Which of the Following Represents a Signal in Linux? A Comprehensive Guide
In the intricate world of Linux, signals serve as vital messengers, facilitating communication between processes and the operating system. These signals are essential for managing the behavior of applications, allowing them to respond to events, terminate gracefully, or even pause their operations. For anyone venturing into the realm of Linux system programming or administration, understanding which signals exist and how they function is crucial. This article will delve into the various types of signals available in Linux, shedding light on their significance and practical applications.
Signals in Linux can be thought of as notifications sent to a process, informing it of events that require its attention. Each signal has a specific purpose, whether it’s to indicate that a user has requested the process to terminate, that a child process has finished executing, or that an error has occurred. The ability to handle these signals effectively can greatly enhance the robustness and responsiveness of applications running on Linux.
As we explore the landscape of signals, we will uncover the different categories they fall into, including standard signals, real-time signals, and the ways in which processes can choose to respond to them. By grasping the nuances of signal handling, developers and system administrators can harness the full potential of Linux, ensuring that their applications not only function correctly but also interact seamlessly with the operating system and other processes
Understanding Signals in Linux
In Linux, signals are a form of inter-process communication that can be used to notify a process that a specific event has occurred. They can be sent by the operating system or other processes. Each signal is identified by a unique number and can be handled by user-defined functions or default actions.
Common Signals
There are several common signals in Linux, each serving different purposes. Here are some of the most frequently used signals:
- SIGINT (2): Sent when a user interrupts a process, typically by pressing Ctrl+C.
- SIGTERM (15): A termination signal that can be sent to request a process to terminate gracefully.
- SIGKILL (9): Forces a process to terminate immediately without cleanup.
- SIGSTOP (19): Stops a process from executing, but it can be resumed later.
- SIGHUP (1): Indicates that a terminal has been disconnected, often used to reload configuration files.
Below is a table summarizing some key signals:
Signal | Number | Description |
---|---|---|
SIGINT | 2 | User interrupt (Ctrl+C) |
SIGTERM | 15 | Termination request |
SIGKILL | 9 | Forceful termination |
SIGSTOP | 19 | Stop the process |
SIGHUP | 1 | Terminal disconnect |
Sending Signals
Signals can be sent using the `kill` command in the terminal. Despite its name, the `kill` command can be used to send any signal, not just termination signals. The basic syntax is:
“`
kill -SIGNAL PID
“`
Where `SIGNAL` is the name or number of the signal you want to send, and `PID` is the Process ID of the target process. For example, to send a SIGTERM to a process with PID 1234, you would use:
“`
kill -15 1234
“`
Alternatively, you can use the `killall` command to send a signal to all processes with a specific name:
“`
killall -SIGNAL process_name
“`
Handling Signals in Programs
Processes can choose how to respond to signals by defining signal handlers. This can be done using the `signal()` or `sigaction()` functions in C/C++. Here is a brief overview of how to set up a signal handler:
- Include required headers:
“`c
include
include
include
“`
- Define the signal handler function:
“`c
void handle_sigint(int sig) {
printf(“Caught SIGINT!\n”);
exit(0);
}
“`
- Register the signal handler:
“`c
signal(SIGINT, handle_sigint);
“`
With this setup, when a SIGINT signal is received, the custom handler will execute instead of the default action.
Conclusion on Signals
Understanding how signals work in Linux is crucial for managing processes effectively. Proper handling of signals allows for better control over program execution and can enhance the robustness of applications by ensuring they respond appropriately to unexpected events.
Understanding Signals in Linux
In Linux, signals are a form of inter-process communication used to notify processes of events. They can be generated by the kernel, user commands, or other processes. Each signal has a specific purpose and can be handled or ignored by processes.
Common Signals in Linux
Signals can be categorized into several types, each serving different functions. Below are some common signals and their representations:
Signal Name | Signal Number | Description |
---|---|---|
SIGINT | 2 | Interrupt from keyboard (Ctrl+C) |
SIGTERM | 15 | Termination signal |
SIGKILL | 9 | Immediate termination of a process |
SIGSTOP | 19 | Stop a process (cannot be caught or ignored) |
SIGHUP | 1 | Hangup detected on controlling terminal |
SIGQUIT | 3 | Quit signal (produces core dump) |
SIGUSR1 | 10 | User-defined signal 1 |
SIGUSR2 | 12 | User-defined signal 2 |
Signal Generation and Handling
Processes can generate signals using various system calls. The most commonly used calls for sending signals include:
- kill: Primarily used to send signals to processes, not just for termination.
