How Can You Write -x in Python? A Step-by-Step Guide
When diving into the world of Python programming, one of the fundamental concepts that often arises is the manipulation of numbers and expressions. Among these operations, writing negative values or performing negation, such as `-x`, is a common requirement. Whether you’re developing a complex algorithm or simply performing basic arithmetic, understanding how to effectively represent and utilize negative numbers in Python can significantly enhance your coding skills. This article will guide you through the nuances of writing `-x` in Python, providing clarity and insight into its applications and implications.
In Python, the expression `-x` serves as a straightforward yet powerful tool for negating values. This simple operation can be applied to integers, floats, and even variables, allowing developers to easily switch the sign of a number. However, the implications of using negative values extend beyond mere arithmetic; they can influence control flow, data structures, and even mathematical functions. As we explore this topic, we’ll uncover the syntax and best practices for implementing negation in various contexts, ensuring you have a solid grasp of how to leverage this functionality in your own projects.
Moreover, understanding how to write `-x` is just the tip of the iceberg. The concept of negation can be intertwined with other programming constructs, such as loops, conditionals, and
Understanding Negation in Python
In Python, negation or the mathematical operation of subtracting a variable from zero can be achieved using the unary minus operator. When you write `-x`, Python interprets this as the negation of the value of `x`.
Examples of Using -x
To illustrate how to write `-x` in Python, consider the following examples:
“`python
x = 5
neg_x = -x neg_x will be -5
print(neg_x)
“`
In the example above, `x` is assigned the value of `5`, and then `-x` negates this value, resulting in `neg_x` being `-5`. The `print` statement outputs `-5` to the console.
Working with Different Data Types
The unary minus operator can be applied to various numerical data types in Python, such as integers and floats. Below are examples demonstrating its usage across different types:
- Integer:
“`python
x = 10
print(-x) Output: -10
“`
- Float:
“`python
x = 3.14
print(-x) Output: -3.14
“`
- Complex Numbers:
“`python
x = 2 + 3j
print(-x) Output: -2 – 3j
“`
Table: Negation of Various Data Types
Data Type | Value | Negated Value |
---|---|---|
Integer | 10 | -10 |
Float | 3.14 | -3.14 |
Complex | 2 + 3j | -2 – 3j |
Using Negation in Expressions
Negation can also be utilized within more complex expressions. For example, you might want to subtract a variable from another or apply negation to a calculation:
“`python
a = 10
b = 5
result = a – b result is 5
neg_result = -result neg_result is -5
print(neg_result)
“`
In this code snippet, `result` computes the difference between `a` and `b`, which is then negated to yield `neg_result`.
Common Use Cases for -x
The negation operator is commonly used in various programming scenarios, including:
- Mathematical calculations: When performing arithmetic operations where negative values are needed.
- Data manipulation: In data analysis, negating values can be useful for transformations or adjustments.
- Conditional logic: In control flow statements, negated values can influence the execution path based on conditions.
By mastering the use of `-x` in Python, developers can effectively manage numerical data and enhance their coding capabilities.
Understanding Negative Values in Python
In Python, the representation of negative values can be achieved through the unary negation operator (`-`). This operator is versatile and can be applied to various data types, including integers, floats, and even complex numbers.
Using the Negation Operator
To write a negative value in Python, you simply place the `-` sign before the number. Below are examples demonstrating how to use this operator:
“`python
Negating integers
a = -5
b = -10
Negating floating-point numbers
c = -3.14
d = -0.001
Negating complex numbers
e = -2 + 3j
“`
In these examples, `a` and `b` represent negative integers, `c` and `d` represent negative floats, and `e` is a negative complex number.
Negating Variables
You can also negate variables that already hold values. Here’s how this can be done:
“`python
x = 15
y = -x y will be -15
z = -x + 10 z will be -15 + 10 = -5
“`
This demonstrates that the negation operator can be dynamically applied to variables.
