How Can You Effectively Loop Through an Object in Python?
### Introduction
In the world of programming, the ability to manipulate and traverse data structures is essential for efficient coding and problem-solving. Python, with its elegant syntax and powerful data handling capabilities, offers a variety of ways to loop through objects, making it a favorite among developers. Whether you’re dealing with dictionaries, lists, or custom objects, understanding how to iterate through these structures can unlock new levels of productivity and creativity in your coding endeavors. In this article, we will explore the various methods to loop through objects in Python, equipping you with the tools to enhance your programming skills.
When working with Python, looping through objects is a fundamental skill that enables you to access and process data seamlessly. Each type of object, from lists to dictionaries, has its own unique characteristics and methods for iteration. By mastering these techniques, you can efficiently extract information, perform calculations, or even transform data as needed. The beauty of Python lies in its versatility, allowing you to choose the most appropriate looping construct for your specific use case.
As we delve deeper into the topic, we will examine the different looping mechanisms available in Python, including the classic `for` loop, the `while` loop, and the powerful list comprehensions. We will also touch on best practices and common pitfalls to avoid,
Looping Through an Object
In Python, looping through objects such as dictionaries and lists can be accomplished using various methods, each with its unique applications and advantages. Understanding these methods is essential for effective data manipulation and retrieval.
Using a For Loop
The most common way to loop through an object is by utilizing a `for` loop. This method is straightforward and works seamlessly with different types of iterable objects.
Example with a Dictionary:
python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key in my_dict:
print(f”Key: {key}, Value: {my_dict[key]}”)
In this example, the loop iterates over the keys of `my_dict`, allowing access to both keys and their corresponding values.
Iterating Over Keys and Values
To iterate over both keys and values simultaneously, Python provides the `.items()` method, which is particularly useful when you need both elements in the loop.
Example:
python
for key, value in my_dict.items():
print(f”Key: {key}, Value: {value}”)
This approach simplifies the process of handling key-value pairs without additional lookups.
Using List Comprehensions
List comprehensions offer a concise way to iterate through iterable objects and create new lists based on existing data. They are particularly useful when you want to apply a transformation or filter data.
Example:
python
squared_values = [value ** 2 for value in my_dict.values()]
This single line creates a new list containing the squares of the values from `my_dict`.
Iterating Over Lists
When working with lists, the iteration process is similar to dictionaries. You can loop through each item directly.
Example:
python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
Using Enumerate for Indexing
The `enumerate()` function is particularly useful when you need both the index and the value of elements in a list. This function returns pairs of index and value, making it easier to keep track of positions.
Example:
python
for index, value in enumerate(my_list):
print(f”Index: {index}, Value: {value}”)
Table of Looping Methods
Method | Use Case | Example |
---|---|---|
For Loop | Iterating over keys or elements | for key in my_dict |
Items() | Accessing key-value pairs in dictionaries | for key, value in my_dict.items() |
List Comprehension | Creating lists from existing data | [value ** 2 for value in my_dict.values()] |
Enumerate | Accessing index and value in lists | for index, value in enumerate(my_list) |
Utilizing these various methods for looping through objects enables developers to write cleaner and more efficient code, ultimately leading to better performance and maintainability.
Looping Through an Object in Python
In Python, the term “object” can refer to various data structures, such as dictionaries, lists, or even user-defined classes. Each type of object has its own method for iteration. Below are some common approaches to loop through different types of objects.
Looping Through a Dictionary
Dictionaries are collections of key-value pairs. You can loop through a dictionary in several ways:
- Looping Through Keys:
python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key in my_dict:
print(key)
- Looping Through Values:
python
for value in my_dict.values():
print(value)
- Looping Through Key-Value Pairs:
python
for key, value in my_dict.items():
print(f”{key}: {value}”)
Looping Through a List
Lists are ordered collections that can hold multiple items. You can loop through a list using:
- Standard Loop:
python
my_list = [1, 2, 3, 4]
for item in my_list:
print(item)
- Using Enumerate:
The `enumerate` function adds a counter to the loop.
