How Can You Check the Version of a Kubernetes Node Using Go?
In the ever-evolving landscape of cloud-native technologies, Kubernetes stands out as a powerhouse for orchestrating containerized applications. As organizations increasingly rely on Kubernetes for their deployment strategies, understanding its components becomes crucial. One essential aspect is knowing the version of the Kubernetes node you are working with, especially when ensuring compatibility and leveraging new features. For developers and system administrators alike, being able to programmatically retrieve this information using Go can streamline workflows and enhance automation.
In this article, we’ll explore the process of determining the version of a Kubernetes node through Go programming. We’ll delve into the significance of this task within the broader context of Kubernetes management and discuss the tools and libraries that facilitate this interaction. By leveraging Go’s capabilities, you can efficiently access node information, which is vital for maintaining a robust and up-to-date Kubernetes environment.
Whether you’re a seasoned Go developer or just starting to explore Kubernetes, understanding how to fetch node version details can empower you to make informed decisions about your cluster’s health and performance. Join us as we unpack the essential steps and best practices for achieving this goal, setting the stage for a deeper dive into practical implementations and code examples.
Accessing Kubernetes Node Version in Go
To determine the version of a Kubernetes node using Go, you can leverage the Kubernetes client-go library, which provides the necessary abstractions to interact with the Kubernetes API. The process typically involves creating a client set, fetching node information, and extracting the version from the node’s status.
Setting Up the Environment
Before you can interact with the Kubernetes API, ensure that you have the following prerequisites:
- Go installed (version 1.15 or later)
- Access to a Kubernetes cluster
- The client-go library
To install the client-go library, use the following command:
“`bash
go get k8s.io/client-go@latest
“`
Code Example
The following Go code illustrates how to retrieve the Kubernetes version for each node in the cluster:
“`go
package main
import (
“context”
“fmt”
“os”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
)
func main() {
// Load kubeconfig
kubeconfig := os.Getenv(“KUBECONFIG”)
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
panic(err.Error())
}
// Create a clientset
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err.Error())
}
// Get nodes
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
panic(err.Error())
}
// Display node versions
for _, node := range nodes.Items {
fmt.Printf(“Node Name: %s, Kubernetes Version: %s\n”, node.Name, node.Status.NodeInfo.KubeletVersion)
}
}
“`
Explanation of the Code
- Importing Packages: The code imports necessary packages from the Kubernetes client-go and the standard library.
- Loading kubeconfig: It retrieves the kubeconfig file, which contains the cluster connection details.
- Creating a Clientset: Using the loaded configuration, it creates a clientset to interact with the Kubernetes API.
- Fetching Node List: It retrieves a list of nodes using the CoreV1 interface.
- Outputting Node Versions: Finally, it iterates through the nodes and prints each node’s name along with its Kubernetes version.
Understanding Node Versioning
Kubernetes versions are important for compatibility and feature usage. Each node’s version can be found in the `Status.NodeInfo.KubeletVersion` field, which indicates the version of the Kubelet running on that node. The output will typically look like this:
Node Name | Kubernetes Version |
---|---|
node1.example.com | v1.22.0 |
node2.example.com | v1.22.0 |
node3.example.com | v1.21.5 |
By keeping track of node versions, administrators can ensure that all nodes are running compatible versions, which is crucial for maintaining the stability and functionality of the cluster.
Using the client-go library, you can effectively access and manage Kubernetes resources, including obtaining node version information. This capability is essential for monitoring and ensuring the health of your Kubernetes environment.
Accessing Kubernetes Node Version Using Go
To retrieve the version of a Kubernetes node using Go, you will need to leverage the Kubernetes client-go library. This library provides a set of tools for interacting with Kubernetes clusters programmatically.
Setting Up Your Go Environment
Ensure that you have Go installed and set up. Additionally, you will need to install the client-go library. You can do this by running the following command:
“`bash
go get k8s.io/client-go@latest
“`
You may also need to include the `k8s.io/apimachinery` package:
“`bash
go get k8s.io/apimachinery@latest
“`
Writing the Code
The following code snippet demonstrates how to get the Kubernetes node version.
“`go
package main
import (
“context”
“fmt”
“os”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
func main() {
// Load Kubernetes configuration
kubeconfig := os.Getenv(“KUBECONFIG”)
if kubeconfig == “” {
kubeconfig = “/path/to/your/kubeconfig”
}
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
panic(err.Error())
}
// Create a Kubernetes client
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err.Error())
}
// Retrieve nodes
nodes, err := clientset.CoreV1().Nodes().List(context.Background(), metav1.ListOptions{})
if err != nil {
panic(err.Error())
}
// Iterate through nodes and print their versions
for _, node := range nodes.Items {
fmt.Printf(“Node Name: %s, Version: %s\n”, node.Name, node.Status.NodeInfo.KubeletVersion)
}
}
“`
Code Explanation
- Kubeconfig Setup: The code retrieves the Kubernetes configuration from the environment variable `KUBECONFIG`. If not set, it defaults to a specified path.
