How Can You Format Decimals in Python for Clearer Output?
When working with numerical data in Python, the way we present our results can be just as important as the calculations themselves. Whether you’re developing a financial application, creating a data visualization, or simply displaying results for user interaction, formatting decimals correctly is crucial for clarity and professionalism. In Python, there are various methods to achieve this, each offering flexibility and precision to meet your specific needs. Understanding how to format decimals not only enhances the readability of your output but also ensures that your data conveys the intended meaning without ambiguity.
In Python, formatting decimals involves controlling the number of digits displayed after the decimal point, which can be essential in fields such as finance, science, and engineering. The language provides several tools to format numbers, including f-strings, the `format()` method, and the older `%` operator. Each of these methods allows you to specify the desired precision, rounding behavior, and even the inclusion of commas for thousands, making it easier to present large numbers in a digestible format.
Moreover, Python’s built-in libraries, such as `decimal`, offer advanced capabilities for handling decimal arithmetic with high precision. This is particularly useful in scenarios where floating-point representation may lead to inaccuracies. By mastering the various techniques for formatting decimals, you can not only improve the aesthetic quality of your
Using the format() Function
The `format()` function in Python is a powerful way to format decimal numbers. It allows for extensive customization of how numbers are displayed, including the number of decimal places. The syntax for using `format()` is as follows:
“`python
formatted_number = “{:.nf}”.format(number)
“`
Here, `n` is the number of decimal places you want to display. For example:
“`python
number = 3.14159
formatted_number = “{:.2f}”.format(number) Result: 3.14
“`
This method is particularly useful for creating formatted strings for display purposes.
Using f-Strings
Since Python 3.6, f-strings have provided a concise way to embed expressions inside string literals. They are easy to read and can also be used to format decimals effectively. The syntax for f-strings is as follows:
“`python
formatted_number = f”{number:.nf}”
“`
For example:
“`python
number = 2.71828
formatted_number = f”{number:.3f}” Result: 2.718
“`
F-strings are not only more readable but also often faster than the traditional `format()` method.
Using the round() Function
The `round()` function can also be used to round a decimal to a specified number of places. However, it’s important to note that `round()` returns a float, which might not always display the desired number of decimal places in string representation.
“`python
rounded_number = round(number, n)
“`
For example:
“`python
number = 1.234567
rounded_number = round(number, 2) Result: 1.23
“`
While `round()` is straightforward, it does not format the number as a string, so for display purposes, you may still want to convert it back to a formatted string.
Formatting with the Percentage Symbol
When working with percentages, you can format decimal numbers by multiplying the number by 100 and appending a percentage sign. This can be done using either the `format()` function or f-strings. Here’s how to do it:
“`python
percentage = 0.12345
formatted_percentage = “{:.2%}”.format(percentage) Result: 12.35%
“`
Using f-strings:
“`python
formatted_percentage = f”{percentage:.2%}” Result: 12.35%
“`
This method automatically handles the conversion and formatting, providing a clear percentage representation.
Table of Formatting Options
The following table summarizes various formatting options for decimals in Python:
Method | Example Code | Result |
---|---|---|
format() | “{:.2f}”.format(3.14159) | 3.14 |
f-Strings | f”{2.71828:.3f}” | 2.718 |
round() | round(1.234567, 2) | 1.23 |
Percentage Formatting | “{:.2%}”.format(0.12345) | 12.35% |
Each method has its own advantages, and the choice of which to use will depend on the specific requirements of your application.
Using the Format Function
The `format()` method in Python provides a versatile way to format decimals. It can control the number of decimal places, padding, alignment, and more.
“`python
value = 3.14159
formatted_value = “{:.2f}”.format(value)
print(formatted_value) Output: 3.14
“`
In this example, the `.2f` specifies that the number should be formatted as a floating point with two decimal places. The following table illustrates various formatting options:
Format Specifier | Description | Example |
---|---|---|
`.2f` | Floating-point number (2 decimal places) | `”{:.2f}”.format(3.1)` |
`.1e` | Scientific notation (1 decimal) | `”{:.1e}”.format(1234)` |
`,.2f` | Comma as thousand separator, 2 decimal places | `”{:,.2f}”.format(1234567.89)` |
Using f-Strings
Introduced in Python 3.6, f-strings offer a more concise way to format strings, including decimals.
“`python
value = 3.14159
formatted_value = f”{value:.3f}”
print(formatted_value) Output: 3.142
“`
F-strings can also accommodate expressions directly within the braces, allowing for dynamic formatting:
“`python
value = 2.71828
precision = 4
formatted_value = f”{value:.{precision}f}”
print(formatted_value) Output: 2.7183
“`
Using the Decimal Module
For precise decimal arithmetic, especially in financial applications, the `decimal` module is preferable. This module avoids floating-point representation issues.
