Why Am I Seeing ‘Module Not Found: Can’t Resolve ‘fs’?’ and How Can I Fix It?
In the world of JavaScript development, encountering errors is an inevitable part of the journey, especially when working with Node.js or frameworks that utilize it. One of the most perplexing messages developers often face is the dreaded “module not found can’t resolve ‘fs’.” This error can halt progress and leave even seasoned programmers scratching their heads. But fear not! Understanding the root causes and solutions to this issue can empower you to navigate your projects with confidence and clarity. In this article, we will delve into the intricacies of the ‘fs’ module, explore common pitfalls that lead to this error, and equip you with the knowledge to resolve it effectively.
The ‘fs’ module, short for “file system,” is a core component of Node.js that allows developers to interact with the file system on a server. However, when working in environments like front-end frameworks or bundlers, the reliance on Node.js modules can lead to compatibility issues. The error message often signifies that the environment does not recognize the ‘fs’ module, which can stem from various reasons, including misconfiguration or the limitations of the platform being used.
As we unpack this topic, we’ll examine scenarios where the ‘fs’ module is essential, the contexts in which it may not be available, and
Understanding the Error
The error message `module not found can’t resolve ‘fs’` typically occurs in JavaScript environments, particularly when using Node.js modules in contexts where they are not supported, such as in the browser. The `fs` module, which stands for “file system,” is a core Node.js module that allows developers to interact with the file system on the server side. It cannot be utilized in client-side JavaScript, leading to the aforementioned error.
Common Scenarios Leading to the Error
Several situations can trigger this error:
- Incorrect Environment: Attempting to use Node.js-specific modules like `fs` in a browser context where they are unavailable.
- Bundler Misconfiguration: Using bundlers such as Webpack or Parcel without proper configuration can lead to this issue, as they may not recognize `fs` as a valid module.
- Dependency Issues: A misconfigured or outdated package that relies on `fs` might not compile correctly for the target environment.
Resolving the Error
To resolve the `module not found can’t resolve ‘fs’` error, consider the following solutions:
- Check the Environment: Ensure that the code utilizing the `fs` module is executed in a Node.js environment. If you need file system access in the browser, consider using alternative methods such as Web APIs.
- Use Conditional Imports: If you have a mixed codebase that targets both Node.js and browser environments, employ conditional imports to load the `fs` module only in Node.js.
- Adjust Webpack Configuration: If using Webpack, modify the configuration to exclude `fs` or provide a fallback. Here’s a basic example of how to configure Webpack:
“`javascript
resolve: {
fallback: {
fs:
}
}
“`
- Explore Alternatives: For operations requiring file handling on the client side, consider libraries like `browserify-fs` or use browser storage options such as `localStorage` or `IndexedDB`.
Practical Example
Suppose you need to read a file in a Node.js application using the `fs` module. Here’s a basic example of how this is done:
“`javascript
const fs = require(‘fs’);
fs.readFile(‘example.txt’, ‘utf8’, (err, data) => {
if (err) {
console.error(‘Error reading file:’, err);
return;
}
console.log(data);
});
“`
In contrast, if you attempt to run the above in a browser environment, you would encounter the `module not found can’t resolve ‘fs’` error.
Alternative Solutions
If the goal is to interact with files in a browser context, consider the following alternatives:
Library/Method | Description |
---|---|
File API | Allows web applications to read and manipulate files selected by users through input elements. |
IndexedDB | A low-level API for client-side storage of significant amounts of structured data. |
localStorage | Provides a simple synchronous key-value store for the web browser. |
browserify-fs | A module that simulates the `fs` API in the browser using IndexedDB. |
By selecting the appropriate solution based on your development environment, you can effectively avoid the `module not found can’t resolve ‘fs’` error while achieving your desired functionality.
Understanding the ‘fs’ Module in Node.js
The ‘fs’ module in Node.js provides an API for interacting with the file system. It allows developers to perform various operations such as reading, writing, and deleting files. This module is essential for server-side applications that need to handle file operations.
Key functionalities of the ‘fs’ module include:
- Reading Files: Use methods like `fs.readFile()` for asynchronous file reading.
- Writing Files: `fs.writeFile()` allows you to create and modify files.
- File Statistics: `fs.stat()` retrieves information about files, such as size and modification date.
- Directory Operations: Create and manage directories using methods like `fs.mkdir()` and `fs.readdir()`.
Common Causes of ‘Module Not Found’ Errors
The error message “module not found can’t resolve ‘fs'” typically indicates a problem with module resolution in your Node.js application. Several factors can lead to this error:
- Incorrect Environment: The ‘fs’ module is a Node.js built-in module and is not available in browser environments. Ensure your code is executed in a Node.js context.
