What Is a Python SQLite Cursor and How Does It Work?
In the world of data management and manipulation, Python’s integration with SQLite stands out as a powerful yet accessible solution for developers and data enthusiasts alike. At the heart of this interaction lies the SQLite cursor, a fundamental component that plays a pivotal role in executing SQL commands and retrieving data from databases. Whether you are building a small application or working on a larger project, understanding how to effectively utilize a cursor can significantly enhance your ability to manage and interact with your data.
A Python SQLite cursor serves as an intermediary between your Python code and the SQLite database, allowing you to execute SQL statements and fetch results seamlessly. By creating a cursor object, you gain the ability to perform a wide range of operations, from inserting and updating records to querying data with precision. This flexibility is essential for anyone looking to harness the full potential of SQLite within their Python applications.
Moreover, cursors are designed to handle the complexities of database interactions, providing methods that simplify the process of navigating through result sets. With the ability to iterate over results, fetch specific records, and even manage transactions, the SQLite cursor empowers developers to write efficient and effective database-driven applications. As we delve deeper into the workings of the SQLite cursor, you’ll discover the nuances that make it an indispensable tool in the realm of Python programming.
Understanding the SQLite Cursor in Python
A cursor in SQLite serves as a control structure that enables you to traverse records in a database. It acts as a pointer that allows you to execute SQL commands, retrieve results, and manage the interactions with the database. Cursors are essential for managing database operations efficiently and effectively.
When you create a cursor in Python using the SQLite module, you typically follow a straightforward pattern: establishing a connection to the database, creating a cursor object, and then using that cursor to execute SQL commands.
Creating a Cursor
To create a cursor, you first need to establish a connection to your SQLite database. Here’s a simple example:
python
import sqlite3
# Connect to the SQLite database
connection = sqlite3.connect(‘example.db’)
# Create a cursor object
cursor = connection.cursor()
Once the cursor is created, it can be used to execute various SQL statements.
Using the Cursor to Execute SQL Commands
Cursors allow for the execution of SQL commands through methods like `execute()`, `executemany()`, and `executescript()`. Here are the primary methods you might use:
- execute(): This method is used for executing a single SQL statement. It can also accept parameters for parameterized queries.
- executemany(): This method allows you to execute the same SQL statement multiple times with different parameters.
- executescript(): This method is designed to execute multiple SQL statements in a single call.
For example, to create a table and insert data using the cursor, you can do the following:
python
# Create a table
cursor.execute(‘CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)’)
# Insert a single record
cursor.execute(‘INSERT INTO users (name, age) VALUES (?, ?)’, (‘Alice’, 30))
# Insert multiple records
cursor.executemany(‘INSERT INTO users (name, age) VALUES (?, ?)’, [(‘Bob’, 25), (‘Charlie’, 35)])
Fetching Data
To retrieve data from the database, the cursor provides several fetch methods:
- fetchone(): Returns the next row of a query result, or None if no more rows are available.
- fetchall(): Fetches all (remaining) rows of a query result, returning a list.
- fetchmany(size): Fetches the next set of `size` rows of a query result.
Here’s an example of fetching data:
python
# Execute a query
cursor.execute(‘SELECT * FROM users’)
# Fetch all results
results = cursor.fetchall()
# Display results
for row in results:
print(row)
Closing the Cursor and Connection
It is important to properly close the cursor and the database connection once your operations are complete. This is crucial for resource management and avoiding potential memory leaks. You can close the cursor and connection as follows:
python
# Close the cursor
cursor.close()
# Close the connection
connection.close()
Method | Description |
---|---|
execute() | Executes a single SQL statement. |
executemany() | Executes the same SQL statement multiple times. |
executescript() | Executes multiple SQL statements. |
fetchone() | Fetches the next row from the result set. |
fetchall() | Fetches all remaining rows in the result set. |
fetchmany(size) | Fetches the next `size` rows from the result set. |
Understanding Python SQLite Cursor
A cursor in Python’s SQLite module is an object that facilitates interaction with the database. It acts as a pointer, enabling the execution of SQL commands and the retrieval of results. The cursor is crucial for performing operations like querying, inserting, updating, and deleting records in the database.
Creating a Cursor
To create a cursor in Python with SQLite, you typically follow these steps:
- Establish a connection to the SQLite database.
- Create a cursor object using the connection.
Here is an example:
python
import sqlite3
# Create a connection to the database
connection = sqlite3.connect(‘example.db’)
# Create a cursor object
cursor = connection.cursor()
Executing SQL Commands
Cursors allow for the execution of various SQL commands. Common methods include:
- `execute(sql_query)`: Executes a single SQL statement.
- `executemany(sql_query, seq_of_parameters)`: Executes the same SQL statement multiple times with different parameters.
- `executescript(sql_script)`: Executes multiple SQL statements in a single call.
