’re a novice coder just starting your journey or a seasoned developer looking to refine your skills, understanding the role of the colon is essential to mastering Python’s syntax. This seemingly simple character plays a crucial part in defining structures, controlling flow, and enhancing code readability. Join us as we delve into the multifaceted uses of the colon in Python, unlocking its secrets and empowering your coding prowess.
The colon is not just a punctuation mark; it serves as a gateway to various programming constructs in Python. From defining functions and classes to initiating loops and conditional statements, the colon signifies the beginning of a new block of code. Its presence indicates that what follows is a suite of statements that belong together, creating a clear and organized structure that is vital for both execution and comprehension.
Moreover, the colon plays an essential role in enhancing the readability of Python code, which is one of the language’s core philosophies. By establishing a clear visual cue for code blocks, the colon helps programmers quickly identify the scope and relationships within their code. As we explore the nuances of this character, you’ll discover how it not only influences the flow of execution but also contributes to
Understanding the Colon in Python
In Python, the colon (`:`) serves multiple purposes, primarily indicating the start of a block of code. It is a crucial syntax element that helps define the structure and flow of the program. Below are the main contexts where the colon is utilized:
- Function Definitions: The colon is used at the end of the function header to denote that the following indented lines are part of the function body.
python
def my_function():
print(“Hello, World!”)
- Conditional Statements: In control flow statements such as `if`, `elif`, and `else`, the colon indicates the beginning of the block that executes when the condition is true.
python
if condition:
do_something()
- Loops: Similar to conditionals, the colon is used in `for` and `while` loops to signify the start of the loop’s body.
python
for i in range(5):
print(i)
- Class Definitions: When defining a class, the colon indicates the start of the class body.
python
class MyClass:
def __init__(self):
self.value = 0
- Dictionary Definitions: Within dictionaries, the colon separates keys from their corresponding values.
python
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
Colon in Slicing
The colon is also used in slicing operations on sequences like lists, tuples, and strings. Slicing allows for retrieving a portion of the sequence. The syntax for slicing is as follows:
python
sequence[start:stop:step]
- start: The index of the first element to include in the slice.
- stop: The index of the first element to exclude from the slice.
- step: The difference between each index in the slice.
For example:
python
my_list = [0, 1, 2, 3, 4, 5]
slice_example = my_list[1:5:2] # Output: [1, 3]
Table of Colon Uses in Python
Context | Usage | Example |
---|---|---|
Function Definitions | Indicates the start of the function body | def func_name(): |
Conditional Statements | Begins the block of code for true conditions | if condition: |
Loops | Starts the loop’s code block | for i in range(5): |
Class Definitions | Marks the start of the class body | class ClassName: |
Dictionaries | Separates keys from values | {'key': 'value'} |
Slicing | Defines the range of indices | my_list[1:5] |
In summary, the colon is a fundamental part of Python’s syntax, facilitating clear structure and readability in code. Understanding its usage is essential for writing effective Python programs.
Usage of `:` in Python
In Python, the colon (`:`) serves several essential functions across different contexts within the language. Its primary uses can be categorized as follows:
Defining Functions and Methods
When defining a function or a method, the colon is used to indicate the beginning of the function body. The syntax is as follows:
python
def function_name(parameters):
# function body
- Example:
python
def greet(name):
print(f”Hello, {name}!”)
Control Flow Statements
Colons are also pivotal in control flow statements like `if`, `for`, and `while`. They signal the start of a block of code that will execute based on a condition or iteration.
- Example with `if`:
python
if condition:
# block of code
- Example with `for`:
python
for item in iterable:
# block of code
Class Definitions
In class definitions, a colon indicates the start of the class body. The syntax mirrors that of functions.
- Example:
python
class MyClass:
def method(self):
# method body
Dictionaries
In Python dictionaries, the colon is used to separate keys from their corresponding values. The syntax is as follows:
python
my_dict = {
‘key1’: ‘value1’,
‘key2’: ‘value2’
}
- Example:
python
person = {
‘name’: ‘Alice’,
‘age’: 30
}
List Slicing
The colon is also utilized in list slicing to define a range of indices. The syntax is as follows:
python
list_name[start:stop:step]
- Example:
python
numbers = [0, 1, 2, 3, 4, 5]
sliced = numbers[1:4] # Output: [1, 2, 3]
Lambda Functions
In the context of lambda functions, the colon separates the parameters from the expression.