- Client Initialization: A Kubernetes client is created using the configuration.
- Node Retrieval: The nodes are fetched from the cluster using `clientset.CoreV1().Nodes().List`.
- Version Display: The version of each node is printed by accessing `node.Status.NodeInfo.KubeletVersion`.
Running the Application
To run the application, ensure your environment is properly set up with access to the Kubernetes cluster. Execute the following command in the terminal:
“`bash
go run yourfilename.go
“`
Replace `yourfilename.go` with the name of your Go file containing the above code. Ensure that your kubeconfig path is correctly specified if not using the environment variable.
Handling Errors
It is crucial to handle potential errors during the execution of your program. Some common errors to check for include:
- Configuration Errors: Ensure the kubeconfig path is correct.
- Connection Issues: Verify that you have network access to the Kubernetes API server.
- Insufficient Permissions: Ensure that your user has permission to list nodes.
You can enhance the error handling in the code by adding specific messages or handling different types of errors more gracefully.
This approach allows you to efficiently retrieve and display the versions of Kubernetes nodes using the Go programming language.
Expert Insights on Retrieving Kubernetes Node Versions Using Go
Dr. Emily Chen (Senior Software Engineer, Cloud Solutions Inc.). “To effectively retrieve the version of a Kubernetes node using Go, developers should leverage the client-go library. This library provides a robust interface to interact with Kubernetes APIs, allowing for seamless retrieval of node details, including their versions.”
Mark Thompson (Kubernetes Consultant, DevOps Masters). “Utilizing the Kubernetes API to fetch node information is essential. By crafting a simple Go application that queries the ‘/api/v1/nodes’ endpoint, developers can extract the node version information efficiently. Proper error handling and context management are crucial in this process.”
Linda Garcia (Cloud Infrastructure Architect, Tech Innovations). “When working with Go to access Kubernetes node versions, it is important to authenticate correctly with the cluster. Using service accounts and ensuring that your Go application has the necessary permissions can streamline the process and enhance security.”
Frequently Asked Questions (FAQs)
How can I check the Kubernetes node version using Go?
You can check the Kubernetes node version by using the Kubernetes client-go library. First, create a Kubernetes client and then retrieve the node object. The node object contains the version information in the `status.nodeInfo` field.
What Go packages are necessary to interact with Kubernetes nodes?
You will need the `k8s.io/client-go` package to create a client and interact with the Kubernetes API. Additionally, `k8s.io/api/core/v1` is required to work with node resources.
Is there a specific API endpoint to get the node version?
Yes, you can use the Kubernetes API endpoint `/api/v1/nodes/{nodeName}` to retrieve details about a specific node, including its version.
Can I get the version of all nodes in a cluster using Go?
Yes, you can list all nodes in the cluster using the `CoreV1().Nodes().List()` method from the client-go library, and then iterate through the nodes to extract their version information.
What is the structure of the node version information in Go?
The version information is found in the `NodeStatus` struct under `NodeInfo`, specifically in the `KubeletVersion` and `KubeProxyVersion` fields, which provide the respective versions of Kubelet and Kube Proxy.
Are there any common errors to watch for when retrieving node versions?
Common errors include authentication issues, network connectivity problems, or misconfigured Kubernetes client settings. Ensure that your client has the correct permissions and is configured to communicate with the Kubernetes API server.
To determine the version of a Kubernetes node using Go, developers can leverage the Kubernetes client-go library, which provides a robust set of tools for interacting with Kubernetes clusters. By utilizing the API provided by this library, one can easily query the node’s status and retrieve its version information. This process typically involves setting up a Kubernetes client, accessing the node resources, and extracting the relevant version details from the node’s metadata.
One of the key insights from this approach is the importance of understanding the structure of Kubernetes objects. Each node in a Kubernetes cluster contains a wealth of information, including its version, which is encapsulated in the NodeStatus. Familiarity with the Kubernetes API and its resource hierarchy is essential for efficiently retrieving this data. Additionally, error handling and authentication are critical components when working with the Kubernetes API, ensuring that the application can access the necessary resources securely.
Furthermore, this method demonstrates the power of Go in cloud-native environments. The language’s concurrency model and performance characteristics make it an excellent choice for developing tools that interact with Kubernetes. By mastering the client-go library and its functionalities, developers can create powerful applications that monitor and manage Kubernetes clusters effectively.
Author Profile
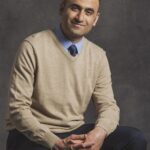
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?