How Can You Create a Program in Python? A Step-by-Step Guide
In today’s digital age, programming has become an essential skill, opening doors to countless opportunities across various fields. Among the myriad of programming languages available, Python stands out as one of the most accessible and versatile options for both beginners and seasoned developers alike. With its clean syntax and robust libraries, Python empowers users to create everything from simple scripts to complex applications with ease. If you’ve ever wondered how to create a program in Python, you’re in the right place. This article will guide you through the fundamental concepts and steps necessary to embark on your programming journey.
Creating a program in Python involves understanding the basic building blocks of the language, such as variables, data types, and control structures. These foundational elements serve as the backbone of any Python application, enabling you to manipulate data and implement logic effectively. Whether you’re aiming to automate mundane tasks, analyze data, or develop web applications, grasping these core concepts is crucial for your success.
As you delve deeper into the world of Python programming, you’ll discover a wealth of libraries and frameworks that can enhance your projects and streamline your development process. From web frameworks like Flask and Django to data analysis libraries like Pandas and NumPy, Python offers a rich ecosystem that caters to diverse programming needs. By following the steps outlined in this article
Setting Up Your Development Environment
To create a program in Python, the first step is to set up your development environment. This involves installing Python and selecting an integrated development environment (IDE) or text editor. Here are some essential steps:
- Install Python: Download the latest version of Python from the official website (https://www.python.org/downloads/). Follow the installation instructions specific to your operating system.
- Choose an IDE/Text Editor:
- Popular options include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Atom
- Select one based on your preference for features, usability, and language support.
Creating Your First Python Program
Once your environment is set up, you can start writing your first Python program. This typically involves creating a new file, writing your code, and then executing it. Follow these steps:
- Create a New File: Open your IDE or text editor and create a new file. Save it with a `.py` extension, for example, `hello.py`.
- Write Your Code: Enter the following simple program that prints “Hello, World!” to the console:
“`python
print(“Hello, World!”)
“`
- Run Your Program: The method to run your program depends on your chosen IDE or editor. For example:
- In a terminal or command prompt, navigate to the directory containing your file and run:
“`
python hello.py
“`
- In an IDE, there is usually a run button or option available.
Understanding Python Syntax
Python syntax is designed to be clear and readable. Here are some fundamental concepts to grasp:
- Variables: Used to store data.
- Data Types: Common types include integers, floats, strings, and lists.
- Control Structures: Include conditionals (`if`, `else`) and loops (`for`, `while`).
A brief overview of data types:
Data Type | Description | Example |
---|---|---|
int | Integer values | 5 |
float | Floating-point numbers | 3.14 |
str | Strings of characters | “Hello” |
list | Ordered collections of items | [1, 2, 3] |
Debugging and Testing Your Code
Debugging is a critical part of programming. Python provides several methods for identifying and fixing issues in your code:
- Print Statements: Use `print()` to display variable values and flow of execution.
- Error Messages: Pay attention to error messages; they often indicate where the problem lies.
- IDEs with Debugging Tools: Many IDEs come with built-in debugging tools that allow you to set breakpoints, step through code, and inspect variables.
Expanding Your Knowledge
Once you are comfortable with the basics, consider exploring more advanced topics in Python:
- Functions: Learn how to create reusable blocks of code.
- Modules and Libraries: Utilize Python’s extensive libraries for various tasks (e.g., NumPy for numerical computations).
- Object-Oriented Programming: Understand the principles of classes and objects.
By following these guidelines and practices, you will be well on your way to creating effective programs in Python.
Setting Up Your Python Environment
To create a program in Python, the first step involves setting up your development environment. This includes installing Python and selecting an Integrated Development Environment (IDE) or text editor.
- Install Python:
- Visit the official Python website: [python.org](https://www.python.org/downloads/).
- Download the latest version suitable for your operating system (Windows, macOS, or Linux).
- Follow the installation instructions, making sure to check the box that adds Python to your system PATH.
- Choose an IDE or Text Editor:
Several options are available, catering to different preferences:
- PyCharm: A powerful IDE specifically designed for Python development.
- Visual Studio Code: A lightweight, customizable editor with extensive Python support through extensions.
- Jupyter Notebook: Ideal for data analysis and interactive coding.
- Sublime Text: A fast, versatile text editor that supports multiple languages.
Writing Your First Python Program
After setting up your environment, the next step is to write your first Python program. This program will typically be a simple “Hello, World!” example.
- Open your chosen IDE or text editor.
- Create a new file: Name it `hello.py`.
- Write the following code:
“`python
print(“Hello, World!”)
“`
- Save the file.
Running Your Python Program
To execute the program, follow these steps:
- Using Command Line:
- Open your command line interface (Terminal on macOS/Linux or Command Prompt on Windows).
- Navigate to the directory where your `hello.py` file is saved.
