How Can You Effectively Compile a Python Program?
In the ever-evolving landscape of programming, Python stands out as one of the most accessible and versatile languages. Whether you’re a budding developer or a seasoned programmer, the ability to compile your Python programs can significantly enhance your workflow and optimize performance. But what does it mean to compile a Python program, and why is it an essential skill to master? In this article, we will unravel the intricacies of compiling Python code, exploring its benefits, the various methods available, and how it can elevate your coding experience.
Compiling a Python program involves transforming your high-level code into a format that a computer can execute more efficiently. This process not only improves the speed of your applications but also allows you to distribute your software in a more manageable way. While Python is traditionally an interpreted language, understanding the compilation process opens up new avenues for performance optimization and deployment strategies.
As we delve deeper into this topic, we will explore the different compilation techniques available, from using built-in tools to leveraging third-party libraries. By the end of this article, you’ll have a clearer understanding of how to compile your Python programs effectively, empowering you to take your coding skills to the next level. Whether you’re looking to enhance your application’s performance or simply want to learn a new skill,
Understanding Python Compilation
Compiling a Python program involves translating the Python code (source code) into bytecode, which is a lower-level, platform-independent representation of your code. This bytecode can then be executed by the Python interpreter. Unlike languages such as C or C++, which compile directly to machine code, Python uses an intermediate step of bytecode compilation.
Tools for Compilation
There are several tools available that can aid in compiling Python programs:
- CPython: This is the standard Python interpreter that compiles Python code to bytecode automatically.
- PyInstaller: A popular tool that packages Python applications into standalone executables, making distribution easier.
- cx_Freeze: Another tool that creates executables from Python scripts, supporting various platforms.
- Nuitka: A Python-to-C++ compiler that can convert Python code into C code, which is then compiled to machine code.
Steps to Compile a Python Program
To compile a Python program, follow these steps:
- Write your Python script: Ensure your script is free of syntax errors.
- Use the Python interpreter: By default, running the script with the Python interpreter will compile it to bytecode. For example:
“`
python your_script.py
“`
- Locate the compiled bytecode: After running your script, check the `__pycache__` directory within your project folder. The compiled files will have a `.pyc` extension.
Compiling with PyInstaller
To create an executable using PyInstaller, you can follow this simple command:
“`
pyinstaller –onefile your_script.py
“`
This command generates a single executable file. The output can be found in the `dist` directory created by PyInstaller.
Comparison of Compilation Tools
When choosing a compilation tool, consider the following features:
Tool | Output Type | Platform Compatibility | Ease of Use |
---|---|---|---|
CPython | Bytecode (.pyc) | Cross-platform | Automatic |
PyInstaller | Executable | Windows, macOS, Linux | Moderate |
cx_Freeze | Executable | Windows, macOS, Linux | Moderate |
Nuitka | Compiled C code | Cross-platform | Advanced |
Choosing the right tool will depend on your specific needs, including the target environment and the complexity of your Python application.
Understanding Python Compilation
Python is an interpreted language, meaning that its code is executed line by line by the Python interpreter. However, there are scenarios where you may want to compile a Python program into bytecode or an executable for various reasons, such as performance enhancement or distribution.
Compiling Python to Bytecode
When Python code is executed, it is first compiled into bytecode, a lower-level representation of the code that the Python virtual machine can execute. This process is automatic; however, you can manually compile Python files using the `py_compile` module.
- To compile a Python file to bytecode:
“`bash
python -m py_compile your_script.py
“`
- This will generate a `.pyc` file located in the `__pycache__` directory.
Creating Executable Files
If you need to create a standalone executable from a Python script, several tools can facilitate this process:
- PyInstaller
- cx_Freeze
- py2exe (Windows only)
Each tool has its own strengths and weaknesses, but PyInstaller is widely used due to its versatility across platforms.
Using PyInstaller
To compile a Python program into an executable using PyInstaller, follow these steps:
- Install PyInstaller:
“`bash
pip install pyinstaller
“`
- Compile your script:
“`bash
pyinstaller –onefile your_script.py
“`
- The `–onefile` flag bundles everything into a single executable.
