What Is a Static Method in Python and How Does It Work?
In the world of Python programming, understanding the nuances of object-oriented design is crucial for writing clean, efficient, and reusable code. Among the various concepts that developers encounter, static methods stand out as a powerful tool that can enhance the functionality of classes without the need for instantiating objects. Whether you’re a seasoned programmer or just starting your coding journey, grasping the concept of static methods can significantly impact the way you structure your code and manage functionality within your classes.
A static method in Python is a unique feature that allows you to define a method that belongs to a class rather than any particular instance of that class. This means that static methods can be called on the class itself, without the need to create an object. They are particularly useful for utility functions that perform a task in isolation, without relying on class or instance attributes. By using static methods, developers can promote code organization and clarity, making it easier to understand the purpose of the method within the context of the class.
Moreover, static methods contribute to the overall design of a program by providing a way to encapsulate functionality that is logically related to the class but does not require access to its instance-specific data. This can lead to more efficient memory usage and a cleaner interface, as static methods can be invoked directly through the
Understanding Static Methods
Static methods in Python are a type of method that belong to a class rather than an instance of the class. They can be called on the class itself, rather than on an instance of the class. This makes static methods particularly useful for utility functions that do not require access to any instance-specific data.
To define a static method, the `@staticmethod` decorator is used above the method definition. Here’s a simple example:
“`python
class MathOperations:
@staticmethod
def add(a, b):
return a + b
result = MathOperations.add(5, 3) This will return 8
“`
In this example, the `add` method is defined as a static method, allowing it to be called directly on the `MathOperations` class without creating an instance.
Key Characteristics of Static Methods
- No Access to Instance or Class Data: Static methods cannot access or modify the class state or instance state. They do not take `self` or `cls` as their first parameter.
- Utility Functions: They are often used for utility functions that perform a task without needing to know anything about the class or its instances.
- Namespace Organization: Static methods help in organizing functions that are related to the class but do not require any data from it.
Comparison with Other Method Types
To better understand static methods, it can be helpful to compare them with instance methods and class methods.
Method Type | Decorator | Access to Instance Data | Access to Class Data |
---|---|---|---|
Instance Method | None | Yes (self) | Yes (cls) |
Class Method | @classmethod | No | Yes (cls) |
Static Method | @staticmethod | No | No |
When to Use Static Methods
Static methods are ideal in situations where:
- The method’s functionality is independent of the class’s instance or state.
- You want to group related functions within a class for better organization.
- You need a method that might be logically related to the class but does not require access to any class or instance data.
By leveraging static methods, developers can write cleaner, more organized code that clearly delineates the responsibilities of various components within a program.
Definition of a Static Method
A static method in Python is a method that belongs to a class rather than an instance of that class. It does not require access to instance-specific data or methods, and it can be called on the class itself rather than on instances of the class. Static methods are defined using the `@staticmethod` decorator.
Characteristics of Static Methods
- No access to instance (`self`) or class (`cls`): Static methods do not take a reference to the instance or the class as the first parameter.
- Utility functions: They can be used to define utility functions within a class that logically belong to the class but do not modify class or instance state.
- Encapsulation: They can encapsulate functionality that is related to the class, maintaining a clean and organized namespace.
How to Define a Static Method
To define a static method, you use the `@staticmethod` decorator before the method definition. Here is an example:
“`python
class MathOperations:
@staticmethod
def add(a, b):
return a + b
Calling the static method
result = MathOperations.add(5, 3) Returns 8
“`
Use Cases for Static Methods
Static methods are particularly useful in the following scenarios:
- Utility Functions: When a method performs a calculation or a task that does not modify class or instance state.
- Factory Methods: When creating instances of a class in a specific way without needing to instantiate the class first.
- Organizing Code: To group related functionalities logically under a class without requiring object instantiation.
Comparison with Instance and Class Methods
Feature | Static Method | Class Method | Instance Method |
---|---|---|---|
Decorator | `@staticmethod` | `@classmethod` | None |
First Parameter | None | Class (`cls`) | Instance (`self`) |
Access to State | No | Yes (via `cls`) | Yes (via `self`) |
Use Case | Utility functions | Factory methods or class state | Operations on instance state |
Limitations of Static Methods
- No Access to Instance Data: Static methods cannot access or modify instance-specific data.
- Less Flexibility: They may not be as flexible as instance methods for tasks that require knowledge of the class state or instance state.
Best Practices
- Use static methods for operations that do not modify the state of the class or instances.
- Keep the logic within static methods simple and focused on a single responsibility.
- Document the static method clearly to indicate its purpose and how it should be used.
Understanding Static Methods in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Static methods in Python serve as a way to define functions that belong to a class rather than an instance of that class. This allows for utility functions that can be called without creating an object, making the code cleaner and more efficient.
James Liu (Python Developer Advocate, CodeCraft). The beauty of static methods lies in their simplicity. They do not require access to instance variables or methods, which makes them ideal for operations that are logically connected to the class but do not need to manipulate instance state.
Sarah Thompson (Lead Data Scientist, Data Solutions Group). In practice, static methods can enhance code organization by grouping related functions within a class. This encapsulation helps maintain a clean namespace and promotes better code readability and maintainability.
Frequently Asked Questions (FAQs)
What is a static method in Python?
A static method in Python is a method that belongs to a class rather than an instance of the class. It does not require access to instance or class-specific data and is defined using the `@staticmethod` decorator.
How do you define a static method in Python?
To define a static method, use the `@staticmethod` decorator above the method definition within a class. For example:
“`python
class MyClass:
@staticmethod
def my_static_method():
pass
“`
When should you use a static method?
Static methods are useful when you need a method that logically belongs to the class but does not require access to instance or class attributes. They can be used for utility functions related to the class.
Can static methods access class or instance variables?
No, static methods cannot access class or instance variables directly. They do not receive the implicit first argument (`self` or `cls`) that instance and class methods do.
What is the difference between a static method and a class method?
A static method does not take any special first parameter and cannot access class or instance data, while a class method, defined with the `@classmethod` decorator, takes `cls` as its first parameter and can access class-level data.
Are static methods inherited in Python?
Yes, static methods are inherited by subclasses in Python. They can be called on the subclass just like instance methods, but they still behave as static methods without access to instance-specific data.
A static method in Python is a method that belongs to a class rather than an instance of the class. It is defined using the `@staticmethod` decorator and does not require a reference to the instance (`self`) or the class (`cls`) as its first parameter. This characteristic allows static methods to be called on the class itself, rather than needing to create an instance of the class. Static methods are often used for utility functions that perform a task in isolation and do not modify class or instance state.
One of the key advantages of static methods is their ability to encapsulate functionality that is logically related to the class but does not require access to instance-specific data. This can lead to cleaner and more organized code, as it allows developers to group related functions within a class context. Additionally, static methods can enhance performance since they do not involve the overhead of instance creation.
In summary, static methods serve as a powerful feature in Python that promotes code organization and efficiency. They provide a mechanism to define functions that are relevant to a class without requiring access to instance or class-level data. Understanding how and when to use static methods can significantly improve the design and maintainability of Python applications.
Author Profile
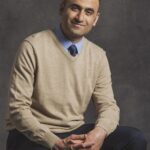
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?