How Can You Effectively Use a Nested Case Statement in SQL?
In the world of SQL, data manipulation and retrieval can often feel like navigating a complex maze. As databases grow in size and complexity, so too does the need for sophisticated querying techniques. One such technique that stands out for its versatility and power is the nested case statement. This advanced SQL feature allows developers to handle intricate logic and conditional expressions, enabling them to derive meaningful insights from their data with precision and clarity. Whether you’re a seasoned database administrator or a budding data analyst, understanding how to effectively utilize nested case statements can elevate your SQL skills to new heights.
At its core, a nested case statement is a tool that allows you to evaluate multiple conditions and return specific values based on those evaluations. By embedding one case statement within another, you can create a hierarchy of conditions that can address complex scenarios in your data. This capability is particularly useful in situations where data needs to be categorized or transformed based on multiple criteria, allowing for more nuanced queries that go beyond simple comparisons.
As you delve deeper into the intricacies of nested case statements, you’ll discover their potential to streamline your SQL queries and enhance the readability of your code. From simplifying complex logic to improving performance, mastering this technique can significantly impact how you interact with your data. In the following sections, we will explore practical examples and best
Understanding Nested Case Statements
Nested CASE statements in SQL allow for complex conditional logic by embedding one CASE statement within another. This structure is particularly useful when multiple layers of conditions need to be evaluated. The syntax follows a straightforward pattern, where each CASE statement can contain one or more WHEN clauses, and each WHEN clause can include its own set of nested CASE statements.
The basic syntax for a nested CASE statement is as follows:
“`sql
CASE
WHEN condition1 THEN
CASE
WHEN nested_condition1 THEN result1
WHEN nested_condition2 THEN result2
ELSE nested_default_result
END
WHEN condition2 THEN result2
ELSE default_result
END
“`
This example illustrates how to evaluate conditions and return results based on those evaluations. The outer CASE checks for `condition1` and `condition2`, while the inner CASE evaluates `nested_condition1` and `nested_condition2`.
Practical Example of Nested CASE Statements
To better understand how nested CASE statements function, consider a scenario involving employee performance ratings. The organization categorizes performance into three levels: “Poor,” “Average,” and “Excellent.” Each level may further depend on various criteria such as sales performance and customer feedback.
Here is a SQL example that demonstrates this concept:
“`sql
SELECT employee_id,
employee_name,
CASE
WHEN sales > 100000 THEN
CASE
WHEN feedback_score >= 4 THEN ‘Excellent’
WHEN feedback_score = 3 THEN ‘Average’
ELSE ