How Can You Create a File in Python? A Step-by-Step Guide
Creating files is a fundamental task in programming, and Python makes it incredibly straightforward. Whether you’re a budding programmer or an experienced developer, understanding how to create a file in Python opens up a world of possibilities for data storage, manipulation, and retrieval. Imagine being able to seamlessly log information, save user inputs, or even generate reports—all with just a few lines of code. In this article, we will explore the various methods available in Python for file creation, equipping you with the skills to manage your data effectively.
At its core, file creation in Python involves using built-in functions that allow you to open or create files with ease. Python’s intuitive syntax and rich standard library provide multiple approaches, whether you need to create a simple text file or a more complex data structure. Understanding the difference between reading, writing, and appending to files is crucial, as it will help you choose the right method for your specific needs.
Moreover, Python’s versatility extends beyond just creating files; it also offers robust error handling and file management capabilities. This ensures that your programs can handle unexpected situations gracefully, such as file permission issues or disk space limitations. As we delve deeper into the mechanics of file creation in Python, you will discover best practices and tips that will enhance your programming toolkit and
Using the `open()` Function
To create a file in Python, the most fundamental method is using the built-in `open()` function. This function opens a file, allowing you to create a new one if it doesn’t already exist. The syntax for `open()` is as follows:
python
file_object = open(‘filename.txt’, ‘mode’)
In this syntax:
- `filename.txt` is the name of the file you want to create.
- `mode` specifies the mode in which the file is opened. For creating a file, you can use either `’w’` (write mode) or `’x’` (exclusive creation mode).
The following modes can be used:
- `’w’`: Opens a file for writing. If the file already exists, it will be truncated.
- `’x’`: Creates a new file. If the file exists, an error will be raised.
- `’a’`: Opens a file for appending. The file pointer is placed at the end of the file if it exists.
Example of creating a file:
python
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
The `with` statement ensures that the file is properly closed after its suite finishes.
Writing to a File
Once a file is created, you can write data to it. The `write()` method allows you to add text to the file. If you want to write multiple lines, you can use `writelines()`, which takes an iterable.
Example of writing multiple lines:
python
lines = [‘First line\n’, ‘Second line\n’, ‘Third line\n’]
with open(‘example.txt’, ‘w’) as file:
file.writelines(lines)
Table of File Modes
Mode | Description | File Behavior |
---|---|---|
‘w’ | Write (create or truncate) | Creates a new file or truncates an existing file |
‘x’ | Exclusive creation | Creates a new file, fails if it already exists |
‘a’ | Append | Creates a new file or appends to an existing file |
‘r’ | Read | Opens a file for reading |
Handling Exceptions
When creating or writing to files, it is important to handle exceptions that may arise, such as `FileNotFoundError` or `PermissionError`. You can use a try-except block to manage these errors effectively.
Example:
python
try:
with open(‘example.txt’, ‘x’) as file:
file.write(‘This file is created safely!’)
except FileExistsError:
print(“The file already exists.”)
This ensures that your program can handle situations where the file cannot be created without crashing.
Creating a File in Python
To create a file in Python, you can utilize the built-in `open()` function, which opens a file and allows you to specify the mode in which you want to work with it. The most common modes for file creation are:
- `’w’`: Write mode. This will create a new file or truncate an existing file to zero length.
- `’x’`: Exclusive creation. This mode will create a new file but will fail if the file already exists.
- `’a’`: Append mode. This will create a new file if it does not exist or append to the end of an existing file.
Basic File Creation Example
Here is a simple example demonstrating how to create a file and write text to it:
python
# Creating a new file and writing text to it
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!\n’)
file.write(‘This is a file created in Python.’)
In this example:
- The `with` statement is used to open the file, ensuring that it is properly closed after the block is executed.
- The `write()` method writes the specified string to the file.
Handling Exceptions
When creating files, it is essential to handle potential exceptions that may arise, such as permission errors or directory issues. Below is an example of how to handle these exceptions:
python
try:
with open(‘example.txt’, ‘x’) as file:
file.write(‘This file will only be created if it does not exist.’)
except FileExistsError:
print(“File already exists.”)
except IOError as e:
print(f”An IOError occurred: {e}”)
This code attempts to create a new file and gracefully handles the situation if the file already exists, ensuring a smooth user experience.
