How Can You Fetch Objects Without a Limit Using Collection.Query?
In the ever-evolving landscape of data management and retrieval, the ability to efficiently access and manipulate collections of objects is paramount for developers and data scientists alike. One common challenge that arises in this realm is the need to fetch objects without imposing limits on the number of results returned. Whether you’re working with large datasets in a database or managing collections in a programming environment, understanding how to perform a `collection.query.fetch` operation without constraints can significantly enhance your application’s performance and user experience.
This article delves into the intricacies of fetching objects from collections without limits, exploring the underlying principles and best practices that govern this process. We will examine the various scenarios in which unrestricted data retrieval is beneficial, as well as the potential pitfalls that can arise when handling extensive datasets. By understanding the mechanics of `collection.query.fetch`, you can optimize your data queries and ensure that your applications remain responsive and efficient, even when dealing with vast amounts of information.
As we navigate through the nuances of this topic, we will also highlight the importance of implementing effective data management strategies. From leveraging indexing techniques to understanding the implications of memory usage, our exploration will equip you with the knowledge necessary to harness the full potential of your data collections. Get ready to unlock the secrets of limitless data fetching and elevate your programming prowess
Understanding Collection Query Fetching
When dealing with a collection in a database or a data structure, fetching objects is a crucial operation. Typically, queries are designed to return a limited number of objects to optimize performance and manage resource usage. However, there are scenarios where it might be necessary to fetch all available objects without imposing any limits. This practice can be beneficial in data analysis, reporting, or when the full dataset is required for processing.
Fetching Objects with No Limit
To fetch objects without any limits, the query syntax and capabilities of the database or data structure in use must be understood. Many systems provide options to specify the number of records returned, but when no limit is set, all records can be retrieved in one operation.
Key considerations include:
- Performance: Fetching a large number of objects at once can lead to performance degradation. It is vital to ensure that the system can handle the load.
- Memory Usage: Returning a significant volume of data may consume substantial memory resources. Monitoring the application’s memory footprint becomes essential.
- Network Overhead: For distributed systems, transferring large data sets can increase network latency and bandwidth usage.
Example Syntax for Fetching
The exact syntax for fetching objects without a limit will depend on the programming language and database management system (DBMS) in use. Below are examples for two common systems:
System | Query Syntax |
---|---|
SQL | SELECT * FROM table_name; |
NoSQL (MongoDB) | db.collection.find({}); |
Considerations for No Limit Fetching
Before executing a fetch operation without limits, consider the following:
- Pagination: Implementing pagination can help manage large datasets by breaking them into smaller, more manageable chunks.
- Filtering: Even when fetching without limits, applying filters can help reduce the dataset size and increase query efficiency.
- Indexing: Ensuring proper indexing can significantly improve performance when querying large datasets.
Best Practices
When fetching objects without a limit, adhere to these best practices to mitigate potential issues:
- Monitor Performance: Regularly assess the impact on application performance and adjust queries as needed.
- Use Streaming: If supported, consider using streaming techniques to handle large datasets incrementally rather than loading everything into memory at once.
- Test with Sample Data: Before deploying queries on large datasets, test them with smaller datasets to identify potential bottlenecks.
By understanding the implications of fetching objects without limits and applying best practices, developers can effectively manage data retrieval processes in their applications.
Fetching Objects Without Limit
When working with collections in various programming environments, the ability to fetch objects without a specified limit can be crucial for applications that require comprehensive data retrieval. Below are methods and considerations for executing such queries effectively.
Using Collection.Query in Different Frameworks
Different frameworks and libraries have their own syntax and methods for fetching data without constraints. The following outlines a few popular ones:
JavaScript (with MongoDB)
In MongoDB, you can use the `find()` method to retrieve all documents from a collection. The absence of a limit means all documents will be fetched.
“`javascript
db.collection.find({});
“`
Python (with SQLAlchemy)
In SQLAlchemy, you can execute queries without limits by simply omitting the `limit()` method.
“`python
query = session.query(YourModel)
results = query.all()
“`
PHP (with Laravel)
In Laravel, retrieving all records from a model can be accomplished using the `all()` method, which does not impose any limit.
“`php
$items = YourModel::all();
“`
Performance Considerations
Fetching an unlimited number of records can lead to performance issues. Key considerations include:
- Memory Consumption: Retrieving large datasets can exhaust server memory.
