Which Data Types in Python Are Immutable? A Comprehensive Guide
In the dynamic world of programming, understanding data types is fundamental to mastering any language, and Python is no exception. As a versatile and widely-used language, Python offers a variety of data types, each with its own unique characteristics and behaviors. Among these, the concept of immutability stands out as a crucial aspect that every Python developer should grasp. Immutability refers to the inability to change an object after it has been created, which can significantly impact how data is handled and manipulated in your programs.
In Python, certain data types are classified as immutable, meaning that once they are defined, their values cannot be altered. This characteristic not only influences how these data types are stored in memory but also affects performance and efficiency in programming. Understanding which data types are immutable can help developers make informed decisions when designing their code, particularly when it comes to managing state and ensuring data integrity.
As we delve deeper into the topic, we will explore the various immutable data types in Python, their applications, and the implications of using them in different programming scenarios. By the end of this discussion, you’ll have a clearer understanding of how immutability can enhance your coding practices and contribute to more robust and efficient Python applications.
Understanding Immutable Data Types in Python
In Python, an immutable data type is one that cannot be altered after its creation. This characteristic is crucial for maintaining data integrity and ensuring that objects remain unchanged throughout their lifecycle. The following data types are considered immutable in Python:
- Strings: Once a string is created, it cannot be modified. Any modification results in the creation of a new string.
- Tuples: Similar to lists, but tuples cannot be changed after they are created. They are often used to store related pieces of data that shouldn’t change.
- Frozensets: These are immutable versions of sets, which means you cannot add or remove items once the frozenset is created.
- Bytes: A bytes object is a sequence of integers in the range of 0 to 255 and is immutable.
Comparison of Mutable and Immutable Data Types
It is important to understand the differences between mutable and immutable data types in Python. The following table summarizes these differences:
Data Type | Mutable/Immutable | Example |
---|---|---|
List | Mutable | [1, 2, 3] |
Dictionary | Mutable | {‘key’: ‘value’} |
Set | Mutable | {1, 2, 3} |
String | Immutable | ‘hello’ |
Tuple | Immutable | (1, 2, 3) |
Frozenset | Immutable | frozenset([1, 2, 3]) |
Bytes | Immutable | b’hello’ |
Implications of Immutability
The immutability of certain data types has several implications in programming:
- Performance: Immutable objects can be more efficient in terms of memory and speed, as they can be cached and reused.
- Safety: Since immutable objects cannot be altered, they reduce the risk of accidental changes to data, particularly in concurrent programming environments.
- Hashability: Immutable types can be used as keys in dictionaries and as elements in sets, while mutable types cannot.
Understanding the characteristics of immutable data types is essential for effective programming in Python, as it influences data management and application design.
Understanding Immutable Data Types in Python
In Python, immutability refers to the property of an object whose state cannot be modified after it is created. This concept plays a crucial role in ensuring data integrity and is particularly relevant in various programming contexts. The following data types in Python are considered immutable:
- Tuples: A tuple is a collection of ordered elements that can contain different data types. Once defined, the elements of a tuple cannot be changed, added, or removed.
- Strings: Strings are sequences of characters. In Python, once a string is created, it cannot be modified. Any operation that alters a string will result in the creation of a new string.
- Frozensets: A frozenset is an immutable version of a set. It is a collection of unique elements that cannot be changed after creation. You cannot add or remove elements from a frozenset.
- Bytes: Bytes represent immutable sequences of bytes. They are used for handling binary data and cannot be modified after creation.
Comparison of Mutable and Immutable Data Types
To better understand the differences between mutable and immutable data types, the following table highlights key characteristics:
Data Type | Mutability | Examples of Operations |
---|---|---|
List | Mutable | Append, Remove, Sort |
Dictionary | Mutable | Add, Update, Delete |
Tuple | Immutable | No modifications allowed |
String | Immutable | Concatenation results in new string |
Set | Mutable | Add, Remove |
Frozenset | Immutable | No modifications allowed |
Bytes | Immutable | No modifications allowed |
Implications of Using Immutable Data Types
Choosing to use immutable data types can have several implications on performance and program design:
- Thread Safety: Immutable objects are inherently thread-safe, as their state cannot be changed. This reduces the complexity of concurrent programming.
- Hashability: Since the content of immutable types cannot change, they can be used as keys in dictionaries and elements in sets, unlike mutable types.
- Memory Efficiency: In certain scenarios, immutable objects can lead to memory optimization. Python can reuse existing immutable objects instead of creating new ones, especially for small integers and strings.
- Functional Programming: Immutable data structures align well with functional programming paradigms, allowing for easier reasoning about code and reducing side effects.
Understanding these characteristics and implications is essential for effective Python programming, as it influences data structure selection and overall application design.
Understanding Immutable Data Types in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, immutable data types include integers, floats, strings, and tuples. These types cannot be altered after their creation, which is crucial for maintaining data integrity and optimizing performance in applications.
Michael Chen (Data Scientist, AI Analytics Group). The concept of immutability in Python is fundamental for functional programming. When working with immutable types, developers can avoid unintended side effects, ensuring that functions behave predictably and data remains consistent throughout the program.
Sarah Thompson (Python Developer, CodeCraft Solutions). Understanding which data types are immutable is essential for effective memory management in Python. Using immutable types like frozensets and tuples can lead to more efficient code, especially in scenarios involving concurrent programming.
Frequently Asked Questions (FAQs)
Which of the following data types is immutable in Python?
Strings, tuples, and frozensets are examples of immutable data types in Python. Once created, their values cannot be changed.
What does it mean for a data type to be immutable?
An immutable data type cannot be modified after its creation. Any operation that attempts to change the value results in the creation of a new object instead.
Can you give examples of mutable data types in Python?
Lists, dictionaries, and sets are mutable data types in Python. They allow modification of their contents without creating a new object.
How do immutable data types affect performance in Python?
Immutable data types can enhance performance by enabling optimizations such as caching and memory reuse, as their state does not change.
Are there any advantages to using immutable data types?
Yes, immutable data types provide benefits such as thread safety, easier debugging, and predictable behavior, as their values remain constant throughout their lifetime.
How can I convert a mutable data type to an immutable one in Python?
You can convert a list to a tuple using the `tuple()` function, or a set to a frozenset using the `frozenset()` function, both of which create immutable versions of the original mutable types.
In Python, immutability refers to the property of an object that prevents it from being modified after its creation. Among the various data types available in Python, some are mutable, meaning they can be altered, while others are immutable, meaning their state cannot be changed. The primary immutable data types in Python include tuples, strings, and frozensets. Understanding these distinctions is crucial for effective programming and memory management.
Strings are one of the most commonly used immutable data types. Once a string is created, any operation that seems to modify it actually creates a new string object. This characteristic is important for ensuring data integrity and avoiding unintended side effects in programs. Tuples, similar to lists, can hold a collection of items, but unlike lists, they cannot be altered after their creation. This makes tuples suitable for fixed collections of items where the integrity of the data must be maintained.
Frozensets are another example of an immutable data type. They are similar to sets but do not allow modifications such as adding or removing elements. This immutability makes frozensets particularly useful in situations where a constant set of values is required, such as using them as keys in dictionaries. Recognizing the properties of these immutable data types allows
Author Profile
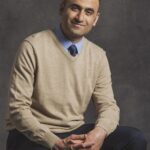
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?