How Can You Clear the Screen in Python?
When working with Python, especially in a console or terminal environment, it’s common to encounter cluttered screens filled with lines of code, outputs, and error messages. This can make it challenging to focus on the task at hand. Whether you’re developing a game, running a script, or simply experimenting with code, the ability to clear the screen can enhance your programming experience and improve readability. In this article, we’ll explore the various methods to clear the screen in Python, ensuring that your workspace remains organized and efficient.
Clearing the screen in Python can be accomplished through several techniques, each suited to different environments and use cases. From utilizing built-in libraries to executing system commands, Python offers flexibility for developers to maintain a clean interface. Understanding these methods not only streamlines your coding process but also helps in creating a more user-friendly experience for those interacting with your scripts.
As we delve deeper into the topic, we will examine the most effective ways to clear the screen, including platform-specific solutions for Windows, macOS, and Linux. We’ll also touch on how to incorporate these techniques into your projects seamlessly, allowing you to focus on what truly matters: writing great code. So, let’s get started and discover how to keep your Python environment tidy and efficient!
Clearing the Screen in Python
In Python, clearing the screen can be accomplished differently depending on the operating system you are using. The most common methods involve using system commands or libraries that interface with the terminal.
Using System Commands
The simplest way to clear the console screen is to use system commands via the `os` module. This method works for both Windows and Unix-based systems, although the commands differ.
- For Windows, the command is `cls`.
- For Unix/Linux/MacOS, the command is `clear`.
Here is how you can implement this in Python:
python
import os
def clear_screen():
# Check if the operating system is Windows
if os.name == ‘nt’:
os.system(‘cls’) # Clear screen command for Windows
else:
os.system(‘clear’) # Clear screen command for Unix/Linux/MacOS
You can call the `clear_screen()` function whenever you need to clear the console.
Using the Subprocess Module
An alternative approach is to use the `subprocess` module, which provides more powerful facilities for spawning new processes and retrieving their results. Here’s how you can do it:
python
import subprocess
def clear_screen():
subprocess.call(‘cls’ if os.name == ‘nt’ else ‘clear’, shell=True)
This method also checks for the operating system and executes the appropriate command.
Considerations for Different Environments
The method you choose may depend on the environment where your Python script is running. For instance, if you are using an Integrated Development Environment (IDE) like PyCharm or Jupyter Notebook, the above methods may not work as expected since these environments do not fully emulate a terminal.
In such cases, you may want to utilize specific features of the IDE or use print statements to create a visual separation of outputs:
python
print(“\n” * 100) # A crude way to push previous output off the screen
Comparison of Methods
Here is a comparison table outlining the different methods for clearing the screen in Python:
Method | Operating System | Code Example |
---|---|---|
os.system | Windows/Unix | os.system(‘cls’ or ‘clear’) |
subprocess.call | Windows/Unix | subprocess.call(‘cls’ or ‘clear’, shell=True) |
Print Newlines | Any | print(“\n” * 100) |
By understanding these methods, you can effectively manage the console output in your Python applications, enhancing user experience and usability.
Clearing the Screen in Python
Clearing the screen in Python can vary depending on the operating system in use. Below are methods to achieve this for both Windows and Unix-like systems, such as Linux and macOS.
Using System Commands
The most straightforward way to clear the screen is by using system commands through the `os` module. This method invokes the shell command appropriate for the operating system.
- For Windows, the command is `cls`.
- For Linux and macOS, the command is `clear`.
Here’s how you can implement this in your Python code:
python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
This function checks the operating system and executes the corresponding command to clear the screen.
Using ANSI Escape Sequences
Another method to clear the terminal screen is by using ANSI escape sequences. This approach is more universal and works in many terminals that support ANSI codes.
To clear the screen using ANSI escape sequences, you can use the following code:
python
def clear_screen():
print(“\033[H\033[J”, end=””)
This code uses escape sequences:
- `\033[H` moves the cursor to the top left corner of the terminal.
- `\033[J` clears the screen from the cursor down to the bottom.
