How to Resolve Java Net BindException: Address Already in Use?
If you’ve ever ventured into the world of Java programming, chances are you’ve encountered the notorious `BindException: Address already in use` error. This frustrating message can halt your application in its tracks, leaving you puzzled and searching for answers. Whether you’re a seasoned developer or a novice just embarking on your coding journey, understanding this error is crucial for maintaining smooth operations in your applications. In this article, we will unravel the intricacies of the `BindException`, explore its common causes, and provide you with practical solutions to overcome this hurdle.
Overview
At its core, the `BindException: Address already in use` error arises when a Java application attempts to bind a socket to a port that is already occupied by another process. This situation can occur in various scenarios, such as when a server application is restarted without properly releasing the port or when multiple instances of the same application are inadvertently launched. Understanding the underlying mechanics of network sockets and port management is essential for developers looking to troubleshoot and prevent this issue.
Moreover, the implications of this error extend beyond mere annoyance; they can disrupt service availability and lead to significant downtime. By delving into the reasons behind the `BindException`, you can equip yourself with the knowledge to effectively manage your application’s network resources, ensuring that
Understanding the BindException
A `BindException` in Java occurs when an application attempts to bind a socket to a port that is already in use. This is a common issue when developing network applications, as it can prevent the application from launching or operating correctly.
When a server socket is created and attempts to bind to a specific port, the operating system checks if that port is already occupied by another process. If it is, the `BindException` is thrown, indicating that the address is unavailable.
Common Causes of BindException
There are several reasons why a `BindException` with the message “address already in use” might occur:
- Multiple Instances: Running multiple instances of the same application on the same machine without modifying the port configuration.
- Stale Processes: An application may not have released the port after termination, leaving the port in a TIME_WAIT state.
- Firewall or Security Software: Security software may bind to ports for monitoring, which can interfere with the application.
- Incorrect Configuration: Specifying the wrong port number in the application configuration.
Diagnosing the Issue
To diagnose a `BindException`, you can follow these steps:
- Identify the Port: Determine which port your application is trying to bind to.
- Check Active Connections: Use command-line tools to check if the port is already in use.
You can use the following commands based on your operating system:
- Windows:
“`bash
netstat -aon | findstr :
- Linux/Mac:
“`bash
lsof -i :
These commands will provide you with the process ID (PID) of any active connections using that port.
Resolving BindException
Once you identify the source of the `BindException`, you can resolve it using one of the following methods:
- Change the Port: Modify your application to use a different port that is free.
- Terminate Conflicting Process: If another application is using the port, terminate it or reconfigure it to use a different port.
- Allow TIME_WAIT to Expire: Wait for the TIME_WAIT state to clear if the port was recently used.
- Check Firewall Settings: Ensure that no firewall rules are preventing the application from binding to the desired port.
Example Configuration Table
Here’s an example of how to configure different applications to use different ports:
Application | Port Number |
---|---|
App A | 8080 |
App B | 8081 |
App C | 8082 |
Using unique port numbers for different applications prevents conflicts and allows for smoother operation without encountering `BindException` errors.
Understanding BindException in Java
A `BindException` in Java typically occurs when an application attempts to bind a socket to a specific address and port that is already in use. This exception is a subclass of `SocketException` and is crucial for network programming, especially when dealing with server applications.
Common Causes of BindException
Several factors can lead to a `BindException`, including:
- Port Already in Use: Another application may be using the same port.
- Improper Socket Closure: If a socket is not closed properly, it may still hold onto the port.
- Firewall or Security Software: Sometimes, security settings can block ports from being reused.
- Insufficient Permissions: Running an application without proper permissions can lead to binding issues.
How to Diagnose the Issue
To effectively diagnose and resolve a `BindException`, follow these steps:
- Check for Active Connections: Use command-line tools to see if the port is already in use.
- On Windows:
“`bash
netstat -ano | findstr :
- On Unix/Linux:
“`bash
netstat -tuln | grep :
- Identify the Process: If a port is in use, identify which process is using it.
- On Windows, use:
“`bash
tasklist /FI “PID eq
“`
- On Unix/Linux, you can use:
“`bash
ps -p
- Check Application Code: Ensure sockets are closed properly in your application code. Use try-with-resources or finally blocks to close the sockets.
Best Practices to Avoid BindException
Implementing best practices can help prevent `BindException` occurrences:
- Use Dynamic Ports: If applicable, let the operating system choose an available port.
