How Can I Use Bash to Loop Over Lines in a File?
In the world of programming and scripting, efficiency is key, and when it comes to processing data, few tools are as powerful as Bash. For anyone who has ever needed to manipulate or analyze text files, understanding how to loop over lines in a file can unlock a treasure trove of possibilities. Whether you’re automating mundane tasks, extracting valuable insights, or simply organizing information, mastering this fundamental skill can significantly enhance your productivity and streamline your workflows.
Bash provides a straightforward yet versatile way to handle files, allowing users to read and process each line individually. This capability is particularly useful for tasks such as parsing logs, transforming data formats, or even generating reports. By leveraging simple loop constructs, you can iterate through each line of a file, applying various operations or commands that suit your specific needs.
As we delve deeper into the mechanics of looping over lines in a file using Bash, we will explore different methods and best practices. From basic loops to more advanced techniques, this guide will equip you with the knowledge to harness the full potential of Bash scripting, enabling you to tackle a wide range of text processing challenges with confidence and ease. Whether you’re a seasoned developer or a curious beginner, there’s something here for everyone looking to elevate their command-line skills.
Using `while` Loop with File Descriptors
A common way to loop over lines in a file in Bash is by using a `while` loop combined with file descriptors. This method reads the file line by line, ensuring that the entire content can be processed efficiently without loading the whole file into memory.
Here is a basic example of this technique:
“`bash
while IFS= read -r line; do
echo “$line”
done < filename.txt
```
In this script:
- `IFS=` prevents leading/trailing whitespace from being trimmed.
- `read -r` reads the line without interpreting backslashes as escape characters.
- `done < filename.txt` specifies the input file to read.
Using `for` Loop with `cat`
Another approach involves using a `for` loop with the `cat` command. This method is simpler but less efficient for large files, as it may load the entire file into memory.
Example:
“`bash
for line in $(cat filename.txt); do
echo “$line”
done
“`
However, this approach can lead to issues with lines containing spaces or special characters. Therefore, it’s generally recommended to use the `while` loop for more robust handling of file contents.
Looping with `awk`
`awk` is a powerful text-processing tool that can also be utilized to loop over lines in a file. It allows for more complex operations and is particularly useful for formatted text or when specific fields need to be accessed.
Example:
“`bash
awk ‘{ print $0 }’ filename.txt
“`
In this example, `awk` processes each line of the file and prints it. The `$0` variable represents the entire line.
Looping with `sed`
Similar to `awk`, `sed` can be used to read and process files line by line. Although primarily a stream editor, it can be combined with loops for specific tasks.
Example:
“`bash
sed -n ‘p’ filename.txt
“`
Here, `-n` suppresses automatic printing, and `p` prints each line, allowing for further processing.
Comparison Table
Method | Efficiency | Complexity | Best Use Case |
---|---|---|---|
while loop | High | Moderate | General file processing |
for loop with cat | Low | Low | Small files, simple processing |
awk | Moderate | High | Field-based processing |
sed | Moderate | High | Stream editing tasks |
These methods provide flexibility and power when handling files in Bash scripts, allowing users to choose the best approach based on their specific needs and the characteristics of the data they are processing.
Bash Loop Over Lines in File
When working with files in Bash, looping over each line is a common task. There are several methods to achieve this, each suited to different scenarios. Below are the most frequently used approaches.
Using `while` Loop
The `while` loop is one of the most effective ways to read a file line by line. This method uses the `read` command to read each line and process it accordingly.
“`bash
while IFS= read -r line; do
echo “$line” Process the line
done < filename.txt
```
Key Components:
- `IFS=`: Prevents leading/trailing whitespace from being trimmed.
- `-r`: Prevents backslashes from being interpreted as escape characters.
- `< filename.txt`: Redirects the file input into the loop.
Using `for` Loop with `cat`
Another approach involves piping the file content into a `for` loop. This method is less preferred for files with spaces in names but is straightforward for many use cases.
“`bash
for line in $(cat filename.txt); do
echo “$line” Process the line
done
“`
Considerations:
- This method splits lines based on whitespace, which can lead to unexpected behavior with lines containing spaces.
Using `mapfile` Command
The `mapfile` command (also known as `readarray`) allows you to read lines from a file directly into an array. This can be particularly useful for processing multiple lines later in the script.
“`bash
mapfile -t lines < filename.txt
for line in "${lines[@]}"; do
echo "$line" Process the line
done
```
Advantages:
- Efficient for processing large files.