Negating Expressions
The negation operator can also be used within more complex expressions. For example:
“`python
result = -(3 * 4 + 5) result will be -17
“`
In this case, the entire expression inside the parentheses is evaluated first, and then the negation is applied.
Common Use Cases
The negation operator is commonly used in various scenarios, including:
- Mathematical calculations: Adjusting values in equations.
- Control structures: Inverting conditions in if statements.
- Data manipulation: Flipping signs of elements in data structures, such as lists or arrays.
Example: Negating Values in a List
You may want to negate all the values in a list. This can be efficiently accomplished using a list comprehension:
“`python
numbers = [1, -2, 3, -4]
negated_numbers = [-num for num in numbers] Results in [-1, 2, -3, 4]
“`
This technique is powerful for transforming data sets.
Using the Negation in Functions
Functions can also utilize the negation operator to return negative values based on input. Below is an example function:
“`python
def make_negative(number):
return -abs(number)
print(make_negative(10)) Output: -10
“`
This function ensures that the output is always negative, regardless of the input.
The negation operator in Python is a straightforward yet powerful tool for manipulating numerical values. By understanding its syntax and application, you can enhance your code’s functionality and readability effectively.
Expert Insights on Writing Functions in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When writing functions in Python, clarity and simplicity should be your guiding principles. Use meaningful names for your functions and parameters to enhance readability, and always include docstrings to describe their purpose and usage.”
Michael Chen (Python Developer, CodeCraft Solutions). “In Python, leveraging built-in functions and libraries can significantly reduce the amount of code you need to write. Familiarize yourself with the standard library, as it provides a wealth of tools that can simplify complex tasks.”
Sarah Patel (Data Scientist, Analytics Hub). “Modular programming is essential in Python. Break your code into smaller, reusable functions to improve maintainability and testing. This approach not only enhances code organization but also facilitates collaboration in team environments.”
Frequently Asked Questions (FAQs)
How do I write a negative number in Python?
You can write a negative number in Python by simply placing a minus sign (-) before the number. For example, `-5` represents negative five.
What is the syntax for negating a variable in Python?
To negate a variable in Python, use the minus sign before the variable name. For instance, if `x = 10`, then `-x` would evaluate to `-10`.
How can I subtract a number from another in Python?
You can subtract a number from another using the minus operator (-). For example, `result = a – b` subtracts `b` from `a`.
Is there a built-in function to negate numbers in Python?
Python does not have a specific built-in function to negate numbers, but you can use the unary minus operator (-) to achieve this.
Can I use the `abs()` function to write a negative number?
The `abs()` function returns the absolute value of a number, which is always non-negative. To write a negative number, you should use the minus sign instead.
How do I handle negative numbers in Python lists?
You can include negative numbers in Python lists just like positive numbers. For example, `my_list = [-1, -2, 3, 4]` is a valid list containing negative and positive integers.
In Python, writing the expression `-x` is straightforward and involves using the unary negation operator. This operator is applied directly to the variable `x`, effectively negating its value. For instance, if `x` holds a positive integer, `-x` will yield a negative integer of the same magnitude. This operation can be performed on various data types, including integers, floats, and even complex numbers, making it a versatile feature of the language.
Moreover, understanding how to utilize the negation operator is essential for various programming tasks, such as mathematical computations, conditional statements, and data manipulation. It allows developers to easily reverse the sign of a number, which is particularly useful in algorithms that require adjustments to values based on certain conditions. Additionally, Python’s dynamic typing means that the same negation operation can be applied without concern for the underlying data type, promoting flexibility in coding practices.
the ability to write `-x` in Python is a fundamental aspect of the language that enhances its expressiveness and functionality. By mastering this simple yet powerful operator, programmers can effectively manage numerical values and implement more complex logic in their applications. Embracing such basic operations is crucial for anyone looking to develop proficiency in
Author Profile
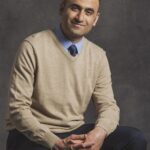
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?