- Locate the executable:
- The compiled executable will be found in the `dist` directory.
Configuration Options for PyInstaller
PyInstaller offers several options to customize the build process:
Option | Description |
---|---|
`–noconsole` | Prevents a console window from appearing (useful for GUI applications). |
`–icon=icon.ico` | Specifies an icon for the executable. |
`–add-data` | Includes additional files or directories. |
`–hidden-import` | Includes hidden imports that may not be detected automatically. |
Testing the Compiled Executable
After compilation, it is crucial to test the executable to ensure it works as expected. Execute the compiled file directly from the command line or double-click it in the file explorer.
- Check for any missing dependencies or errors that may arise during execution.
- Use logging or output redirection to capture runtime information for debugging.
Conclusion on Compilation Practices
While the Python ecosystem is primarily designed for ease of use through interpreted execution, compiling scripts into bytecode or executables can provide significant benefits. It is advisable to carefully choose the compilation method that best suits your project requirements and to rigorously test the final product for any issues.
Expert Insights on Compiling Python Programs
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Compiling a Python program typically involves converting your Python code into bytecode, which the Python interpreter can execute. This is often done automatically when you run a Python script. However, for applications requiring distribution, tools like PyInstaller or cx_Freeze can be utilized to create standalone executables.”
James Patel (Lead Developer, CodeCraft Solutions). “While Python is an interpreted language, compiling can enhance performance in certain scenarios. Utilizing tools like Cython allows developers to compile Python code into C, which can significantly speed up execution times for computationally intensive tasks.”
Linda Martinez (Python Educator, LearnPython.org). “For beginners, understanding the distinction between running and compiling Python code is crucial. While ‘compiling’ in Python is less about generating a binary and more about creating bytecode, using an IDE like PyCharm can simplify the process by managing these steps seamlessly.”
Frequently Asked Questions (FAQs)
How do I compile a Python program into an executable?
You can compile a Python program into an executable using tools like PyInstaller, cx_Freeze, or py2exe. These tools package your Python code and its dependencies into a standalone executable file.
What is the difference between compiling and interpreting Python code?
Compiling converts Python code into bytecode, which can be executed by the Python interpreter. Interpreting, on the other hand, involves executing the code line by line without converting it into a separate bytecode file.
Can I compile Python code for different operating systems?
Yes, you can compile Python code for different operating systems by using cross-compilation tools. However, it is often easier to compile on the target operating system to avoid compatibility issues.
Is it necessary to compile Python code for performance improvements?
Compiling Python code is not strictly necessary for performance improvements, as Python is primarily an interpreted language. However, compiling can help reduce startup time and improve execution speed in some cases.
What are the common errors encountered while compiling Python programs?
Common errors include missing dependencies, syntax errors, and issues with incompatible libraries. Ensuring that all required packages are installed and that the code is error-free can help mitigate these issues.
How can I run a compiled Python program?
You can run a compiled Python program by executing the generated executable file in the command line or by double-clicking it, depending on the operating system and how the executable was created.
Compiling a Python program involves transforming the source code written in Python into a format that can be executed by a computer. While Python is primarily an interpreted language, there are methods available to compile Python code into bytecode or executable formats. This process can enhance performance, protect source code, and facilitate distribution. Tools such as PyInstaller, cx_Freeze, and py_compile are commonly used to achieve this, allowing developers to create standalone executables or packaged applications.
One of the key takeaways is that compiling a Python program can significantly improve its portability and execution speed. By converting the code into bytecode, the program can run faster since the interpreter does not need to parse the source code each time. Additionally, compiling can help protect intellectual property by obscuring the source code, making it more challenging for unauthorized users to access or modify it.
Furthermore, it is essential to understand the differences between compiling to bytecode and creating standalone executables. While bytecode compilation is a straightforward process that retains the Python environment, creating an executable often involves bundling the Python interpreter and any necessary libraries. This ensures that the application can run independently on systems without a pre-installed Python environment.
compiling a Python program is
Author Profile
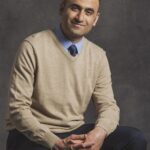
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?