How Can You Retrieve Input Values in JavaScript?
### Introduction
In the dynamic world of web development, understanding how to interact with user inputs is crucial for creating engaging and responsive applications. JavaScript, the backbone of modern web interactivity, provides developers with powerful tools to capture and manipulate data from various input elements. Whether you’re building a simple form or a complex user interface, knowing how to get the value from an input in JavaScript is a fundamental skill that can elevate your coding prowess and enhance user experience.
As you delve into the intricacies of JavaScript, you’ll discover that retrieving input values is not just about accessing data; it’s about creating a seamless flow of information between the user and the application. From text fields and checkboxes to dropdown menus, each input type has its own method for extraction, allowing you to tailor your approach based on the context of your project. Understanding these methods will empower you to validate user entries, trigger events, and ultimately, build more interactive web applications.
In this article, we will explore the various techniques for obtaining input values in JavaScript, equipping you with the knowledge to handle user data efficiently. Whether you are a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this guide will provide you with the insights needed to harness the full potential of user inputs
Accessing Input Values in JavaScript
To retrieve the value from an input element in JavaScript, you typically access the input field using the Document Object Model (DOM). The process involves selecting the input element and then using the `value` property to get its current value.
Here are the steps to get the value from an input element:
- Select the Input Element: Use methods like `document.getElementById()`, `document.querySelector()`, or any other DOM selection method to target the input field.
- Retrieve the Value: Once the element is selected, access the `value` property to obtain the data entered by the user.
Consider the following example:
In this example:
- An input field and a button are created.
- When the button is clicked, the `getInputValue` function is called, which retrieves the value from the input field and displays it in a paragraph.
Using Event Listeners for Input Retrieval
For more dynamic applications, especially when you want to respond to user input in real-time, using event listeners is beneficial. This allows you to capture input values as the user types.
In this example, the `oninput` event triggers the `updateValue` function every time the user types in the input field, providing immediate feedback.
Handling Different Input Types
The method of retrieving values can vary slightly depending on the type of input element. Below is a comparison of various input types and how to handle them.
Input Type | Value Retrieval Method |
---|---|
Text | inputElement.value |
Checkbox | inputElement.checked (returns true/) |
Radio | document.querySelector(‘input[name=”radioName”]:checked’).value |
Select (dropdown) | selectElement.value |
This table summarizes the appropriate methods to access values from different types of input fields, demonstrating the flexibility of JavaScript in handling various user inputs.
By understanding how to access input values effectively, developers can create more interactive and responsive web applications.
Accessing Input Values in JavaScript
To retrieve the value from an input element in JavaScript, you typically use the Document Object Model (DOM) to select the input and then access its value property. Here are the key steps involved:
- Select the Input Element: You can use methods like `getElementById`, `querySelector`, or other DOM selection methods to target the specific input element.
- Retrieve the Value: Once the input element is selected, access its `value` property to obtain the current value entered by the user.
### Example Code
In this example:
- An input element with the ID `myInput` is defined.
- A button triggers a function when clicked, which retrieves and logs the input value.
Using Query Selector
For more complex selectors or when working with multiple elements, `querySelector` can be particularly useful. It allows for CSS-style selectors to access elements.
### Example Code
This method is advantageous for targeting elements based on class names or other attributes, enhancing flexibility in your JavaScript code.
Handling Different Input Types
When working with various input types (e.g., text, checkbox, radio), the approach to retrieve values differs slightly. Below is a concise breakdown:
Input Type | Method to Retrieve Value |
---|---|
Text | `inputElement.value` |
Checkbox | `inputElement.checked` (returns boolean) |
Radio Button | `document.querySelector(‘input[name=”group”]:checked’).value` |
### Example for Checkbox
Check me
### Example for Radio Buttons
Option 1
Option 2
Best Practices for Input Handling
When working with input values in JavaScript, adhere to the following best practices:
- Validation: Always validate user input to avoid unexpected results or errors.
- Event Handling: Use event listeners to capture input changes or button clicks rather than inline event handlers for better separation of concerns.
- Accessibility: Ensure your input elements are accessible, using appropriate labels and ARIA attributes when necessary.
By following these guidelines, you can create a more robust and user-friendly experience within your web applications.
Expert Insights on Retrieving Input Values in JavaScript
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To effectively retrieve the value from an input element in JavaScript, one must utilize the Document Object Model (DOM) methods. The most common approach is to use `document.getElementById()` or `document.querySelector()` to access the input field and then extract its value using the `.value` property.”
Michael Thompson (JavaScript Specialist, CodeCraft Academy). “Understanding event handling is crucial when working with input values. By attaching an event listener, such as ‘change’ or ‘input’, you can dynamically capture the value as the user interacts with the input field, ensuring that you always have the most current data.”
Lisa Patel (Front-End Developer, Creative Solutions LLC). “It’s essential to validate the input value after retrieval. Using methods like `trim()` to remove unnecessary whitespace and implementing regular expressions can help ensure that the data collected from user inputs meets the required format before further processing.”
Frequently Asked Questions (FAQs)
How can I retrieve the value of an input field in JavaScript?
You can retrieve the value of an input field by using the `value` property of the input element. For example, if you have an input with the id `myInput`, you can access its value using `document.getElementById(‘myInput’).value`.
What is the difference between `getElementById` and `querySelector` for accessing input values?
`getElementById` retrieves an element by its unique ID, while `querySelector` can select elements using CSS selectors, allowing for more complex queries. Both can be used to access the `value` property of input fields.
Can I get the value of an input field on a button click?
Yes, you can retrieve the value of an input field when a button is clicked by adding an event listener to the button. Inside the event handler, access the input field’s value using its `value` property.
How do I handle input values from multiple fields?
To handle values from multiple input fields, you can use their respective IDs or classes to access each field’s value individually. Store these values in variables or an object for further processing.
Is it possible to get the value of an input field in a form submission event?
Yes, you can access input values during a form submission by using the `submit` event. In the event handler, prevent the default submission behavior and retrieve input values using their `value` properties.
What should I do if the input field is empty?
You can check if the input field is empty by evaluating its `value` property. If it is an empty string, you can handle it accordingly, such as displaying an error message or preventing form submission.
In JavaScript, obtaining the value from an input element is a fundamental task that can be accomplished using the Document Object Model (DOM). The most common method involves selecting the input element using methods such as `getElementById`, `querySelector`, or `getElementsByClassName`, and then accessing its `value` property. This straightforward approach allows developers to retrieve user input effectively for further processing or validation.
It is essential to consider the timing of when to access the input value. Typically, this is done in response to user actions, such as form submissions or button clicks. By attaching event listeners to these actions, developers can ensure they capture the most recent input from users. Additionally, it is crucial to handle input types appropriately, as different input types (e.g., text, checkbox, radio) may require specific methods to retrieve their values accurately.
Moreover, incorporating error handling and validation when retrieving input values enhances the robustness of the application. This practice ensures that the data collected is both valid and secure, ultimately leading to a better user experience. Overall, mastering the retrieval of input values in JavaScript is a foundational skill that supports a wide range of interactive web functionalities.
Author Profile
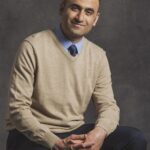
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?