- Implement Retry Logic: If a `BindException` occurs, consider implementing a retry mechanism with exponential backoff.
- Graceful Shutdown: Ensure your application handles shutdowns gracefully, closing all sockets properly.
- Port Management: Maintain a clear documentation of which ports are being used by your applications.
Example Code Handling BindException
The following Java code snippet demonstrates how to handle a `BindException` effectively:
“`java
import java.net.ServerSocket;
import java.net.BindException;
public class Server {
public static void main(String[] args) {
int port = 8080;
while (true) {
try (ServerSocket serverSocket = new ServerSocket(port)) {
System.out.println(“Server started on port: ” + port);
// Accept connections…
break; // Exit loop upon successful binding
} catch (BindException e) {
System.err.println(“Port ” + port + ” is already in use. Trying another port…”);
port++; // Increment port number and retry
} catch (Exception e) {
e.printStackTrace();
break; // Exit loop on other exceptions
}
}
}
}
“`
This example demonstrates a simple way to retry binding on an incremented port if a `BindException` is caught. Adjustments can be made based on your application’s needs.
While a `BindException` can be a common hurdle in network programming, understanding its causes and implementing effective strategies can help mitigate these issues. Proper diagnosis and coding practices are essential for maintaining robust network applications.
Understanding Java Net BindException: Address Already in Use
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘java.net.BindException: Address already in use’ error typically indicates that your application is attempting to bind to a port that is already occupied by another process. This can occur when multiple instances of an application are running or when a previous instance has not released the port properly.”
Michael Chen (Network Architect, Cloud Solutions Group). “To resolve the BindException, it is crucial to identify the process currently using the port. Tools like ‘netstat’ or ‘lsof’ can help you find the culprit. Once identified, you can either terminate that process or configure your application to use a different port.”
Sarah Thompson (Java Development Consultant, CodeCraft Experts). “In some cases, this error may arise from improper shutdown of applications. Implementing a graceful shutdown mechanism in your Java application can prevent ports from being left in a TIME_WAIT state, which can lead to this BindException.”
Frequently Asked Questions (FAQs)
What does `java.net.BindException: Address already in use` mean?
This exception indicates that a network application is attempting to bind to a port that is already in use by another process, preventing it from establishing a listening socket.
How can I identify which process is using the port?
You can use command-line tools such as `netstat` or `lsof` on Unix-based systems, or `netstat -ano` on Windows, to identify the process occupying the port. This will allow you to terminate or reconfigure the conflicting application.
What steps can I take to resolve this error?
To resolve this error, you can either stop the process using the port, change your application to use a different port, or configure the conflicting application to release the port.
Is it possible to bind to a port that is in a TIME_WAIT state?
No, binding to a port in the TIME_WAIT state is not allowed. You can either wait for the state to clear or use the `SO_REUSEADDR` socket option to allow reuse of the address.
Can firewall settings cause `java.net.BindException`?
While firewall settings typically do not cause this specific exception, they can block incoming connections to your application. Ensure that the firewall allows traffic on the port your application is trying to bind.
What is the best practice for handling `BindException` in Java applications?
Best practices include implementing error handling to catch `BindException`, logging the error for troubleshooting, and providing fallback mechanisms, such as trying an alternate port or notifying the user.
The `java.net.BindException: Address already in use` error typically occurs when a Java application attempts to bind a socket to a port that is already occupied by another process. This situation can arise in various scenarios, such as when a server application is restarted without properly releasing the port, or when multiple instances of the same application are inadvertently launched. Understanding the underlying causes of this exception is crucial for developers to effectively troubleshoot and resolve the issue.
To address this error, developers should first verify which process is currently using the desired port. Tools such as `netstat`, `lsof`, or platform-specific utilities can help identify the conflicting process. Once identified, the developer can either terminate the conflicting process or configure the application to use a different port. Additionally, ensuring that proper cleanup routines are in place when the application shuts down can prevent this issue from recurring.
It is also beneficial for developers to implement error handling in their applications to gracefully manage binding exceptions. This could include retry mechanisms with exponential backoff or user notifications that inform them of the issue. By proactively addressing the potential for `BindException`, developers can enhance the robustness and reliability of their networked applications.
Author Profile
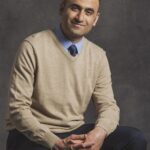
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?