“`python
from decimal import Decimal, ROUND_HALF_UP
value = Decimal(‘3.14159’)
formatted_value = value.quantize(Decimal(‘0.01’), rounding=ROUND_HALF_UP)
print(formatted_value) Output: 3.14
“`
You can specify the precision by changing the `Decimal(‘0.01’)` to another value, as shown below:
Precision | Example |
---|---|
`Decimal(‘0.1’)` | `value.quantize(Decimal(‘0.1’))` |
`Decimal(‘0.001’)` | `value.quantize(Decimal(‘0.001’))` |
Using String Formatting Operators
The old-style string formatting uses the `%` operator, which is still supported but less preferred in modern Python.
“`python
value = 3.14159
formatted_value = “%.2f” % value
print(formatted_value) Output: 3.14
“`
This method allows for basic formatting options, but it is recommended to use `format()` or f-strings for more complex formatting needs.
Conclusion on Formatting Decimals
Selecting the appropriate method for formatting decimals in Python depends on the requirements of your project. The `format()` method, f-strings, and the `decimal` module each have distinct advantages and use cases.
Expert Insights on Formatting Decimals in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “When formatting decimals in Python, utilizing the built-in `format()` function or f-strings can significantly enhance readability. These methods allow for precise control over the number of decimal places, ensuring that your output meets both aesthetic and functional requirements.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “For financial applications, it is crucial to format decimals correctly to avoid rounding errors. The `decimal` module in Python provides a robust solution, allowing for fixed-point and floating-point arithmetic with user-defined precision, which is essential for accurate calculations.”
Sarah Lee (Python Programming Instructor, Code Academy). “Understanding the difference between string formatting methods in Python is vital for effective decimal formatting. While older methods like `%` formatting are still in use, transitioning to f-strings not only simplifies the syntax but also improves performance and clarity in your code.”
Frequently Asked Questions (FAQs)
How can I format a float to two decimal places in Python?
You can format a float to two decimal places using the `format()` function or f-strings. For example, `”{:.2f}”.format(value)` or `f”{value:.2f}”` will both yield a string representation of the float rounded to two decimal places.
What is the purpose of the round() function in Python?
The `round()` function in Python is used to round a floating-point number to a specified number of decimal places. For example, `round(value, 2)` will round the `value` to two decimal places.
How do I format decimals using the Decimal module?
The `Decimal` module allows for precise decimal arithmetic. You can format decimals by creating a `Decimal` object and using the `quantize()` method. For example, `decimal_value.quantize(Decimal(‘0.00’))` will format the decimal to two decimal places.
Can I use string interpolation for formatting decimals?
Yes, string interpolation can be used for formatting decimals. Using f-strings, you can format a decimal by writing `f”{value:.2f}”`, which formats the value to two decimal places directly within the string.
What is the difference between formatting with f-strings and the format() method?
F-strings are a more concise and readable way to format strings, introduced in Python 3.6. The `format()` method is more versatile and can be used with older versions of Python. Both achieve similar results, but f-strings are generally preferred for their simplicity.
How can I handle rounding errors when formatting decimals?
To handle rounding errors, use the `Decimal` module, which provides better precision for decimal arithmetic. Avoid using floating-point arithmetic for financial calculations, as it can lead to inaccuracies. Instead, use `Decimal` for precise control over rounding and formatting.
In Python, formatting decimals is an essential skill for presenting numerical data clearly and accurately. The language offers several methods to format decimal numbers, including the built-in `format()` function, f-strings (formatted string literals), and the `round()` function. Each method provides flexibility in specifying the number of decimal places, ensuring that the output meets the desired precision and presentation standards.
Using f-strings is one of the most modern and convenient approaches, allowing for inline expressions and straightforward syntax. For example, using `f”{value:.2f}”` formats the number to two decimal places. The `format()` function is also versatile, enabling more complex formatting options, such as padding and alignment. Additionally, the `round()` function can be used for rounding numbers to a specified number of decimal places, though it does not format the output as a string.
It is crucial to choose the appropriate formatting method based on the context of the data presentation. For instance, when displaying monetary values, ensuring consistent decimal places enhances readability and professionalism. Furthermore, understanding the implications of floating-point arithmetic in Python can help avoid common pitfalls associated with decimal representation.
mastering decimal formatting in Python not only improves the clarity of numerical
Author Profile
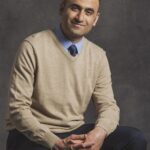
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?