How Can You Create a VB Script to Open an Access Database?
In the world of automation and data management, Visual Basic (VB) scripting stands out as a powerful tool for streamlining tasks and enhancing productivity. One common requirement for many professionals is the ability to interact with Microsoft Access databases programmatically. Whether you’re a developer looking to automate data entry, a business analyst needing to generate reports, or simply someone wanting to simplify routine database operations, creating a VB script that opens an Access database can be a game changer. In this article, we will explore the essentials of crafting such a script, empowering you to harness the full potential of your data with ease.
Opening an Access database using VB scripting is not just about executing a few lines of code; it involves understanding the underlying principles of database connectivity and how to manipulate data effectively. By leveraging the capabilities of VB, users can create scripts that automate repetitive tasks, ensuring efficiency and accuracy. This process can significantly reduce the time spent on manual data handling, allowing you to focus on more strategic activities.
As we delve deeper into this topic, we will cover the necessary components required to establish a connection to an Access database, including the relevant libraries and references. We will also highlight best practices for error handling and performance optimization, ensuring that your scripts run smoothly and reliably. Whether you’re a seasoned
Creating a VB Script to Open an Access Database
To create a VBScript that opens an Access database, you will need to utilize the `Access.Application` object. This object allows you to control Microsoft Access via automation. Below is a detailed walkthrough of how to set up the script and the necessary components involved.
Basic Structure of the VB Script
The basic structure of your VBScript will include the creation of an instance of Access, opening the database, and optionally displaying it to the user. Below is an example of how the script can be structured:
“`vbscript
Dim accessApp
Set accessApp = CreateObject(“Access.Application”)
‘ Path to the Access database
Dim dbPath
dbPath = “C:\path\to\your\database.accdb”
‘ Open the database
accessApp.OpenCurrentDatabase dbPath
‘ Optionally make the Access application visible
accessApp.Visible = True
‘ Cleanup
Set accessApp = Nothing
“`
In this script:
- `CreateObject(“Access.Application”)` initializes a new instance of Microsoft Access.
- `OpenCurrentDatabase` method opens the specified Access database.
- Setting `accessApp.Visible` to `True` allows the user to see the Access interface.
Essential Components
To effectively execute the script, consider the following components:
- Path to Database: Ensure the path to your Access database is correct. Use double backslashes (`\\`) or a single forward slash (`/`) to avoid path errors.
- Error Handling: Implement error handling to manage scenarios where the database may not open correctly.
Here’s an example of how to incorporate error handling:
“`vbscript
On Error Resume Next
accessApp.OpenCurrentDatabase dbPath
If Err.Number <> 0 Then
WScript.Echo “Error opening database: ” & Err.Description
Err.Clear
End If
On Error GoTo 0
“`
Running the Script
To run the script, save it with a `.vbs` extension, such as `OpenAccessDatabase.vbs`. You can execute it by double-clicking the file or running it via the command prompt.
Common Issues and Troubleshooting
When working with VBScript and Access, you may encounter common issues. Here are some troubleshooting tips:
Issue | Solution |
---|---|
Access not installed | Ensure Microsoft Access is installed on your machine. |
Incorrect database path | Verify the path to the database is correct. |
Permissions issue | Ensure you have the necessary permissions to access the database file. |
Access version compatibility | Ensure that the version of Access is compatible with the script. |
Utilizing VBScript to automate the opening of an Access database can streamline processes and enhance productivity. By following the outlined steps, you can effectively set up your script and handle potential issues that may arise.
Understanding VBScript and Access Database Integration
VBScript is a scripting language developed by Microsoft, primarily used for automation and web development tasks. Integrating VBScript with Microsoft Access can streamline the process of opening and managing databases programmatically.
Prerequisites
Before creating a VBScript to open an Access database, ensure the following:
- Microsoft Access is installed on your machine.
- You have the necessary permissions to access the database file.
- Familiarity with the file path and database structure.
Creating the VBScript
To create a VBScript that opens an Access database, follow these steps:
- Open a Text Editor: Use Notepad or any text editor of your choice.
- Write the VBScript Code: Below is a sample script that you can modify according to your database file path.
“`vbscript
Dim accessApp
Dim dbPath
‘ Specify the path to your Access database
dbPath = “C:\Path\To\Your\Database.accdb”
‘ Create an instance of Access application
Set accessApp = CreateObject(“Access.Application”)
‘ Open the database
accessApp.OpenCurrentDatabase dbPath
‘ Optionally, make Access visible
accessApp.Visible = True
‘ Clean up
Set accessApp = Nothing
“`
Explanation of the Code
- Dim accessApp: Declares a variable to hold the Access application object.