- raise: Sends a signal to the calling process itself.
- sigqueue: Sends a signal along with additional data to a specified process.
Processes can handle signals using signal handlers. A signal handler is a function defined by the programmer that executes when a specific signal is received.
Signal Handling Mechanisms
The following mechanisms can be employed for handling signals:
- Default Action: Each signal has a default action which could be to terminate the process, ignore the signal, or stop the process.
- Custom Handlers: Developers can define custom signal handlers to execute specific code in response to a signal.
- Ignoring Signals: A process can choose to ignore certain signals, although some signals, like SIGKILL and SIGSTOP, cannot be ignored.
Signal Safety
When writing signal handlers, it’s crucial to ensure that the code is reentrant and safe. Only certain functions are considered safe to call within a signal handler:
- `signal()`
- `sigaction()`
- `write()`
- `exit()`
- `fork()`
Avoid using non-reentrant functions like `malloc()`, `printf()`, or any function that modifies global state.
Conclusion of Signal Representation
In summary, signals are integral to process management in Linux, allowing for effective communication and control. Understanding the various types of signals, their handling mechanisms, and the safety considerations is essential for developing robust applications that interact with the Linux operating system.
Understanding Signals in Linux: Expert Insights
Dr. Emily Carter (Senior Linux Systems Engineer, Tech Innovations Inc.). “In the Linux operating system, signals are a crucial mechanism for inter-process communication, allowing processes to notify each other about events such as termination or the need for attention.”
Michael Chen (Lead Software Developer, Open Source Solutions). “Signals in Linux are represented by specific integers, such as SIGINT for interrupt signals or SIGKILL for termination. Understanding these signals is essential for effective process management and debugging.”
Sarah Thompson (Linux Kernel Developer, Advanced Computing Labs). “Each signal in Linux has a defined purpose and behavior. For instance, the SIGTERM signal is used to gracefully terminate a process, while SIGSEGV indicates a segmentation fault, highlighting the importance of signals in error handling.”
Frequently Asked Questions (FAQs)
Which of the following represents a signal in Linux?
In Linux, signals are represented by integer values, typically defined in the `
How are signals generated in Linux?
Signals can be generated by the kernel in response to events such as hardware interrupts, or by processes using system calls like `kill()`, `raise()`, or `abort()`.
What is the purpose of signals in Linux?
Signals serve as a mechanism for notifying processes of events that require immediate attention, such as termination requests or the occurrence of specific conditions.
Can a process ignore signals in Linux?
Yes, a process can choose to ignore certain signals by using the `signal()` or `sigaction()` system calls to set the signal handler to `SIG_IGN`.
What happens when a signal is received by a process?
When a signal is received, the process can either handle it with a custom signal handler, ignore it, or allow the default action to occur, which may include termination or stopping the process.
Are there different types of signals in Linux?
Yes, there are two main types of signals: standard signals, which are predefined and have specific meanings, and real-time signals, which are user-defined and can be queued for delivery.
In Linux, a signal is a limited form of inter-process communication used to notify a process that a specific event has occurred. Signals can be generated by the operating system, by user actions, or by other processes. Each signal is represented by a unique integer value and can be sent to processes to inform them of events such as termination requests, interruptions, or specific conditions like segmentation faults. Common signals include SIGINT, SIGTERM, and SIGKILL, each serving distinct purposes in process management.
Understanding signals is essential for effective process control in Linux. Signals allow processes to handle asynchronous events, enabling them to respond appropriately without the need for constant polling. This mechanism is crucial for maintaining system stability and ensuring that applications can gracefully handle interruptions or termination requests. Furthermore, developers can customize how their applications respond to specific signals, enhancing the robustness and reliability of their software.
Key takeaways from the discussion on signals in Linux include the importance of signal handling in process communication and the ability of developers to define custom behaviors for their applications in response to various signals. This capability not only improves user experience by allowing applications to manage resources efficiently but also contributes to overall system performance and reliability. A comprehensive understanding of signals is vital for anyone looking to work effectively
Author Profile
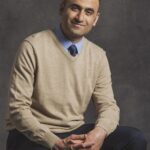
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?