Integration in Applications
When integrating screen-clearing functions into applications, consider the context. For example, in a game or continuous output scenario, clearing the screen can help present information more clearly.
Here is a simple example of a loop that clears the screen before displaying a countdown:
python
import time
def countdown(seconds):
for i in range(seconds, 0, -1):
clear_screen()
print(f”Countdown: {i} seconds remaining”)
time.sleep(1)
clear_screen()
print(“Time’s up!”)
countdown(5)
In this example, the `clear_screen()` function is called within a loop to refresh the display each second, enhancing user experience.
Considerations
- Compatibility: Always ensure that the method used is compatible with the target environment. The `os.system` method is generally reliable across different platforms.
- User Experience: Frequent clearing of the screen may be disorienting for users. Use it judiciously to maintain a smooth user interface.
- Testing: Test your screen-clearing functions in different environments to ensure consistent behavior across platforms.
By utilizing these methods, you can effectively manage screen output in your Python applications, enhancing clarity and user interaction.
Expert Insights on Clearing the Screen in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Clearing the screen in Python can be accomplished using various methods depending on the operating system. The most common approach is to use the ‘os’ module to execute a clear command, which is platform-dependent. For instance, ‘os.system(“cls”)’ works for Windows, while ‘os.system(“clear”)’ is suitable for Unix-based systems.”
Michael Thompson (Python Developer, CodeMaster Solutions). “In addition to using the ‘os’ module, developers can also create a function that clears the screen by printing multiple new lines. While this method is less efficient, it can be useful in environments where system calls are restricted or undesirable.”
Linda Zhang (Technical Writer, Python Programming Journal). “For those using interactive Python environments like Jupyter Notebook, clearing the screen can be achieved by using the ‘IPython.display’ module. The ‘clear_output()’ function is especially handy for creating clean outputs during iterative coding sessions.”
Frequently Asked Questions (FAQs)
How can I clear the screen in Python on Windows?
You can clear the screen in Python on Windows by using the command `os.system(‘cls’)`. This command invokes the Windows command line to clear the console.
What command should I use to clear the screen in Python on Unix/Linux?
For Unix/Linux systems, use `os.system(‘clear’)`. This command executes the clear command available in the terminal to remove all previous output.
Is there a cross-platform method to clear the screen in Python?
Yes, you can use the following code snippet that checks the operating system:
python
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
This ensures compatibility across both Windows and Unix/Linux systems.
Can I clear the screen in Python without using the os module?
While the `os` module is the most common approach, you can also use libraries like `curses` for terminal manipulation, but this is more complex and less straightforward for simple screen clearing.
Does clearing the screen affect the performance of my Python script?
Clearing the screen does not significantly impact performance. However, frequent calls to clear the screen can lead to flickering and may disrupt the user experience in console applications.
Are there any GUI libraries in Python that provide screen clearing functionality?
Yes, GUI libraries such as Tkinter or PyQt allow you to clear or refresh the display area. For example, in Tkinter, you can use the `delete` method on canvas or text widgets to remove existing content.
Clearing the screen in Python can be accomplished using different methods depending on the operating system in use. For Windows users, the command `os.system(‘cls’)` is commonly employed, while Unix-based systems, including Linux and macOS, utilize `os.system(‘clear’)`. These commands effectively remove all previous output from the terminal, providing a clean slate for new information to be displayed.
It is important to note that the `os` module must be imported to use these commands, which can be done with a simple `import os` statement. Additionally, there are alternative approaches, such as using the `subprocess` module, which offers more control and flexibility. This can be particularly useful in complex applications where screen management is required.
In summary, understanding how to clear the screen in Python is a useful skill for enhancing user experience in terminal-based applications. By utilizing the appropriate command for the operating system, developers can ensure that their output remains organized and easy to read. This practice not only improves aesthetics but also aids in debugging and monitoring the program’s performance.
Author Profile
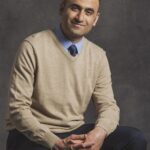
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?