How Can You Effectively Ignore Warnings in Python?
In the world of programming, warnings are like the caution lights on a dashboard—they signal potential issues that could lead to bigger problems down the road. For Python developers, these warnings can sometimes feel overwhelming, especially when they clutter the console and distract from the core functionality of the code. While it’s crucial to address genuine concerns, there are instances when developers may want to ignore these warnings to maintain focus or streamline their workflow. Whether you’re working on a legacy project, experimenting with new libraries, or simply trying to keep your output clean, knowing how to manage warnings effectively can enhance your coding experience.
Ignoring warnings in Python isn’t just about silencing the noise; it’s about understanding the context in which those warnings arise. Python’s built-in warning system is designed to alert developers to potential issues without halting execution, providing a balance between safety and functionality. However, there are scenarios where these warnings may not be relevant to your specific use case. By learning how to selectively suppress these messages, you can create a more efficient development environment that allows you to focus on the task at hand.
In this article, we will explore various methods to ignore warnings in Python, from using built-in libraries to customizing warning filters. Whether you’re a seasoned developer or a newcomer to the language, you’ll gain insights into how to
Using the `warnings` Module
The Python `warnings` module provides a way to issue warning messages to the user. However, there are situations where you may want to suppress these warnings. You can manage warnings using the functions provided by this module.
To ignore warnings, you can use the `filterwarnings` function. Here is how you can implement this:
“`python
import warnings
Ignore all warnings
warnings.filterwarnings(“ignore”)
“`
By setting the filter to `”ignore”`, all warnings will be suppressed. This approach can be useful in scenarios such as when you are working with legacy code or third-party libraries where warnings may not be relevant.
Ignoring Specific Warnings
If you only want to ignore specific warnings, you can specify the category of the warning to be ignored. The `warnings` module defines several warning categories, such as `DeprecationWarning`, `SyntaxWarning`, and `RuntimeWarning`.
Here is an example of how to ignore a specific warning:
“`python
import warnings
Ignore only DeprecationWarnings
warnings.filterwarnings(“ignore”, category=DeprecationWarning)
“`
This method allows you to maintain awareness of other warnings that may be critical for your application.
Context Managers for Warning Control
Python also provides context managers that allow you to ignore warnings temporarily within a specific block of code. This is particularly useful when you want to silence warnings during a certain operation without affecting the entire script.
Here’s how to use a context manager to ignore warnings:
“`python
import warnings
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
Code that may generate warnings
“`
By using `warnings.catch_warnings()`, you can ensure that only the warnings within the `with` block are ignored.
Table of Warning Control Methods
The following table summarizes the various methods to ignore warnings in Python:
Method | Description |
---|---|
Global Ignore | Suppresses all warnings throughout the script. |
Specific Ignore | Suppresses only specified categories of warnings. |
Context Manager | Suppresses warnings only within a specific block of code. |
Using these methods allows you to control the visibility of warnings, making your output cleaner and focusing on critical issues that may arise during code execution.
Using the warnings Module
In Python, the `warnings` module provides a robust framework for issuing and controlling warnings. To ignore specific warnings or all warnings, you can utilize the following methods:
- Ignore a Specific Warning: Use `warnings.filterwarnings()` to ignore a particular warning category.
“`python
import warnings
Ignore a specific warning
warnings.filterwarnings(“ignore”, category=UserWarning)
“`
- Ignore All Warnings: To suppress all warnings, you can set the filter to ignore everything.
“`python
import warnings
Ignore all warnings
warnings.filterwarnings(“ignore”)
“`
This method is beneficial for cleaning up output during development or when working with third-party libraries that may generate warnings you do not want to address immediately.
Using Context Managers
Context managers can be employed for temporary suppression of warnings in specific code blocks. The `warnings` module offers a context manager to achieve this.
“`python
import warnings
Ignore warnings temporarily in a specific block
with warnings.catch_warnings():
warnings.simplefilter(“ignore”)
Code that may generate warnings
“`
This approach is useful when you want to suppress warnings only in a specific section of your code without affecting the global warning filter.
Command Line Options
When running Python scripts, you can also control warning display through command-line options. The following flags can be used:
- -W ignore: Ignore all warnings.
- -W ignore::UserWarning: Ignore only UserWarnings.
Example usage in the command line:
“`bash
python -W ignore my_script.py
“`
This method is effective for quick suppression without modifying the script itself.
