Understanding the Role of the ‘for’ Index Value in Python Enums: What Does It Do?
### Introduction
In the world of Python programming, enums (short for enumerations) offer a powerful way to define a set of named values that can be used to represent distinct categories or states. But what happens when you need to access not just the names but also their corresponding index values? This is where the `for index, value in enum` construct comes into play, providing a streamlined method to iterate through your enumerated types. Understanding this functionality can significantly enhance your coding efficiency and clarity, especially when working with collections of related constants.
Enums in Python, introduced in version 3.4 through the `enum` module, allow developers to create symbolic names bound to unique, constant values. By utilizing the `for index, value in enum` pattern, programmers can easily loop through the enum members while simultaneously keeping track of their positions. This dual access to both the index and the value opens up a range of possibilities for data manipulation, making it easier to implement logic that depends on the order of elements or to perform operations that require both the name and its associated index.
As we delve deeper into this topic, we will explore the mechanics of enums in Python, how to effectively utilize the `for index, value in enum` syntax, and the practical applications that arise from this
Understanding Enum in Python
In Python, the `enum` module provides a way to create enumerations, which are a set of symbolic names bound to unique, constant values. Enumerations can be used to create more readable code by using names instead of numbers or strings. The `for index, value in enum` construct is particularly useful for iterating over these enumerations, allowing access to both the index and the corresponding value.
Using the Index with Enum
When you iterate through an enumeration, you can retrieve both the index and the value of each member. The index represents the position of the enum member within its definition, starting from zero. This can be particularly useful in scenarios where the order of enumeration members matters, or when you need to perform operations based on their positions.
For example, consider the following enumeration:
python
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
You can iterate through the enumeration while accessing both the index and the enum member like this:
python
for index, color in enumerate(Color):
print(f’Index: {index}, Color: {color.name}, Value: {color.value}’)
This code will produce the following output:
Index: 0, Color: RED, Value: 1
Index: 1, Color: GREEN, Value: 2
Index: 2, Color: BLUE, Value: 3
Benefits of Using Index Value in Enum
Utilizing the index value when working with enumerations offers several advantages:
- Clarity: Provides clear identification of the position of each enum member.
- Flexibility: Allows for operations that depend on the order of members.
- Ease of Use: Simplifies tasks such as looping through enums and accessing their properties.
Example Usage Table
To illustrate the benefits further, consider the following table that summarizes the index and value of various enum members.
Index | Color Name | Color Value |
---|---|---|
0 | RED | 1 |
1 | GREEN | 2 |
2 | BLUE | 3 |
In summary, using the `for index, value in enum` syntax in Python provides an efficient way to iterate over enumeration members while gaining access to their indices. This enhances code readability and allows for more versatile programming patterns.
Understanding the Index Value in Python Enums
In Python, the `enum` module provides a way to define enumerations, which are a set of symbolic names bound to unique, constant values. When defining an enumeration, each member can be automatically assigned an index value, which corresponds to its position in the enumeration. This feature is particularly useful for referencing the order of enumeration members.
How Index Values are Assigned
When you create an enumeration using the `Enum` class, Python automatically assigns integer values starting from 1 by default. However, you can also specify custom values for each member. Here’s how it works:
- Default behavior: If no values are provided, the first member is assigned 1, the second member 2, and so forth.
- Custom values: You can explicitly assign values to members, which can be integers, strings, or any other type.
Example of Default Index Assignment
python
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
In this example, the members `RED`, `GREEN`, and `BLUE` are assigned values of 1, 2, and 3, respectively.
Example of Custom Index Assignment
python
from enum import Enum
class Status(Enum):
PENDING = 0
IN_PROGRESS = 1
COMPLETED = 2
In the `Status` enum, custom values are specified for each member. The index values do not have to follow a specific pattern; they can be any integers.
