How Can You Call a Function Inside Another Function in Python?
In the world of programming, functions are the building blocks that allow us to structure our code in a logical and efficient manner. Python, a language celebrated for its simplicity and readability, empowers developers to create reusable pieces of code through functions. However, the true power of functions is unlocked when we learn how to call one function from within another. This technique not only enhances code organization but also promotes modularity, making complex tasks more manageable. Whether you are a beginner eager to grasp the fundamentals or an experienced coder looking to refine your skills, understanding how to call a function inside another function is a crucial step in your programming journey.
At its core, calling a function within another function in Python is about leveraging the capabilities of one piece of code to enhance another. This practice allows for the creation of more dynamic and flexible programs, where functions can interact and share data seamlessly. By nesting functions, we can break down larger problems into smaller, more digestible parts, leading to cleaner and more efficient code. This approach not only simplifies debugging but also fosters a deeper understanding of the relationships between different components of your code.
As we delve deeper into this topic, we will explore various scenarios and examples that illustrate the mechanics of function calling in Python. From basic function interactions to more advanced applications, you
Understanding Function Scope
In Python, functions have their own scope, meaning they can access variables defined within them but not those defined outside. When calling a function inside another function, it’s important to understand the scope of variables and how they interact.
- A function can access variables defined in its own scope.
- A function can access variables defined in the global scope.
- A function cannot access variables defined in the scope of another function unless they are passed as parameters.
This concept is critical for writing clean and efficient code, particularly when dealing with nested function calls.
Calling a Function Within Another Function
To call a function inside another function, simply use the function’s name followed by parentheses. If the inner function requires arguments, you must provide them in the call.
Here’s a simple example:
“`python
def greet(name):
return f”Hello, {name}!”
def welcome_user():
user_name = “Alice”
greeting_message = greet(user_name)
print(greeting_message)
welcome_user()
“`
In the above code, the `greet` function is called within the `welcome_user` function. The output will be:
“`
Hello, Alice!
“`
Passing Arguments Between Functions
When calling a function inside another function, you may need to pass arguments. Here’s how to do it effectively:
- Ensure the inner function’s parameters are defined.
- Pass the appropriate arguments from the outer function.
Consider this example:
“`python
def add(a, b):
return a + b
def calculate_sum(x, y):
result = add(x, y)
print(f”The sum is: {result}”)
calculate_sum(5, 3)
“`
This would output:
“`
The sum is: 8
“`
Function Return Values
When a function returns a value, you can capture that value in the calling function. This allows for further processing or use of the returned data.
Function Name | Purpose |
---|---|
greet | Returns a greeting message |
add | Returns the sum of two numbers |
calculate_sum | Captures the result from `add` and prints it |
Here’s an example showcasing this behavior:
“`python
def multiply(x, y):
return x * y
def display_product(a, b):
product = multiply(a, b)
print(f”The product is: {product}”)
display_product(4, 5)
“`
The output will be:
“`
The product is: 20
“`
Best Practices for Nested Function Calls
When working with functions nested within other functions, keep the following best practices in mind:
- Keep functions small and focused: Each function should perform a single task.
- Use descriptive names: This improves readability and maintainability.
- Limit side effects: Functions should avoid changing global state whenever possible.
- Document functions: Use docstrings to explain what each function does.
By adhering to these practices, you can create clear and efficient code that is easier to understand and maintain.
Understanding Function Calls in Python
In Python, functions can be defined and invoked within one another. This is a powerful feature that allows for modular programming and code reusability. Here are the essential steps for calling a function inside another function:
- Define the first function: This is the function that will be called.
- Define the second function: This function will contain the call to the first function.
- Invoke the first function within the second: Use the function name followed by parentheses.
Example of Calling a Function Inside Another Function
Consider the following example:
“`python
def greet(name):
return f”Hello, {name}!”
def welcome_user(username):
message = greet(username)
return message
print(welcome_user(“Alice”))
“`
In this example:
- The `greet` function takes a `name` as an argument and returns a greeting.
- The `welcome_user` function takes a `username`, calls the `greet` function, and returns the greeting message.
- The output will be: `Hello, Alice!`
Passing Arguments Between Functions
When calling a function inside another function, you can pass arguments as needed. Here are some important points to consider:
- Positional Arguments: Passed in the order defined.
- Keyword Arguments: Passed using the syntax `parameter_name=value`.
- Default Arguments: Allow for optional parameters.
