How Can You Effectively Remove Specific Text from a File?
In the digital age, managing and manipulating text files is a fundamental skill that can save you time and enhance your productivity. Whether you’re a programmer, a data analyst, or simply someone who frequently works with documents, knowing how to remove specific text from a file can be invaluable. Imagine having a lengthy report filled with outdated information or a code file cluttered with unnecessary comments; the ability to efficiently clean up these files can streamline your workflow and improve clarity. In this article, we will explore various methods and tools that can help you effortlessly eliminate unwanted text, allowing you to focus on what truly matters.
Removing specific text from a file may seem like a daunting task, but it can be accomplished through a variety of techniques tailored to your needs. Depending on the complexity of the text you wish to remove and the tools at your disposal, you can choose from simple manual edits to more advanced automated scripts. Each method has its own advantages, whether it be ease of use, efficiency, or precision, and understanding these options will empower you to select the best approach for your situation.
As we delve deeper into the topic, we will cover the most common methods for text removal, including the use of text editors, command-line tools, and programming languages. We will also discuss best practices to ensure that
Using Text Editors
Text editors are an effective way to manually remove specific text from a file. Most text editors, such as Notepad++, Sublime Text, or Visual Studio Code, offer powerful find-and-replace functionality. Here’s how to do it:
- Open the file in your preferred text editor.
- Use the “Find” feature, usually accessible via Ctrl + F (or Command + F on Mac).
- Enter the specific text you wish to remove.
- Navigate to the “Replace” feature, often found as a separate option or within the Find dialog (Ctrl + H).
- Leave the “Replace with” field empty to remove the text.
- Click “Replace All” to remove all instances of the specified text throughout the document.
This method is straightforward for files that are not excessively large or complex.
Command Line Tools
For users comfortable with command line interfaces, tools like `sed` or `awk` can be used to remove specific text efficiently. These commands are particularly useful for processing large files or automating the task across multiple files.
– **Using `sed`:**
The `sed` command stream editor can remove text directly from files. The syntax is as follows:
“`bash
sed -i ‘s/text_to_remove//g’ filename
“`
- The `-i` option edits the file in place.
- The `s/old/new/g` syntax specifies substitution; `old` is the text to remove, and `new` is left empty.
– **Using `awk`:**
`awk` can also be used for more complex patterns. Here’s a basic example:
“`bash
awk ‘{gsub(/text_to_remove/, “”); print}’ filename > newfile
“`
This command creates a new file without the specified text.
Programming Approaches
For developers or those familiar with programming, writing a script in languages like Python can provide more control over text removal, especially for files with specific formatting. Here’s a simple Python example:
“`python
with open(‘filename.txt’, ‘r’) as file:
data = file.read()
data = data.replace(‘text_to_remove’, ”)
with open(‘filename.txt’, ‘w’) as file:
file.write(data)
“`
This script reads the file, replaces the specified text, and writes the result back to the same file.
Considerations When Removing Text
When removing specific text from files, consider the following:
- Backup: Always back up your files before performing bulk edits.
- Context: Ensure the text to be removed is not part of other important data.
- Encoding: Be aware of the file encoding, as it may affect text processing.
Comparison of Methods
Here’s a table summarizing the different methods for removing text from files:
Method | Ease of Use | Best For |
---|---|---|
Text Editors | Easy | Small to Medium Files |
Command Line Tools | Moderate | Large Files, Automation |
Programming Approaches | Advanced | Complex Tasks, Custom Requirements |
Selecting the appropriate method depends on your specific needs, file size, and comfort level with technology.
Methods to Remove Specific Text from a File
Removing specific text from a file can be achieved through various methods, depending on the tools and programming languages available. Below are some common approaches.
Using Text Editors
Most text editors provide a simple find-and-replace functionality that allows users to remove specific text. Here’s how to do it in popular text editors:
- Notepad++
- Open the file in Notepad++.
- Press `Ctrl + H` to open the Replace dialog.
- Enter the text you want to remove in the “Find what” box.
- Leave the “Replace with” box empty.
- Click “Replace All” to remove all instances.
- Sublime Text
- Open your file.
- Press `Ctrl + H` to bring up the Replace panel.
- Input the text to remove in the “Find” field.
- Leave the “Replace” field empty.
- Click “Replace All”.
- Visual Studio Code
- Open the file in Visual Studio Code.
- Press `Ctrl + H`.
- Enter the text you want to remove in the first box.
- Leave the second box empty.
- Click on the “Replace All” button.
Using Command Line Tools
Command line tools are efficient for batch processing and automating tasks. Below are methods using `sed` and `awk`, two common command-line utilities.
Using `sed`
The `sed` command is a stream editor that can modify files in place. To remove specific text, use the following syntax:
“`bash
sed -i ‘s/text_to_remove//g’ filename
“`
- `-i`: Edit the file in place.