- Retains line breaks and whitespace.
Using `awk` for Advanced Processing
For more advanced text processing, `awk` can be utilized. This tool is powerful for handling complex patterns and conditions.
“`bash
awk ‘{print}’ filename.txt
“`
Features:
- Can perform calculations or modify the output format.
- Allows for inline processing and filtering with conditions.
Method | Pros | Cons |
---|---|---|
`while` loop | Handles whitespace well | Slightly slower for huge files |
`for` loop | Simple syntax | Poor handling of spaces |
`mapfile` | Efficient for large files | May not be available in all shells |
`awk` | Powerful text processing | Requires familiarity with syntax |
Using `sed` for Line Processing
The `sed` command can also be employed to process lines in a file, allowing for substitution and deletion operations.
“`bash
sed -n ‘p’ filename.txt
“`
Features:
- Ideal for in-place edits and transformations.
- Can be combined with other commands for enhanced functionality.
These methods provide a robust toolkit for iterating over lines in a file using Bash. Depending on the specific requirements of your script, you can choose the most appropriate approach for optimal performance and readability.
Expert Insights on Looping Over Lines in Bash Files
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When looping over lines in a file using Bash, it is essential to utilize the `while read` construct. This method ensures that you handle each line efficiently, especially with files containing spaces or special characters, which can be problematic with simpler approaches.”
Mark Thompson (Linux System Administrator, TechOps Magazine). “Using a `for` loop with `cat` can be tempting, but it is generally less efficient than using a `while` loop. The `while read` approach allows you to read lines one at a time, which is particularly advantageous for large files, minimizing memory usage.”
Lisa Chen (DevOps Consultant, Cloud Innovations). “It is crucial to remember that when processing files in Bash, you should always consider the input format. For instance, if the file contains trailing newlines or unexpected line endings, using `IFS` (Internal Field Separator) can help manage how lines are read and processed.”
Frequently Asked Questions (FAQs)
How do I loop over lines in a file using bash?
You can use a `while` loop combined with the `read` command. For example:
“`bash
while IFS= read -r line; do
echo “$line”
done < filename.txt
```
What does the `IFS` variable do in a bash loop?
The `IFS` (Internal Field Separator) variable defines how bash recognizes word boundaries. Setting `IFS=` ensures that leading/trailing whitespace is preserved when reading lines.
Can I loop through a file with a `for` loop instead?
Yes, you can use a `for` loop, but it splits the file into words rather than lines. A common approach is:
“`bash
for line in $(cat filename.txt); do
echo “$line”
done
“`
However, this method may not handle spaces correctly.
How can I process each line in a file without using a temporary variable?
You can directly process the line within the loop without storing it in a variable. For example:
“`bash
while IFS= read -r; do
echo “$REPLY”
done < filename.txt
```
Here, `$REPLY` contains the current line.
Is there a way to loop over lines in a file while also handling empty lines?
Yes, using the `read` command with `-r` prevents backslashes from being interpreted, and you can include a check for empty lines:
“`bash
while IFS= read -r line || [[ -n $line ]]; do
echo “$line”
done < filename.txt
```
What are some common pitfalls when looping over lines in a file in bash?
Common pitfalls include not preserving whitespace, failing to handle empty lines, and using `for` loops that split lines incorrectly. Always use `while read` for line-by-line processing to avoid these issues.
Bash scripting provides a powerful way to automate tasks in Unix-like operating systems, and looping over lines in a file is a common requirement for many scripts. The primary method to achieve this is through the use of the `while` loop in combination with the `read` command. This approach allows for efficient processing of each line in a file, enabling users to manipulate or analyze data line by line without loading the entire file into memory.
Another method involves using the `for` loop, which can iterate over the output of commands like `cat` or `grep`. However, this method may not handle lines with spaces correctly, making the `while read` approach more robust for most scenarios. Additionally, using `IFS` (Internal Field Separator) can help manage how lines are read, particularly when dealing with delimited data. Overall, understanding these techniques is essential for effective file manipulation in bash scripting.
Key takeaways include the importance of choosing the right looping construct based on the specific requirements of the task at hand. The `while read` method is generally preferred for its reliability and ability to handle complex line structures. Furthermore, incorporating error handling and input validation can enhance the robustness of scripts that process files. Mastery of these techniques not
Author Profile
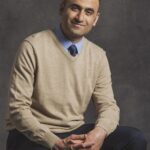
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?