- dbPath: Specifies the path to the Access database file. Ensure the path is correct.
- CreateObject: This function creates a new instance of the Access application.
- OpenCurrentDatabase: This method opens the specified database.
- Visible: Setting this property to `True` makes the Access application window visible to the user.
- Set accessApp = Nothing: Cleans up the variable, freeing resources.
Running the VBScript
To execute the script, follow these steps:
- Save the Script: Save your file with a `.vbs` extension, for example, `OpenAccessDB.vbs`.
- Run the Script: Double-click the saved file to execute the script. The Access application should open with the specified database.
Troubleshooting Tips
If the script does not work as expected, consider the following:
- Check File Path: Ensure the database path is correct and accessible.
- Access Permissions: Verify that you have permission to open the database.
- VBScript Settings: Ensure that your system allows running VBScript.
Additional Considerations
- Error Handling: Incorporate error handling in your script to manage exceptions effectively.
- Database Locking: Be aware of database locking issues if multiple users are accessing the same database simultaneously.
- Compatibility: Ensure that the VBScript is compatible with your version of Access.
Extending Functionality
You can extend the script’s functionality by adding features such as:
- Opening specific forms or reports within the database.
- Automating data entry or retrieval tasks using SQL queries.
- Creating user prompts for dynamic database file selection.
Implementing these extensions can enhance the usability and effectiveness of your Access database automation.
Expert Insights on Creating VB Scripts for Access Databases
Dr. Emily Carter (Database Systems Analyst, Tech Innovations Corp.). “Creating a VB script to open an Access database is a straightforward process that can significantly enhance automation in data handling. It is essential to ensure that the correct references are set in the VB environment to avoid runtime errors.”
Michael Thompson (Senior Software Developer, CodeCrafters Inc.). “When writing a VB script for Access, it is crucial to handle exceptions effectively. This ensures that the script can gracefully manage scenarios where the database may be inaccessible or corrupted, thereby maintaining data integrity.”
Linda Garcia (IT Consultant, Database Solutions Group). “Utilizing VB scripts to open Access databases can streamline workflows, especially in environments that require frequent data manipulation. It is advisable to include comments in the script for better maintainability and understanding by future developers.”
Frequently Asked Questions (FAQs)
How can I create a VB script to open an Access database?
To create a VB script that opens an Access database, use the following code snippet:
“`vbscript
Dim accessApp
Set accessApp = CreateObject(“Access.Application”)
accessApp.OpenCurrentDatabase “C:\path\to\your\database.accdb”
accessApp.Visible = True
“`
This script initializes an Access application object, opens the specified database, and makes it visible.
What file extension should I use for my Access database?
The file extension for Access databases is typically `.accdb` for newer versions (2007 and later) and `.mdb` for older versions (2003 and earlier). Ensure you use the correct extension based on your database version.
Do I need to have Microsoft Access installed to run the VB script?
Yes, Microsoft Access must be installed on your machine for the VB script to execute successfully, as it relies on the Access application object.
Can I run the VB script from any location on my computer?
You can run the VB script from any location, but ensure that the path to the Access database is correctly specified in the script. If the path is incorrect, the script will fail to open the database.
Is it possible to automate tasks within the Access database using VB script?
Yes, you can automate various tasks within the Access database using VB script. This includes executing queries, generating reports, and manipulating data through the Access application object.
What should I do if the script fails to open the Access database?
If the script fails, check for common issues such as incorrect file paths, missing Access installation, or database file permissions. Ensure that the database is not already open in another instance of Access.
In summary, creating a VBScript to open an Access database is a straightforward process that leverages the capabilities of both VBScript and Microsoft Access. The script typically involves using the ADO (ActiveX Data Objects) library to establish a connection to the database file. By specifying the correct connection string and utilizing the appropriate methods, users can successfully open and interact with their Access databases programmatically.
One of the key takeaways from this discussion is the importance of understanding the connection string format. The connection string must accurately reflect the path to the Access database file and specify the necessary provider. Additionally, error handling should be implemented to manage any potential issues that may arise during the connection process, ensuring that the script runs smoothly and effectively.
Furthermore, users should be aware of the security implications when working with database connections. Proper permissions and security measures must be in place to protect sensitive data. By following best practices in coding and security, users can create robust VBScript solutions that enhance their database management capabilities.
Author Profile
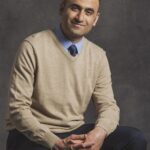
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?