Why Am I Getting ‘Index 0 Out of Bounds for Length 0’ Error?
Have you ever encountered the frustrating error message “index 0 out of bounds for length 0” while coding? If so, you’re not alone. This cryptic phrase often pops up in programming languages like Java, Python, and others, leaving developers scratching their heads. It signifies a common pitfall that can derail even the most seasoned programmers: trying to access an element in an empty collection. As we delve deeper into this topic, we’ll unpack the meaning behind this error, explore its causes, and offer strategies for avoiding it in your coding endeavors.
Overview
At its core, the error “index 0 out of bounds for length 0” indicates that a program is attempting to access the first element of a list, array, or similar data structure that is currently empty. This situation arises when developers assume that a collection contains data without first verifying its contents. Understanding this error is crucial, as it highlights the importance of proper data validation and error handling in programming.
In the world of software development, such errors are not just nuisances; they can lead to significant bugs and system failures if left unaddressed. By examining common scenarios that lead to this error, programmers can learn to anticipate potential pitfalls and implement best practices to ensure their code runs
Error Message Explanation
The error message “index 0 out of bounds for length 0” typically arises in programming contexts when an attempt is made to access an element in an array or list that is empty. This situation often occurs in languages such as Java, Python, and JavaScript, where arrays and lists are common data structures. Understanding the implications of this error is crucial for debugging and ensuring robust code.
When you see this error, it indicates that the program is attempting to access the first element (index 0) of an array or list that has no elements (length 0). This can lead to exceptions or crashes, depending on the language and runtime environment.
Common Causes
Several scenarios can lead to this error:
- Empty Collections: The most direct cause is trying to retrieve an element from an empty collection.
- Conditional Logic Flaws: Failing to account for cases where a collection may not contain elements can lead to erroneous index access.
- Improper Initialization: Not initializing an array or list correctly before accessing its elements.
- Asynchronous Operations: In cases where data is fetched asynchronously, the code may attempt to access data before it is available.
Prevention Techniques
To avoid encountering the “index out of bounds” error, developers can employ several strategies:
- Check for Length: Always verify the length of the array or list before attempting to access its elements.
- Use Try-Catch Blocks: Implement error handling to manage situations where an invalid index might be accessed.
- Logical Conditions: Ensure that conditions leading to index access are sound and account for all possible states of the data structure.
Example of Error Handling
Here’s a simple example in Java that demonstrates how to safely access elements in an array:
“`java
int[] numbers = new int[0]; // Empty array
try {
System.out.println(numbers[0]); // This will throw an exception
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println(“Error: Attempted to access index 0 of an empty array.”);
}
“`
Best Practices
Implementing best practices in coding can significantly reduce the likelihood of encountering this error:
- Use Collections: Prefer using collections (like ArrayList in Java or List in Python) which offer built-in methods to handle size checks.
- Debugging Tools: Utilize debugging tools and IDE features that can help identify potential out-of-bounds errors during development.
- Unit Testing: Write comprehensive unit tests that include scenarios for empty collections to ensure that your code handles such cases gracefully.
Summary Table of Key Practices
Practice | Description |
---|---|
Check Length | Always verify the size of the collection before accessing its elements. |
Try-Catch Blocks | Implement error handling to manage exceptions from invalid index access. |
Logical Conditions | Ensure all logical conditions are in place to handle empty collections. |
Use Collections | Utilize collections that provide methods for safe element access. |
Unit Testing | Write tests that include cases for empty collections to ensure code robustness. |
Understanding the Error
The error message “index 0 out of bounds for length 0” typically arises in programming languages that utilize zero-based indexing, such as Java, Python, and JavaScript. This error indicates that an attempt has been made to access an element at an index that does not exist in the array or collection.
- Zero-Based Indexing: In zero-based indexing, the first element of an array is accessed with index 0. If an array has a length of 0, there are no valid indices to access.
- Common Scenarios:
- Attempting to retrieve an element from an empty array.
- Looping through an array without confirming it contains elements.
- Incorrectly managing the states of dynamic arrays or lists.
Debugging Steps
To address the “index out of bounds” error, follow these debugging steps:
- Check Array Length: Before accessing elements, always verify the length of the array or list.
- Review Logic Flow: Ensure that the code logic properly handles cases where the array might be empty.
- Use Defensive Programming:
- Implement checks before accessing indices.
