What Is a Python Script and How Can It Enhance Your Coding Skills?
### Introduction
In the ever-evolving landscape of technology, programming languages have become the backbone of countless innovations, and Python stands out as a favorite among developers and enthusiasts alike. But what exactly is a Python script? This seemingly simple question opens the door to a world of possibilities, where lines of code can transform ideas into reality, automate mundane tasks, and even power complex applications. Whether you are a seasoned programmer or just dipping your toes into the coding waters, understanding Python scripts is essential for harnessing the full potential of this versatile language.
At its core, a Python script is a collection of instructions written in the Python programming language, designed to be executed by the Python interpreter. These scripts can range from simple commands that perform straightforward tasks to intricate programs that handle complex data processing or web development. The beauty of Python lies in its readability and simplicity, making it an ideal choice for both beginners and experienced developers looking to streamline their workflows.
As we delve deeper into the world of Python scripts, we will explore their structure, functionality, and the myriad of applications they can serve. From automating repetitive tasks to developing robust software solutions, Python scripts are a powerful tool in any programmer’s arsenal. Join us on this journey to uncover the nuances of Python scripting and discover how you can leverage
Components of a Python Script
A Python script is composed of various elements that work together to perform a specific task or set of tasks. Understanding these components can help in writing effective scripts.
- Variables: Variables are used to store data that can be referenced and manipulated throughout the script. They can hold different types of data, including integers, strings, and lists.
- Functions: Functions are reusable blocks of code that perform a specific operation. They can take parameters and return results, promoting code modularity and reuse.
- Control Structures: Control structures such as loops and conditional statements dictate the flow of the script. Common control structures include:
- `if`, `elif`, and `else` for decision-making
- `for` and `while` loops for iteration
- Libraries and Modules: Python scripts can import external libraries and modules that provide additional functionality. This allows developers to leverage pre-built functions and tools, which can save time and effort.
- Comments: Comments are crucial for documentation within scripts. They are ignored by the Python interpreter but provide clarity to anyone reading the code. They can be single-line (using `#`) or multi-line (enclosed in triple quotes).
Basic Structure of a Python Script
The basic structure of a Python script typically includes:
- Shebang Line: The first line often begins with `#!/usr/bin/env python3`, indicating the script should be run using Python.
- Import Statements: Any required libraries or modules are imported next.
- Function Definitions: Functions may be defined to organize code logically.
- Main Execution Block: The main code is usually encapsulated in a `if __name__ == “__main__”:` block, ensuring it runs only when the script is executed directly.
Here is a simple example of a Python script structure:
python
#!/usr/bin/env python3
import math
def calculate_area(radius):
return math.pi * radius ** 2
if __name__ == “__main__”:
radius = 5
area = calculate_area(radius)
print(f”The area of a circle with radius {radius} is {area:.2f}”)
Common Use Cases for Python Scripts
Python scripts are versatile and can be used in various domains. Here are some common use cases:
- Data Analysis: Automating data cleaning and analysis tasks using libraries like Pandas and NumPy.
- Web Scraping: Extracting data from websites using libraries such as Beautiful Soup and Scrapy.
- Automation: Automating repetitive tasks like file manipulation or report generation.
- Machine Learning: Implementing machine learning algorithms and models with libraries like scikit-learn and TensorFlow.
- Web Development: Creating backend services or APIs using frameworks like Flask or Django.
Use Case | Description | Popular Libraries |
---|---|---|
Data Analysis | Process and analyze large datasets. | Pandas, NumPy |
Web Scraping | Extract data from web pages. | Beautiful Soup, Scrapy |
Automation | Perform routine tasks automatically. | Selenium, PyAutoGUI |
Machine Learning | Build and train predictive models. | scikit-learn, TensorFlow |
Web Development | Create web applications and APIs. | Flask, Django |
Understanding these components and use cases can greatly enhance the effectiveness of Python scripts in various applications.
Definition of a Python Script
A Python script is a file containing Python code that is executed by the Python interpreter. Typically saved with a `.py` extension, these scripts can contain a sequence of commands, functions, and classes that allow for various functionalities, from simple calculations to complex web applications.
Characteristics of Python Scripts
Python scripts are characterized by several key features:
- Interpreted Language: Python is an interpreted language, meaning that scripts are executed line-by-line at runtime, making it easier to debug and test code.
- Readability: Python emphasizes readability, utilizing indentation to define code blocks rather than traditional braces or keywords.
- Dynamic Typing: Variables in Python do not require explicit declaration of type, allowing for more flexible code writing.
- Extensive Libraries: Python scripts can leverage a vast array of libraries and frameworks, enhancing functionality without the need to write extensive code from scratch.
Common Uses of Python Scripts
Python scripts can be employed in a variety of scenarios, including but not limited to:
- Web Development: Using frameworks like Flask and Django to create web applications.