python
for index, value in enumerate(my_list):
print(f”Index {index}: {value}”)
Looping Through a Set
Sets are unordered collections of unique items. Looping through a set is straightforward:
python
my_set = {1, 2, 3, 4}
for item in my_set:
print(item)
Looping Through a User-Defined Object
To loop through a user-defined object, you must define the `__iter__()` and `__next__()` methods in the class. Here’s an example:
python
class MyCollection:
def __init__(self):
self.items = [1, 2, 3]
def __iter__(self):
self.index = 0
return self
def __next__(self):
if self.index < len(self.items):
result = self.items[self.index]
self.index += 1
return result
else:
raise StopIteration
my_collection = MyCollection()
for item in my_collection:
print(item)
Using List Comprehensions
List comprehensions provide a concise way to create lists and can be used to loop through objects in a single line:
python
squared = [x**2 for x in range(10)]
print(squared)
This method is particularly useful for applying transformations or filtering data while iterating.
Python provides various mechanisms to loop through objects, enabling flexibility and efficiency in data handling. By selecting the appropriate method for the object type, you can effectively manage and manipulate data in your applications.
Expert Insights on Looping Through Objects in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When looping through objects in Python, it is essential to understand the data structure you are working with. Utilizing methods like `items()` for dictionaries or `values()` for lists can significantly enhance the clarity and efficiency of your code.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “I recommend using list comprehensions when looping through objects, as they not only simplify the syntax but also improve performance. This approach allows for cleaner and more readable code, which is crucial in collaborative environments.”
Lisa Chen (Data Scientist, Analytics Hub). “For complex objects, leveraging the `for` loop with custom methods can provide greater flexibility. It is important to ensure that your objects are iterable, and using Python’s built-in functions can help in efficiently accessing and manipulating data.”
Frequently Asked Questions (FAQs)
How do I loop through an object in Python?
You can loop through an object in Python using a `for` loop. For example, if the object is a dictionary, you can iterate over its keys or values using `for key in my_dict` or `for value in my_dict.values()`.
What types of objects can be looped through in Python?
In Python, you can loop through various iterable objects, including lists, tuples, dictionaries, sets, and strings. Each of these supports iteration using a `for` loop.
Can I use the `enumerate()` function while looping through an object?
Yes, the `enumerate()` function can be used to loop through an iterable while keeping track of the index. This is particularly useful for lists and tuples, allowing you to access both the index and the value during iteration.
What is the difference between looping through keys and values in a dictionary?
Looping through keys retrieves the dictionary’s keys, while looping through values retrieves the corresponding values. You can use `my_dict.keys()` for keys and `my_dict.values()` for values.
How can I loop through a list of objects in Python?
You can loop through a list of objects using a `for` loop. For example, `for obj in my_list:` allows you to access each object in the list sequentially.
Is it possible to loop through a class instance’s attributes in Python?
Yes, you can loop through a class instance’s attributes using the `vars()` function or the `__dict__` attribute. For example, `for attr, value in vars(my_instance).items():` iterates through all attributes and their values.
Looping through an object in Python is a fundamental skill that allows developers to efficiently access and manipulate data stored within various data structures. Objects in Python, such as dictionaries, lists, and sets, can be iterated over using different methods, including for loops and comprehensions. Understanding how to traverse these objects is essential for performing operations like data retrieval, transformation, and analysis.
One of the most common ways to loop through a dictionary is by using the items() method, which provides both keys and values. In contrast, lists can be iterated over directly, allowing access to each element in sequence. Additionally, the use of comprehensions offers a concise and readable way to create new lists or dictionaries based on existing ones. Mastery of these techniques can significantly enhance the efficiency and clarity of your code.
In summary, effectively looping through objects in Python is crucial for data manipulation and processing tasks. By utilizing built-in methods and comprehensions, developers can write cleaner and more efficient code. A solid grasp of these concepts not only improves programming skills but also contributes to better software development practices overall.
Author Profile
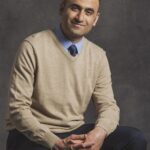
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?