Writing Multiple Lines
To write multiple lines to a file, you can use a list and the `writelines()` method. Here’s how:
python
lines = [‘First line\n’, ‘Second line\n’, ‘Third line\n’]
with open(‘example_lines.txt’, ‘w’) as file:
file.writelines(lines)
In this example:
- Each line in the list is written to the file in sequence.
- Note that `writelines()` does not add newline characters automatically, so they must be included in the strings.
Using File Modes Effectively
Here’s a quick reference table for different file modes:
Mode | Description |
---|---|
`w` | Write mode; creates or truncates a file. |
`x` | Exclusive creation; fails if file exists. |
`a` | Append mode; creates if not exists; adds to the end. |
`r` | Read mode; opens a file for reading. |
While the above sections cover basic file creation and writing techniques in Python, there are many additional functionalities available, such as reading from files, modifying file contents, and working with different file formats. These capabilities enable robust file manipulation in various applications.
Expert Insights on Creating Files in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Creating a file in Python is a straightforward process that can be accomplished using the built-in `open()` function. It’s essential to specify the mode, such as ‘w’ for writing or ‘a’ for appending, to ensure that the file is created or modified as intended.”
Michael Thompson (Lead Python Developer, CodeCrafters). “When working with file creation in Python, I recommend using the `with` statement. This approach not only simplifies the syntax but also ensures that files are properly closed after their suite finishes, which is crucial for resource management.”
Sarah Patel (Data Scientist, Analytics Hub). “For data-driven applications, it is important to create files in formats like CSV or JSON. Utilizing libraries such as `csv` and `json` in Python can streamline the process and enhance data integrity when generating these files.”
Frequently Asked Questions (FAQs)
How do I create a text file in Python?
You can create a text file in Python using the built-in `open()` function with the mode set to `’w’` for writing. For example: `with open(‘filename.txt’, ‘w’) as file: file.write(‘Hello, World!’)`.
What is the difference between ‘w’ and ‘a’ modes in file creation?
The `’w’` mode opens a file for writing and truncates the file if it already exists, while the `’a’` mode opens a file for appending data without truncating it, allowing you to add new content at the end of the file.
Can I create a file in a specific directory using Python?
Yes, you can create a file in a specific directory by providing the full path in the `open()` function. For instance: `with open(‘/path/to/directory/filename.txt’, ‘w’) as file:`.
What happens if I try to create a file that already exists?
If you use the `’w’` mode, the existing file will be truncated and overwritten. If you use the `’a’` mode, the existing file will remain intact, and new content will be added at the end.
How can I handle exceptions when creating a file in Python?
You can handle exceptions using a `try-except` block. For example:
python
try:
with open(‘filename.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
except IOError as e:
print(f”An error occurred: {e}”)
Is it possible to create a binary file in Python?
Yes, you can create a binary file by opening it in binary mode using `’wb’`. For example: `with open(‘filename.bin’, ‘wb’) as file: file.write(b’Binary data’)`.
In summary, creating a file in Python is a straightforward process that can be accomplished using built-in functions and methods. The most common way to create a file is by utilizing the `open()` function, which allows you to specify the file name and the mode in which the file should be opened. The ‘w’ mode is typically used for writing, which creates a new file if it does not exist or truncates the file if it does. Additionally, using the ‘x’ mode can ensure that a file is created only if it does not already exist, preventing accidental overwriting.
Moreover, it is essential to manage file operations properly to avoid resource leaks. This can be achieved by using the `with` statement, which automatically handles the closing of the file after its suite finishes execution. This practice not only enhances code readability but also ensures that files are properly closed, reducing the risk of data corruption or loss.
Key takeaways include understanding the different file modes available in Python, such as ‘r’, ‘w’, ‘a’, and ‘x’, and their implications on file creation and manipulation. Additionally, embracing the use of context managers can significantly improve file handling practices in Python, leading to more robust and maintainable code.
Author Profile
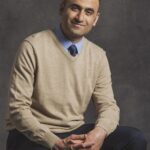
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?