- Data Analysis: Utilizing libraries such as Pandas and NumPy to process and analyze data sets.
- Automation: Writing scripts to automate repetitive tasks, such as file management or data entry.
- Machine Learning: Implementing algorithms with libraries like TensorFlow and scikit-learn for predictive modeling.
Structure of a Python Script
A typical Python script may include the following components:
Component | Description |
---|---|
Shebang | `#!/usr/bin/env python3` at the top of the script for execution context. |
Comments | Lines starting with `#` for annotations that explain code behavior. |
Imports | Statements to include libraries, e.g., `import math`, `from datetime import datetime`. |
Functions | Defined with the `def` keyword to encapsulate reusable code blocks. |
Main Execution | A block typically guarded by `if __name__ == “__main__”:` to run the script. |
Executing a Python Script
To execute a Python script, follow these steps:
- Open a terminal or command prompt.
- Navigate to the directory containing the script using the `cd` command.
- Run the script using the command:
python script_name.py
Replace `script_name.py` with the actual name of your script.
Debugging Python Scripts
Debugging is a crucial aspect of script development. Common methods include:
- Print Statements: Inserting `print()` functions to display variable values at different execution points.
- Python Debugger (pdb): Using the built-in debugger to set breakpoints and step through code interactively.
- IDE Tools: Utilizing integrated development environments (IDEs) that provide debugging tools, such as PyCharm or Visual Studio Code.
Best Practices for Writing Python Scripts
Consider the following best practices to enhance the quality of your Python scripts:
- Follow PEP 8 Guidelines: Adhere to the Python Enhancement Proposal (PEP) 8 for style consistency.
- Use Meaningful Variable Names: Choose descriptive names that convey the purpose of the variable.
- Modularize Code: Break down scripts into functions and modules for better organization and reusability.
- Handle Exceptions: Implement error handling with `try` and `except` blocks to manage potential runtime errors gracefully.
Understanding Python Scripts Through Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A Python script is a file containing Python code that can be executed to perform a specific task or a series of tasks. It is an essential tool for automating processes, data analysis, and web development, making Python a versatile language in the programming landscape.”
Mark Thompson (Lead Data Scientist, Data Insights Lab). “In the realm of data science, Python scripts are invaluable. They allow data professionals to manipulate data, build models, and visualize results efficiently. The simplicity and readability of Python scripts make them accessible to both beginners and experienced programmers alike.”
Linda Garcia (Professor of Computer Science, University of Technology). “Teaching students about Python scripts is crucial in modern programming education. These scripts exemplify fundamental programming concepts such as control structures, functions, and libraries, providing a practical foundation for students to build upon in their coding journey.”
Frequently Asked Questions (FAQs)
What is a Python script?
A Python script is a file containing a sequence of Python code that is executed by the Python interpreter. It typically has a `.py` file extension and can include functions, classes, and executable statements.
How do you create a Python script?
To create a Python script, use a text editor or an Integrated Development Environment (IDE) to write your Python code. Save the file with a `.py` extension, and it can be executed using the command line or terminal.
What are the common uses of Python scripts?
Python scripts are commonly used for automation, data analysis, web development, machine learning, and scripting tasks that require repetitive actions. They are versatile and can interact with various systems and applications.
Can Python scripts be run on any operating system?
Yes, Python scripts are cross-platform and can be run on any operating system that has the Python interpreter installed, including Windows, macOS, and Linux.
What libraries can be used in Python scripts?
Python scripts can utilize a wide range of libraries, such as NumPy for numerical computations, Pandas for data manipulation, Flask for web development, and TensorFlow for machine learning, among many others.
How do you execute a Python script?
To execute a Python script, open a terminal or command prompt, navigate to the directory containing the script, and run the command `python script_name.py`, replacing `script_name.py` with the actual name of your script.
A Python script is a file containing a sequence of instructions written in the Python programming language. These scripts are typically saved with a .py extension and can be executed to perform a wide range of tasks, from simple calculations to complex data analysis and web development. The versatility of Python, combined with its readability and ease of use, makes it a popular choice among both novice and experienced programmers for automating tasks and developing applications.
One of the key advantages of using Python scripts is their ability to leverage the extensive libraries and frameworks available within the Python ecosystem. This allows developers to implement functionalities without having to write code from scratch, thereby enhancing productivity and efficiency. Furthermore, Python scripts can be run on various platforms, making them highly portable and adaptable to different environments.
In summary, Python scripts serve as powerful tools for executing a wide array of programming tasks. Their simplicity and the rich set of libraries available empower users to create efficient and effective solutions to complex problems. As the demand for automation and data analysis continues to grow, the relevance and utility of Python scripts are likely to expand even further in various industries.
Author Profile
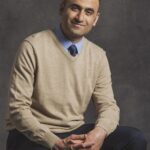
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?