How Do You Push Your Node.js Project to GitHub?
In the ever-evolving landscape of web development, sharing your work and collaborating with others has never been more crucial. For developers using Node.js, a powerful JavaScript runtime, pushing your project to GitHub not only showcases your skills but also opens the door to community feedback and contributions. Whether you’re working on a personal project or a collaborative endeavor, understanding how to effectively push your Node.js application to GitHub can significantly enhance your development workflow and visibility in the tech community.
Getting your Node.js project onto GitHub involves a series of straightforward steps that integrate version control with your development process. First, you’ll need to ensure your project is properly set up with Git, which serves as the backbone for tracking changes and managing your codebase. Once your local repository is configured, the process of pushing your project to GitHub becomes a seamless experience that allows you to maintain a backup of your work while also sharing it with others.
As you embark on this journey, it’s essential to familiarize yourself with the GitHub interface and commands that will facilitate this transfer. From initializing your repository to committing changes and pushing your code, each step builds upon the last to create a cohesive workflow. By mastering these techniques, you’ll not only enhance your own coding practices but also contribute to a vibrant community of developers eager to
Setting Up Your Local Git Repository
To begin pushing your Node.js project to GitHub, you first need to set up a Git repository locally. If you haven’t already initialized your project as a Git repository, follow these steps:
- Navigate to your project directory using the terminal.
- Initialize a Git repository by running the command:
“`
git init
“`
- Add your project files to the staging area:
“`
git add .
“`
- Commit the changes with a descriptive message:
“`
git commit -m “Initial commit”
“`
By completing these steps, you have created a local Git repository and made your first commit.
Creating a GitHub Repository
Next, you need to create a repository on GitHub where you can push your local project. Follow these steps:
- Log in to your GitHub account.
- Click the “+” icon in the top right corner and select “New repository.”
- Fill in the repository name, description, and choose whether to make it public or private.
- Click the “Create repository” button.
After creating the repository, GitHub will provide you with a URL that you will use to link your local repository to the remote one.
Linking Your Local Repository to GitHub
Once you have your GitHub repository set up, you need to link it to your local repository. Use the following command in your terminal:
“`
git remote add origin
“`
Replace `
“`
git remote -v
“`
This command will display the remote repositories linked to your local project.
Pushing Your Changes to GitHub
After linking your local repository to GitHub, you can push your committed changes. Use the following command to push the changes to the main branch:
“`
git push -u origin main
“`
If your default branch is named differently (like `master`), make sure to replace `main` with your branch name. The `-u` flag sets the upstream tracking for your branch, making future pushes easier.
Managing Future Changes
As you continue to work on your Node.js project, it’s essential to manage your commits and pushes effectively. Here’s a simple workflow:
- Make changes to your project files.
- Add the changes to the staging area:
“`
git add .
“`
- Commit the changes with a clear message:
“`
git commit -m “Description of changes”
“`
- Push the changes to GitHub:
“`
git push
“`
This workflow ensures your GitHub repository stays updated with your latest project developments.
Common Git Commands for Node.js Projects
Here is a table summarizing some common Git commands that can be useful for managing your Node.js project:
Command | Description |
---|---|
git init | Initialize a new Git repository. |
git add . | Add all changes to the staging area. |
git commit -m “message” | Commit staged changes with a message. |
git push -u origin main | Push changes to the remote repository. |
git status | Check the status of your working directory. |
git log | View the commit history. |
This table provides a quick reference to essential commands that will help you effectively manage your Node.js project with Git.
Setting Up a GitHub Repository
To push your Node.js project to GitHub, you first need to create a repository on GitHub. Follow these steps:
- Log in to your GitHub account.
- Click on the “+” icon in the top right corner and select “New repository.”
- Fill out the repository details:
- Repository name: Choose a unique name for your project.
- Description: Optionally, add a description of your project.
- Public/Private: Decide whether your repository will be public or private.
- Optionally, choose to initialize the repository with a README, .gitignore, or license file.
- Click on “Create repository.”
Preparing Your Local Node.js Project
Ensure your Node.js project is ready for version control. Here are the essential steps:
- Navigate to your project directory using the terminal:
“`bash
cd path/to/your/project
“`
- Initialize Git in your project directory if you haven’t already:
“`bash
git init
“`
- Create a `.gitignore` file to exclude files and directories that shouldn’t be tracked, such as `node_modules`:
“`bash
echo “node_modules/” >> .gitignore
“`
Committing Your Code
After initializing Git and preparing your project, commit your code using the following commands:
- Stage your files for commit:
“`bash
git add .