- Run the command:
“`bash
python hello.py
“`
- You should see the output: `Hello, World!`
- Using IDE:
- In most IDEs, simply click the ‘Run’ button or use a designated keyboard shortcut (usually F5 or Ctrl+Shift+R).
Understanding Python Syntax and Structure
Familiarizing yourself with Python syntax is crucial. Python is known for its readability and simplicity. Here are some fundamental concepts:
– **Variables**: Used to store data.
- Example: `name = “Alice”`
– **Data Types**: Common types include:
- Integer: `int`
- Floating-point: `float`
- String: `str`
- Boolean: `bool`
– **Control Structures**: Used for decision-making.
– **If Statement**:
“`python
if age >= 18:
print(“Adult”)
“`
- Loops: For repeated execution.
- For Loop:
“`python
for i in range(5):
print(i)
“`
Creating Functions and Modules
Functions are essential for structuring your program. They allow for code reuse and better organization.
- Defining a Function:
“`python
def greet(name):
return f”Hello, {name}!”
“`
- Calling a Function:
“`python
print(greet(“Alice”))
“`
- Modules: You can organize related functions into modules. Create a file named `mymodule.py` and add functions. Import it in your main program:
“`python
import mymodule
“`
Debugging and Testing Your Program
Debugging is a critical aspect of programming. Here are strategies for effective debugging:
- Print Statements: Use `print()` to display variable values.
- Python Debugger (pdb): A built-in module for stepping through code.
- Unit Testing: Use the `unittest` module to create test cases for your functions.
Debugging Technique | Description |
---|---|
Print Statements | Quick checks of variable values and flow |
pdb | Step through code execution |
unittest | Automated testing of functions and methods |
By following these structured steps, you can efficiently create, run, and debug Python programs.
Expert Insights on Creating Programs in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To create a program in Python, it is crucial to start with a clear understanding of the problem you aim to solve. This involves defining the requirements and planning the architecture before diving into coding. A well-structured approach will lead to more maintainable and efficient code.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Utilizing Python’s extensive libraries can significantly accelerate the development process. Leveraging existing modules for tasks such as data manipulation or web scraping allows developers to focus on the unique aspects of their application, enhancing productivity and reducing redundancy.”
Sarah Patel (Educational Technology Specialist, LearnPython.org). “For beginners, starting with small, manageable projects is essential. This fosters confidence and reinforces learning. As skills develop, progressively tackling more complex projects will deepen understanding and proficiency in Python programming.”
Frequently Asked Questions (FAQs)
What are the basic steps to create a program in Python?
To create a program in Python, start by installing Python on your machine. Next, choose a code editor or an Integrated Development Environment (IDE) such as PyCharm or VS Code. Write your code in a new file with a `.py` extension, and then run the program using the command line or the IDE’s built-in features.
How do I install Python on my computer?
You can install Python by downloading the installer from the official Python website (python.org). Choose the version compatible with your operating system, run the installer, and follow the prompts to complete the installation. Ensure to check the box to add Python to your system PATH.
What is the difference between a script and a module in Python?
A script is a standalone file containing Python code that is executed as a program. A module, on the other hand, is a file containing Python definitions and statements that can be imported and reused in other scripts or modules, promoting code reusability.
How do I run a Python program from the command line?
To run a Python program from the command line, open the terminal or command prompt, navigate to the directory containing your `.py` file, and type `python filename.py`, replacing `filename.py` with the name of your script. Press Enter to execute the program.
What are some common errors to avoid when writing Python code?
Common errors include syntax errors, indentation errors, and type errors. Ensure proper indentation, use correct syntax for functions and statements, and be mindful of data types when performing operations to avoid these issues.
How can I debug my Python program effectively?
To debug a Python program, use built-in tools like `print()` statements to track variable values and program flow. Additionally, consider using a debugger available in your IDE, or leverage Python’s `pdb` module for more advanced debugging techniques.
Creating a program in Python involves several key steps that guide you from the initial concept to a functional application. First, it is essential to define the problem you want to solve or the task you want to automate. This clarity will help you determine the necessary features and functionalities of your program. Next, you should familiarize yourself with Python’s syntax and basic programming concepts, such as variables, data types, control structures, functions, and object-oriented programming. Understanding these fundamentals is crucial for writing efficient and effective code.
Once you have a solid grasp of the basics, you can start designing your program. This includes outlining the program’s structure, creating flowcharts, or writing pseudocode to visualize the logic before diving into actual coding. After planning, you can begin writing the code in a Python environment, such as an Integrated Development Environment (IDE) or a text editor. During this phase, it is important to test your code frequently to identify and fix any errors early in the development process.
Finally, after completing your program, you should focus on refining and optimizing your code. This includes adding comments for clarity, improving performance, and ensuring that the code is maintainable. Additionally, consider sharing your program with others for feedback and potential collaboration.
Author Profile
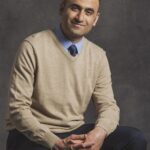
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?