- Example:
python
square = lambda x: x ** 2
Type Hints
With the introduction of type hints in Python 3.5, the colon is used to annotate variable types and function return types.
- Example:
python
def add(a: int, b: int) -> int:
return a + b
Comprehensions
In list and dictionary comprehensions, the colon appears in the context of key-value pairs.
- Example for dictionary comprehension:
python
squares = {x: x ** 2 for x in range(5)} # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In summary, the colon plays a crucial role in various syntactic structures within Python, marking the beginning of code blocks, separating elements in dictionaries, and facilitating type annotations, among other uses. Understanding its significance is essential for writing clear and effective Python code.
Understanding the Significance of Colons in Python Programming
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). The colon in Python serves as a critical syntactical element that indicates the start of an indented block of code. This is particularly important in defining control structures such as loops and conditional statements, as well as in function and class definitions.
Michael Thompson (Lead Python Developer, CodeCraft Solutions). In Python, the colon is not merely a punctuation mark; it plays a pivotal role in enhancing code readability and structure. By signaling the beginning of a new block, it helps maintain clarity in the flow of the program, which is essential for effective collaboration among developers.
Sarah Patel (Python Instructor, Future Coders Academy). Understanding the use of the colon is fundamental for anyone learning Python. It is used in various contexts, such as defining slices in lists and dictionaries. Mastery of this simple yet powerful symbol can significantly improve a programmer’s ability to write clean and efficient code.
Frequently Asked Questions (FAQs)
What does the colon (:) signify in Python?
The colon (:) in Python is used to indicate the start of an indented block of code. It is commonly found in control structures like if statements, loops, and function definitions.
How is the colon (:) used in function definitions?
In function definitions, the colon follows the function signature to indicate the beginning of the function body. For example, in `def my_function():`, the colon precedes the indented block that contains the function’s code.
What role does the colon (:) play in slicing lists or strings?
In slicing, the colon separates the start and end indices. For instance, `my_list[1:3]` retrieves elements from index 1 to index 2, excluding index 3.
Can the colon (:) be used in dictionary comprehensions?
Yes, in dictionary comprehensions, the colon is used to separate keys from values. For example, `{key: value for key, value in iterable}` creates a dictionary from the specified key-value pairs.
What does the colon (:) mean in a conditional expression?
In a conditional expression (also known as a ternary operator), the colon separates the two possible outcomes. The syntax is `value_if_true if condition else value_if_`, where the colon precedes the outcome.
Is the colon (:) used in any other contexts in Python?
Yes, the colon is also used in annotations, such as type hints in function signatures. For example, `def add(a: int, b: int) -> int:` uses colons to specify the expected types of parameters and the return type.
The colon (:) in Python serves multiple important functions that are crucial for understanding the syntax and structure of the language. Primarily, it is used to indicate the start of an indented block of code, which is essential for defining control structures such as functions, loops, and conditional statements. For instance, after a function definition or an if statement, the colon signals that the subsequent indented lines belong to that particular block, establishing a clear hierarchy and flow of execution.
Additionally, the colon is also utilized in dictionary definitions and slices. In dictionaries, it separates keys from their corresponding values, allowing for the creation of key-value pairs. In the context of slicing, the colon is used to specify a range of indices, enabling users to extract specific portions of lists or strings efficiently. This versatility makes the colon a fundamental component of Python’s syntax.
In summary, understanding the various uses of the colon in Python is essential for writing clear and effective code. Its role in defining code blocks, separating dictionary keys and values, and facilitating slicing operations highlights its significance in the language. Mastery of these concepts not only enhances coding proficiency but also contributes to better readability and maintainability of code.
Author Profile
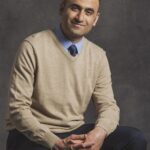
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?