Accessing Index Values
You can access both the name and the value of an enum member. The index value can be accessed via the `.value` attribute. Here’s an example:
python
print(Color.RED.value) # Output: 1
print(Status.COMPLETED.value) # Output: 2
Iterating Over Enum Members
You can iterate over the members of an enumeration, which allows you to access both their names and values easily.
python
for color in Color:
print(color, color.value)
This will produce:
Color.RED 1
Color.GREEN 2
Color.BLUE 3
Key Points to Remember
- The index values in enums are automatically assigned starting from 1 unless specified otherwise.
- Custom values can be assigned to enum members, which allows for flexibility in defining enums.
- Accessing the index value is done using the `.value` attribute.
Using Enums for Better Code Clarity
Enums enhance code readability and maintainability. Instead of using arbitrary constants, enums provide meaningful names that clarify the intent of the code. Here’s a summary of the advantages:
- Readability: Code is easier to read and understand.
- Maintainability: Changes to the enumeration only need to be made in one place.
- Type safety: Enums prevent invalid values from being used, reducing errors.
By using enums effectively, developers can write cleaner and more robust Python code.
Understanding the Role of Index Values in Python Enums
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, the `for index value` in an Enum serves as an integral part of the enumeration process. It allows developers to associate a unique integer with each enumerated value, facilitating easier comparisons and iterations. This is particularly useful in scenarios where enumerated types need to be stored in databases or transmitted over networks, as the integer representation is often more efficient.
Mark Thompson (Python Developer Advocate, Open Source Community). The index value in Python Enums provides a clear and concise way to manage a set of related constants. By default, the first value starts at 0, but developers can customize these values, which enhances readability and maintainability of the code. This feature is especially beneficial in large applications where clarity in constant definitions is paramount.
Linda Zhang (Lead Data Scientist, AI Solutions Group). Utilizing index values in Python Enums not only promotes code organization but also allows for the implementation of logic that depends on these values. For instance, when iterating over an Enum, one can easily access both the name and the index, making it simpler to implement algorithms that require positional data. This duality of access enhances the power and flexibility of Enums in data-driven applications.
Frequently Asked Questions (FAQs)
What is an Enum in Python?
An Enum (short for enumeration) in Python is a distinct data type that consists of a set of named values. It allows for the creation of symbolic names bound to unique, constant values, enhancing code readability and maintainability.
What does the ‘for index value in enum’ syntax mean?
The syntax ‘for index value in enum’ is used to iterate over the members of an Enum. During each iteration, ‘index’ refers to the current member’s index, while ‘value’ represents the member itself, allowing access to both the name and value of each Enum member.
How do you define an Enum in Python?
An Enum can be defined using the `Enum` class from the `enum` module. You create a subclass of `Enum` and define the members as class attributes, assigning them unique values.
Can you access the index of Enum members?
Yes, you can access the index of Enum members by using the `list()` function. For example, `list(MyEnum).index(MyEnum.MEMBER_NAME)` will return the index of the specified member.
What is the purpose of using indices in Enums?
Using indices in Enums allows for easier access and manipulation of Enum members in situations where their order is significant. It also facilitates operations such as sorting or filtering based on their position.
Are Enum members mutable in Python?
No, Enum members are immutable. Once defined, their names and values cannot be changed, ensuring the integrity and consistency of the Enum throughout the program.
In Python, the `enum` module provides a way to define enumerations, which are a set of symbolic names bound to unique, constant values. When you create an enumeration using the `Enum` class, each member of the enumeration is assigned an index value automatically, starting from zero. This index value serves as a unique identifier for each member within the enumeration, allowing for easy reference and comparison.
The index value can be particularly useful when you need to iterate over the enumeration or when you want to convert enumeration members into a list or other data structures. The ability to access these index values enhances the usability of enumerations in various programming scenarios, such as when implementing state machines or managing configurations where specific states or options need to be represented clearly.
Furthermore, developers can also define custom values for enumeration members if the default index values do not suffice for their application needs. This flexibility allows for the integration of more meaningful or context-specific values while still retaining the benefits of using enumerations. Overall, understanding the role of index values in Python enums can significantly improve code clarity and maintainability.
Author Profile
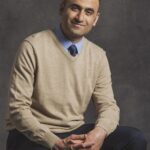
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?