“`
- Commit the staged files with a meaningful message:
“`bash
git commit -m “Initial commit”
“`
Linking Local Repository to GitHub
Next, link your local repository to the GitHub repository you created:
- Copy the repository URL from GitHub (either HTTPS or SSH).
- Add the remote repository to your local Git configuration:
“`bash
git remote add origin
“`
Pushing Your Project to GitHub
Finally, push your changes to the GitHub repository:
- Push your local commits to the remote repository:
“`bash
git push -u origin main
“`
- Replace `main` with `master` if your default branch is named differently.
Verifying Your Push
To ensure that your project has been successfully pushed:
- Navigate to your GitHub repository in a web browser.
- Check that all your files and folders appear as expected.
- Review the commit history to confirm your latest commit is listed.
Managing Future Changes
For ongoing development, follow this workflow:
- Make changes to your project.
- Use `git add .` to stage changes.
- Commit with `git commit -m “Your message”`.
- Push changes using `git push origin main`.
This structured approach ensures your Node.js project remains well-organized and version-controlled on GitHub.
Expert Insights on Pushing Node.js Projects to GitHub
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To effectively push a Node.js project to GitHub, it is crucial to first initialize a Git repository in your project directory using ‘git init’. After staging your files with ‘git add .’, make sure to commit your changes with a descriptive message. Finally, link your local repository to the remote GitHub repository using ‘git remote add origin [repository URL]’ and push your changes with ‘git push -u origin main’.”
Michael Thompson (DevOps Specialist, Cloud Solutions Group). “When pushing a Node.js project to GitHub, it is essential to include a .gitignore file to prevent unnecessary files, such as node_modules, from being tracked. This keeps your repository clean and ensures that only relevant code is shared. Additionally, using branches for feature development can help maintain a stable main branch.”
Sarah Patel (Open Source Advocate, Code for Change). “Before pushing your Node.js project to GitHub, ensure that your code is well-documented and follows best practices. This not only aids in collaboration but also enhances the project’s visibility within the developer community. Consider including a README.md file that outlines the project’s purpose, installation instructions, and usage examples.”
Frequently Asked Questions (FAQs)
How do I initialize a Node.js project for GitHub?
To initialize a Node.js project for GitHub, create a new directory for your project, navigate into it using the command line, and run `npm init` to generate a `package.json` file. This file will manage your project’s dependencies and metadata.
What files should I include in my Node.js project when pushing to GitHub?
You should include all relevant source code files, configuration files, and the `package.json` file. Additionally, consider adding a `.gitignore` file to exclude files and directories that should not be tracked, such as `node_modules` and environment variables.
How do I create a new Git repository for my Node.js project?
To create a new Git repository, navigate to your project directory in the command line and run `git init`. This command initializes a new Git repository, allowing you to track changes and push your project to GitHub.
What commands do I use to push my Node.js project to GitHub?
After initializing your Git repository and adding your files using `git add .`, commit your changes with `git commit -m “Initial commit”`. Then, link your local repository to GitHub using `git remote add origin
How do I handle authentication when pushing to GitHub?
When pushing to GitHub, you may be prompted for your GitHub username and password. For enhanced security, consider using SSH keys or a personal access token instead of your password, especially if you have two-factor authentication enabled.
Can I update my Node.js project on GitHub after the initial push?
Yes, you can update your Node.js project on GitHub. Make changes to your local files, stage them using `git add .`, commit the changes with `git commit -m “Your message”`, and push the updates using `git push origin master`.
In summary, pushing a Node.js project to GitHub involves several key steps that ensure your code is properly managed and shared. First, you need to initialize a Git repository in your project directory if you haven’t done so already. This is accomplished using the command `git init`, which sets up the necessary files for version control. Following this, you should add your project files to the staging area with `git add .`, which prepares them for committing.
Once your files are staged, the next step is to commit your changes with a meaningful message using `git commit -m “Your commit message”`. This action saves your changes in the local repository. After committing, you must link your local repository to a remote GitHub repository using the command `git remote add origin
Finally, you can push your local commits to the remote repository with the command `git push -u origin main` (or `master`, depending on your branch naming). This command uploads your code to GitHub, making it accessible to others and allowing for collaborative development. By following these steps, you ensure that your Node.js project is effectively version
Author Profile
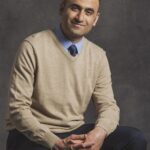
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?