- Response Time: The more data fetched, the longer the response time.
- Network Latency: Large payloads can increase data transmission time.
Best Practices for Fetching Data
When dealing with unlimited data fetching, consider the following best practices:
- Pagination: Implement pagination to fetch data in manageable chunks, improving performance and user experience.
- Filtering: Apply filters to narrow down results based on specific criteria, ensuring only relevant data is retrieved.
- Asynchronous Processing: Use asynchronous methods to prevent blocking the main thread during data fetching operations.
- Caching: Store frequently accessed data in cache to reduce the need for repeated queries.
Example of Pagination Implementation
Here is a simple example of how pagination can be structured, taking JavaScript with MongoDB as a reference:
“`javascript
const pageSize = 10; // Number of records per page
const pageNumber = 1; // Current page number
db.collection.find({})
.skip((pageNumber – 1) * pageSize)
.limit(pageSize);
“`
This structure allows for fetching data in smaller, more manageable portions, thereby optimizing performance and resource usage.
Efficient data retrieval is essential for application performance. By leveraging appropriate methods and practices, one can fetch objects from collections without limits while maintaining system integrity and user experience.
Expert Insights on Fetching Objects Without Limits in Collections
Dr. Emily Carter (Data Architect, Tech Innovations Inc.). “When querying collections without a limit, it is crucial to consider the implications on performance and memory usage. Fetching an unbounded number of objects can lead to significant overhead, especially in large datasets, which may ultimately degrade application responsiveness.”
James Liu (Senior Software Engineer, Cloud Solutions Co.). “While fetching objects without a limit can simplify certain operations, it is essential to implement pagination or lazy loading strategies. This approach not only enhances user experience but also ensures that backend systems remain efficient and scalable.”
Maria Gonzalez (Database Administrator, DataSafe Systems). “In my experience, executing collection queries without a limit can lead to unexpected results, especially when dealing with concurrent modifications. Ensuring data consistency and integrity should always be a priority, which is why I advocate for controlled fetching practices.”
Frequently Asked Questions (FAQs)
What does it mean to fetch objects with no limit in a collection query?
Fetching objects with no limit in a collection query refers to retrieving all available records from a specified dataset without imposing any restrictions on the number of results returned.
How can I implement a collection query to fetch objects with no limit?
To implement a collection query without limits, you typically use a query method or function that does not specify a maximum number of results, such as `collection.query.fetch()` in programming frameworks that support such operations.
Are there performance considerations when fetching objects with no limit?
Yes, fetching a large number of objects without limit can lead to performance issues, including increased memory usage and slower response times. It is advisable to implement pagination or filtering to manage large datasets effectively.
Can I apply filters while fetching objects with no limit?
Yes, you can apply filters to narrow down the results while still fetching all matching records. This allows you to retrieve a manageable subset of data based on specific criteria.
What are the potential risks of fetching too many objects at once?
Fetching too many objects at once can result in application crashes, timeouts, and excessive load on the database server. It may also lead to poor user experience due to slow loading times.
Is there a way to limit the number of objects fetched after an initial unlimited query?
Yes, after an initial unlimited query, you can apply additional logic in your application to limit the results, such as slicing the dataset or using pagination techniques to display a subset of the total records.
In the context of using collection.query.fetch to retrieve objects without a specified limit, it is essential to understand the implications of such an approach. Fetching objects without a limit can lead to performance issues, especially when dealing with large datasets. This method retrieves all available records, which may result in excessive memory usage and slower response times. Therefore, it is crucial to consider the size and structure of the dataset before executing such queries.
Additionally, best practices suggest implementing pagination or setting reasonable limits to manage the volume of data being processed. By doing so, developers can enhance application performance and provide a better user experience. It is also important to incorporate error handling and data validation to ensure that the fetched data meets the necessary criteria for further processing.
In summary, while using collection.query.fetch without a limit may seem convenient for retrieving all objects, it is vital to weigh the potential drawbacks against the benefits. Adopting strategies such as pagination and data management techniques can significantly improve efficiency and maintain system integrity. Ultimately, a thoughtful approach to data fetching can lead to more robust applications and optimized performance.
Author Profile
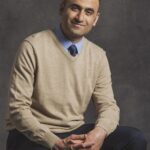
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?