Using Environment Variables
Another way to ignore warnings globally is to set the `PYTHONWARNINGS` environment variable. This can be done in your shell or command prompt:
“`bash
export PYTHONWARNINGS=”ignore”
“`
For Windows:
“`cmd
set PYTHONWARNINGS=ignore
“`
This method will apply to all Python scripts executed in that environment, making it a broad solution for managing warnings.
Considerations When Ignoring Warnings
While ignoring warnings can be useful, it is essential to consider the implications:
- Risk of Missing Important Alerts: Ignoring warnings may lead to overlooking significant issues in your code.
- Code Quality and Maintenance: Suppressing warnings can affect code readability and maintainability, as developers may miss critical feedback from the interpreter.
Method | Scope | Use Case |
---|---|---|
`warnings.filterwarnings` | Global or specific | When you want to silence specific or all warnings. |
Context Manager | Specific code block | To suppress warnings in a controlled environment. |
Command Line Options | Global for script | Quick suppression for script execution. |
Environment Variables | Global for environment | Broad suppression across multiple scripts. |
By carefully managing warnings, you can maintain a clean output while also ensuring that important alerts are not overlooked.
Expert Strategies for Ignoring Warnings in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Ignoring warnings in Python can be essential for maintaining focus on critical issues. The use of the `warnings` module allows developers to filter out specific warnings or suppress them entirely using `warnings.filterwarnings(‘ignore’)`. This approach helps streamline the debugging process, especially in large codebases.”
Michael Chen (Lead Data Scientist, Tech Innovations Corp). “In data science projects, it is common to encounter warnings related to deprecated functions or libraries. While it is crucial to address these warnings eventually, using `with warnings.catch_warnings():` in conjunction with `warnings.simplefilter(‘ignore’)` can provide a temporary solution to keep the workflow uninterrupted during exploratory analysis.”
Sarah Thompson (Python Educator, Code Academy). “For beginners, it is important to understand that while ignoring warnings can be tempting, it is generally advisable to investigate their causes. However, if you must ignore them, using the `-W ignore` command line option when running scripts can effectively suppress all warnings, allowing for a cleaner output during development.”
Frequently Asked Questions (FAQs)
How can I ignore warnings in Python?
You can ignore warnings in Python by using the `warnings` module. Specifically, you can call `warnings.filterwarnings(‘ignore’)` to suppress all warnings.
Is it possible to ignore specific warnings in Python?
Yes, you can ignore specific warnings by using the `warnings.filterwarnings()` method with parameters that match the warning type you want to suppress. For example, `warnings.filterwarnings(‘ignore’, category=DeprecationWarning)` will ignore only deprecation warnings.
What is the effect of using `warnings.simplefilter(‘ignore’)`?
Using `warnings.simplefilter(‘ignore’)` sets the filter for all warnings to ignore, effectively silencing them. This method is a simpler alternative to `filterwarnings()` and is typically used for general suppression.
Can I suppress warnings in a specific context or function?
Yes, you can suppress warnings in a specific context by using the `warnings.catch_warnings()` context manager. This allows you to temporarily change the warning filter within a block of code.
Are there any risks associated with ignoring warnings in Python?
Ignoring warnings can lead to missing important information about potential issues in your code. It is advisable to address the root causes of warnings rather than suppressing them entirely.
How do I re-enable warnings after ignoring them?
To re-enable warnings after ignoring them, you can call `warnings.filterwarnings(‘default’)` or `warnings.simplefilter(‘always’)`, which will restore the default behavior for warnings in your code.
In Python, warnings are typically used to alert developers about potential issues in their code that may not necessarily raise an error but could lead to unexpected behavior. While it is generally advisable to address these warnings, there are scenarios where developers may want to suppress them, particularly during development or when working with legacy code. Python provides several methods to ignore warnings, including the use of the `warnings` module, which allows for fine-grained control over warning messages.
One common approach to ignore warnings is to use the `warnings.filterwarnings()` function. By setting the filter to ignore specific warnings or all warnings, developers can prevent them from cluttering the output. Additionally, the `warnings.simplefilter()` function can be employed to set a global filter for all warnings. It is also possible to suppress warnings temporarily using a context manager, which is particularly useful in scenarios where warnings are expected in a specific code block but should not affect the overall execution flow.
Key takeaways include the importance of understanding the implications of ignoring warnings. While suppressing warnings can enhance code readability and reduce distractions, it is crucial to evaluate the underlying issues that trigger these warnings. Ignoring warnings without addressing their root causes can lead to more significant problems down the line. Therefore,
Author Profile
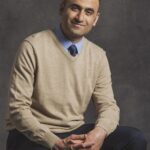
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?