Example of executing a query:
python
# Creating a table
cursor.execute(”’CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)”’)
# Inserting data
cursor.execute(”’INSERT INTO users (name) VALUES (?)”’, (‘Alice’,))
Fetching Data
Once a query has been executed, the cursor provides methods to fetch the results:
- `fetchone()`: Fetches the next row of a query result set.
- `fetchall()`: Fetches all (remaining) rows of a query result set.
- `fetchmany(size)`: Fetches the next set of `size` rows.
Example of fetching data:
python
# Querying data
cursor.execute(‘SELECT * FROM users’)
# Fetching one record
user = cursor.fetchone()
# Fetching all records
all_users = cursor.fetchall()
Cursor Context Management
Using cursors within a context manager ensures proper resource management. This automatically closes the cursor after the block of code is executed, preventing memory leaks.
Example:
python
with connection:
with connection.cursor() as cursor:
cursor.execute(‘SELECT * FROM users’)
users = cursor.fetchall()
Closing the Cursor
It is essential to close the cursor after its use to release database resources:
python
cursor.close()
Alternatively, if a cursor is created within a `with` statement, it will be automatically closed when the block is exited.
Best Practices
- Always close cursors when done to free resources.
- Use parameterized queries to prevent SQL injection.
- Handle exceptions using try-except blocks to manage errors gracefully.
Following these practices ensures that database interactions remain efficient and secure.
Understanding Python SQLite Cursors: Expert Insights
Dr. Emily Carter (Database Systems Analyst, TechData Solutions). “A Python SQLite cursor is an essential object that allows developers to execute SQL commands and fetch data from a SQLite database. It serves as a pointer, enabling interaction with the database in a structured manner, which is crucial for efficient data manipulation.”
Michael Chen (Senior Software Engineer, DataFlow Innovations). “In Python, the SQLite cursor provides a convenient interface for executing queries and retrieving results. By using methods like `execute()` and `fetchall()`, developers can streamline their database interactions, making it easier to handle large datasets.”
Sarah Thompson (Lead Python Developer, CodeCraft Labs). “Understanding how to utilize a SQLite cursor effectively is vital for any Python developer working with databases. It not only facilitates the execution of SQL statements but also manages the context of the database connection, ensuring data integrity throughout the application.”
Frequently Asked Questions (FAQs)
What is a Python SQLite cursor?
A Python SQLite cursor is an object that allows you to interact with the SQLite database. It facilitates the execution of SQL commands and retrieval of data from the database.
How do you create a cursor in Python using SQLite?
To create a cursor in Python using SQLite, you first need to establish a connection to the database using `sqlite3.connect()`, and then call the `cursor()` method on the connection object.
What are the primary functions of a SQLite cursor?
The primary functions of a SQLite cursor include executing SQL statements, fetching data from the result set, and managing the context of the database operations. It serves as an intermediary between the Python program and the database.
How do you execute SQL commands using a SQLite cursor?
You can execute SQL commands using a SQLite cursor by calling the `execute()` method on the cursor object, passing the SQL command as a string argument. For example, `cursor.execute(“SELECT * FROM table_name”)`.
What methods are available for fetching data with a SQLite cursor?
The main methods for fetching data with a SQLite cursor are `fetchone()`, `fetchall()`, and `fetchmany(size)`. `fetchone()` retrieves the next row, `fetchall()` retrieves all remaining rows, and `fetchmany(size)` retrieves a specified number of rows.
How do you close a SQLite cursor?
To close a SQLite cursor, you should call the `close()` method on the cursor object. This action releases any resources held by the cursor and ensures proper cleanup of the database connection.
A Python SQLite cursor is an essential component of the SQLite database interface in Python, primarily used to execute SQL commands and manage the context of the database operations. It acts as a control structure that enables interaction with the database, allowing users to perform various tasks such as querying data, inserting records, updating entries, and deleting data. The cursor provides methods that facilitate these operations, making it a fundamental tool for database manipulation in Python applications.
One of the key features of the SQLite cursor is its ability to fetch data from the database. It supports several methods, including fetchone(), fetchall(), and fetchmany(), which allow users to retrieve query results in different formats. Additionally, the cursor maintains the state of the database connection and ensures that transactions are managed effectively, providing a layer of abstraction that simplifies database interactions for developers.
Moreover, the use of cursors in Python SQLite promotes efficient resource management. By utilizing context managers, developers can ensure that cursors are properly closed after their operations are completed, preventing potential memory leaks and ensuring that database connections are released. Overall, understanding the functionality and best practices associated with SQLite cursors is crucial for anyone looking to work with databases in Python, as it enhances both performance and code maintainability.
Author Profile
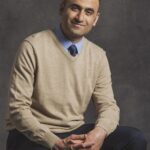
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?