- Use conditional statements to handle empty cases.
- Print Debug Statements: Log the values of variables leading up to the error to identify miscalculations or unexpected states.
Examples of Error Handling
Here are some programming examples demonstrating how to handle this error properly:
**Java Example**:
“`java
int[] array = new int[0]; // An empty array
if (array.length > 0) {
System.out.println(array[0]); // Safe access
} else {
System.out.println(“Array is empty.”); // Handling the case
}
“`
**Python Example**:
“`python
array = [] An empty list
if len(array) > 0:
print(array[0]) Safe access
else:
print(“List is empty.”) Handling the case
“`
Preventative Measures
To avoid encountering this error in future code, consider implementing the following preventative measures:
- Input Validation: Always validate inputs to functions or methods that expect arrays or lists.
- Use Default Values: When initializing arrays, consider using default values that indicate valid states.
- Error Handling Mechanisms: Utilize try-catch blocks (or equivalent) to gracefully handle exceptions.
Conclusion of Understanding the Error Context
The “index 0 out of bounds for length 0” error can often be mitigated through careful programming practices and thorough testing. By understanding the underlying principles of indexing and implementing the suggested strategies, developers can significantly reduce the likelihood of encountering this issue in their applications.
Understanding the “Index 0 Out of Bounds for Length 0” Error
Dr. Emily Carter (Software Engineer, Tech Solutions Inc.). “The error message ‘index 0 out of bounds for length 0’ typically arises in programming when an attempt is made to access an element in an empty array or list. It serves as a reminder for developers to implement proper checks before accessing data structures to avoid runtime exceptions.”
Mark Thompson (Lead Developer, CodeSafe Technologies). “This specific error highlights a common pitfall in programming—assuming that a data structure contains elements when it may not. Implementing defensive programming techniques, such as validating the size of an array before access, can significantly reduce the occurrence of such errors.”
Lisa Chen (Data Analyst, Insight Analytics). “In data processing, encountering ‘index 0 out of bounds for length 0’ often indicates a flaw in the data pipeline where expected data is missing. It is crucial to ensure that data validation steps are in place to catch these issues early in the workflow.”
Frequently Asked Questions (FAQs)
What does “index 0 out of bounds for length 0” mean?
This error message indicates an attempt to access the first element of an array or list that is currently empty, meaning there are no elements to retrieve.
What causes the “index 0 out of bounds for length 0” error?
The error typically occurs when a program tries to access an element at index 0 of a data structure that has a length of 0, indicating that it has not been initialized or populated with any data.
How can I fix the “index 0 out of bounds for length 0” error?
To resolve this error, ensure that the data structure is properly initialized and contains elements before attempting to access its indices. Implement checks to confirm the length is greater than 0.
Is “index 0 out of bounds for length 0” specific to any programming language?
This error message is common in many programming languages, including Java, Python, and JavaScript, but the exact wording may vary. It generally relates to array or list access violations.
Can this error occur in multi-threaded applications?
Yes, this error can occur in multi-threaded applications if one thread modifies a data structure while another thread attempts to access it, leading to race conditions and potential out-of-bounds access.
What debugging techniques can help identify the cause of this error?
Utilize debugging tools to step through the code and monitor the state of data structures. Implement logging to track their initialization and population, which can help pinpoint where the error originates.
The error message “index 0 out of bounds for length 0” typically occurs in programming contexts, particularly when working with data structures such as arrays or lists. This message indicates that an attempt was made to access an element at index 0 of a collection that is currently empty. Understanding this error is crucial for developers, as it highlights issues in the logic or flow of the code that need to be addressed to prevent runtime exceptions.
One of the main points to consider is the importance of validating data structures before attempting to access their elements. Implementing checks to ensure that a collection is not empty can help avoid this error. For instance, using conditional statements to verify the length of an array or list before accessing its elements can enhance the robustness of the code and improve overall program stability.
Additionally, this error serves as a reminder of the significance of proper error handling in programming. By anticipating potential issues and incorporating exception handling mechanisms, developers can create more resilient applications that gracefully manage unexpected scenarios. This approach not only improves user experience but also aids in debugging and maintaining code quality.
In summary, the “index 0 out of bounds for length 0” error underscores the necessity for careful data management and error handling in programming
Author Profile
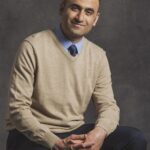
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?