- Typographical Errors: Check for spelling mistakes in the require statement, such as `require(‘fs’)`.
- Module Path Issues: Ensure that the module is not being referenced incorrectly, especially if it’s used in a bundler setup like Webpack.
- Bundler Configuration: If you’re using a bundler, make sure it is configured correctly to handle Node.js built-in modules.
Resolving the ‘fs’ Module Not Found Error
To troubleshoot and resolve the “module not found can’t resolve ‘fs'” error, consider the following steps:
- Check Environment: Ensure that your code is running in Node.js and not in a browser environment.
- Review Code: Verify that the require statement is correctly written:
“`javascript
const fs = require(‘fs’);
“`
- Inspect Bundler Configurations: If using Webpack or another bundler, adjust your configuration:
- For Webpack, ensure that the `target` is set to `node`.
- Use the `node` configuration option to include built-in modules.
“`javascript
module.exports = {
target: ‘node’,
// other configuration options
};
“`
- Check Dependencies: Ensure that there are no conflicting dependencies that might affect module resolution.
Alternatives to the ‘fs’ Module in Frontend Development
If your project requires file system interactions in a frontend context, consider using alternative APIs or libraries since ‘fs’ is not available in browsers:
Alternative | Description |
---|---|
File API | Allows reading files from user inputs (e.g., ``). |
Blob API | Enables creating and manipulating file-like objects in memory. |
IndexedDB | A low-level API for storing significant amounts of structured data. |
By using the above alternatives, developers can work with files in a manner suited for client-side applications, avoiding the limitations associated with the ‘fs’ module.
Understanding the ‘Module Not Found’ Error in Node.js
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘module not found can’t resolve ‘fs” error typically arises when a developer attempts to use Node.js built-in modules in a browser environment. Since ‘fs’ is a file system module exclusive to Node.js, it cannot be accessed in client-side JavaScript.”
Mark Thompson (Lead Backend Developer, CodeCraft Solutions). “To resolve the ‘fs’ module error, ensure that your code is running in a Node.js environment. If you are using a bundler like Webpack, you may need to configure it to handle Node.js modules appropriately.”
Linda Chen (Technical Consultant, DevOps Experts). “If you encounter the ‘module not found’ error for ‘fs’, check your project structure and dependencies. It is crucial to verify that your Node.js version supports the features you are trying to implement and that the environment is correctly set up.”
Frequently Asked Questions (FAQs)
What does the error “module not found can’t resolve ‘fs'” mean?
This error indicates that the Node.js ‘fs’ (File System) module is not available in the context where you are trying to use it, typically when running code in a browser environment instead of a Node.js environment.
Why am I getting this error in a React application?
React applications are typically executed in the browser, which does not support Node.js core modules like ‘fs’. Therefore, attempting to import ‘fs’ in a React component will result in this error.
How can I resolve the “module not found can’t resolve ‘fs'” error?
To resolve this error, avoid using the ‘fs’ module in client-side code. If you need file system access, consider using server-side code or APIs that handle file operations.
Are there alternatives to using ‘fs’ in a browser environment?
Yes, for file operations in the browser, you can use the File API, Blob, or libraries like FileSaver.js that allow you to handle files without needing ‘fs’.
Can I use ‘fs’ in server-side code with Node.js?
Yes, the ‘fs’ module can be used in server-side code with Node.js. Ensure that your code is executed in a Node.js environment to access the ‘fs’ functionalities.
What should I do if I need to access files both in the browser and Node.js?
Consider using a universal approach by creating separate modules for server-side and client-side code. Use ‘fs’ for server-side operations and browser-compatible methods for client-side file handling.
The error message “module not found can’t resolve ‘fs'” typically arises in Node.js or JavaScript environments when the ‘fs’ (file system) module is not accessible in the context where it is being used. This module is a core part of Node.js, designed for file manipulation tasks. However, it is not available in browser environments, which can lead to confusion for developers transitioning between server-side and client-side code.
Understanding the context of your code is crucial. If you encounter this error while running JavaScript in a browser, it is essential to recognize that the ‘fs’ module is not supported in that environment. To resolve this, developers must either run their code in a Node.js environment or utilize alternative methods for file handling, such as using the File API or libraries specifically designed for client-side file operations.
Another key takeaway is the importance of environment management in JavaScript development. Developers should be aware of the distinctions between Node.js and browser environments, as well as the modules available in each. Properly structuring code to separate server-side logic from client-side logic can prevent such errors and enhance the overall development process.
Author Profile
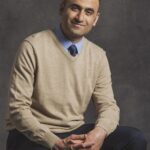
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?