- `s`: Substitute command.
- `g`: Global replacement (all occurrences).
Using `awk`
The `awk` command is another powerful text processing tool. To remove specific text, use:
“`bash
awk ‘{gsub(/text_to_remove/, “”); print}’ filename > newfile
“`
- `gsub`: Global substitution.
- The output will be saved to `newfile`.
Using Programming Languages
Various programming languages can also be utilized to remove specific text from files.
Python
Python provides a straightforward way to manipulate text files. Below is a sample code snippet:
“`python
with open(‘filename.txt’, ‘r’) as file:
data = file.read()
data = data.replace(‘text_to_remove’, ”)
with open(‘filename.txt’, ‘w’) as file:
file.write(data)
“`
JavaScript (Node.js)
For those using Node.js, the following code demonstrates how to remove specific text:
“`javascript
const fs = require(‘fs’);
fs.readFile(‘filename.txt’, ‘utf8’, (err, data) => {
if (err) throw err;
const result = data.replace(/text_to_remove/g, ”);
fs.writeFile(‘filename.txt’, result, ‘utf8’, (err) => {
if (err) throw err;
});
});
“`
Using Regex for Complex Patterns
For more complex text removal, regular expressions (Regex) can be employed. Most programming languages and text editors support Regex.
- Regex Example: To remove any number of digits:
- Pattern: `\d+`
In Python, you could use:
“`python
import re
with open(‘filename.txt’, ‘r’) as file:
data = file.read()
data = re.sub(r’\d+’, ”, data)
with open(‘filename.txt’, ‘w’) as file:
file.write(data)
“`
By utilizing the appropriate method based on your environment and requirements, you can effectively remove specific text from files with minimal effort.
Expert Insights on Removing Specific Text from Files
Emily Carter (Senior Software Developer, CodeCraft Solutions). “When removing specific text from a file, I recommend using regular expressions for precision. This method allows you to define patterns that match the text you want to eliminate, making it highly effective for complex scenarios.”
James Liu (Data Management Specialist, InfoSecure Inc.). “Utilizing scripting languages like Python can streamline the process of text removal. Libraries such as `re` for regex operations can be particularly powerful, enabling batch processing of multiple files with minimal effort.”
Sarah Thompson (IT Consultant, TechWise Advisors). “For users who prefer graphical interfaces, text editors like Notepad++ offer find-and-replace functionalities that can effectively remove specific text. This method is user-friendly and suitable for those less familiar with coding.”
Frequently Asked Questions (FAQs)
How can I remove specific text from a file using a text editor?
You can open the file in a text editor, use the “Find” feature to locate the specific text, and then delete it manually or use the “Replace” function to replace it with an empty string.
Is there a command-line method to remove specific text from a file?
Yes, you can use command-line tools such as `sed` or `awk`. For example, the command `sed -i ‘s/specific_text//g’ filename.txt` will remove all instances of “specific_text” from the specified file.
Can I remove specific text from a file using a programming language?
Yes, many programming languages provide file handling capabilities. For instance, in Python, you can read the file, replace the specific text using the `str.replace()` method, and then write the changes back to the file.
Are there any software tools available for batch removal of text from multiple files?
Yes, there are various tools such as Notepad++, Sublime Text, and specialized batch processing software that can perform find-and-replace operations across multiple files simultaneously.
What precautions should I take before removing text from a file?
Always create a backup of the original file before making changes. This ensures that you can restore the file if the removal process does not yield the desired results.
Can I undo the removal of text if I make a mistake?
If you are using a text editor, you can typically use the “Undo” feature (often Ctrl+Z) immediately after the change. However, if you have saved the file after the removal, you may need to restore from a backup.
In summary, removing specific text from a file can be accomplished through various methods, depending on the tools and programming languages available to the user. Common approaches include using text editors, command-line utilities, and programming scripts. Each method has its own set of advantages, such as ease of use, flexibility, and automation capabilities, which can significantly enhance productivity when dealing with large volumes of text.
Utilizing text editors like Notepad++ or Sublime Text allows for quick manual removal of text through search and replace functionalities. For more automated solutions, command-line tools such as `sed` or `awk` can be employed, providing powerful options for batch processing. Additionally, programming languages like Python or Perl offer robust libraries and functions that facilitate the removal of specific text patterns, making them suitable for more complex tasks.
Key takeaways include recognizing the importance of selecting the right tool for the task at hand. Users should consider factors such as the size of the file, the complexity of the text to be removed, and their own familiarity with the tools available. By understanding the different methods and their applications, users can efficiently manage and manipulate text files, ultimately saving time and improving workflow.
Author Profile
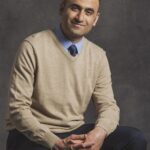
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?