Example:
“`python
def multiply(a, b=1):
return a * b
def calculate_square(num):
return multiply(num, num)
print(calculate_square(5)) Output: 25
“`
In this case, `multiply` can utilize both default and positional arguments.
Returning Values from Nested Function Calls
Functions can return values that can be used by the calling function. This allows for flexible and dynamic programming.
Example:
“`python
def add(x, y):
return x + y
def calculate_sum(a, b):
return add(a, b)
result = calculate_sum(10, 20)
print(result) Output: 30
“`
Here, `calculate_sum` calls `add`, and the returned value is stored in `result`.
Best Practices for Nested Function Calls
When working with nested function calls, adhere to the following best practices:
- Keep Functions Focused: Each function should have a single responsibility.
- Use Descriptive Names: Function names should convey their purpose clearly.
- Limit Nesting Levels: Avoid overly complex nesting to maintain readability.
- Document Functions: Use docstrings to explain each function’s purpose and parameters.
Debugging Nested Function Calls
Debugging can be more challenging with nested functions. Consider these strategies:
Strategy | Description |
---|---|
Print Statements | Use print statements to track function calls and variable values. |
Logging | Implement logging to capture detailed information about function execution. |
Breakpoints | Use a debugger to step through code and inspect function calls. |
Unit Tests | Write tests for individual functions to ensure they behave as expected. |
By following these guidelines, you can effectively call and manage functions within each other in Python.
Understanding Function Nesting in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, calling a function inside another function is a fundamental concept that enhances modularity and code organization. It allows developers to break down complex problems into smaller, manageable parts, facilitating easier debugging and maintenance.
James Liu (Lead Python Developer, CodeMasters). When you call a function within another function, you can pass parameters and return values seamlessly. This feature is particularly useful in recursive functions, where the inner function may call itself to solve a problem iteratively.
Sarah Thompson (Python Educator, LearnPythonFast). Understanding how to call a function inside another function is crucial for beginners. It not only helps in grasping the concept of scope and variable accessibility but also lays the groundwork for more advanced programming techniques like decorators and higher-order functions.
Frequently Asked Questions (FAQs)
How do I define a function in Python?
To define a function in Python, use the `def` keyword followed by the function name and parentheses. Inside the parentheses, you can include parameters. For example:
“`python
def my_function(param1, param2):
function body
“`
Can I call a function before it is defined in the code?
No, in Python, a function must be defined before it is called. If you attempt to call a function before its definition, you will encounter a `NameError`.
How do I call a function inside another function in Python?
To call a function inside another function, simply use the function name followed by parentheses within the body of the outer function. Ensure that any required parameters are passed correctly. For example:
“`python
def outer_function():
inner_function()
def inner_function():
print(“Hello from inner function!”)
“`
What happens if the inner function has parameters?
If the inner function has parameters, you must provide the required arguments when calling it from the outer function. For example:
“`python
def outer_function():
inner_function(“Hello”)
def inner_function(message):
print(message)
“`
Can I return values from an inner function?
Yes, you can return values from an inner function. The outer function can capture this return value and use it as needed. For example:
“`python
def outer_function():
result = inner_function()
return result
def inner_function():
return “Hello from inner function!”
“`
Is it possible to call the outer function from the inner function?
Yes, you can call the outer function from the inner function, but be cautious of potential recursion issues. Ensure that the call is necessary and does not lead to infinite recursion. For example:
“`python
def outer_function():
inner_function()
def inner_function():
outer_function() Be careful with this to avoid infinite recursion.
“`
In Python, calling a function inside another function is a fundamental concept that enhances code organization and reusability. This practice allows developers to break down complex tasks into smaller, manageable components. By defining functions that perform specific tasks, programmers can invoke these functions within other functions, thereby creating a modular structure that improves readability and maintainability of the code.
When calling a function within another function, it is essential to consider the scope of variables and the return values. The inner function can access variables defined in its own scope as well as those in the outer function’s scope. However, the outer function cannot access the inner function’s local variables. Understanding this scope hierarchy is crucial for effective function design and avoiding potential errors.
Moreover, utilizing functions within functions can lead to more efficient code execution. For instance, if a certain operation is needed multiple times within a larger function, encapsulating that operation in a separate function reduces redundancy and promotes code reuse. This approach not only simplifies debugging but also enhances collaboration among developers, as functions can be tested independently.
In summary, calling functions within other functions is a powerful technique in Python programming. It fosters better code organization, enhances reusability, and promotes a clear understanding of variable scope
Author Profile
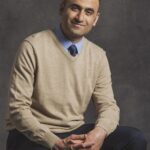
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?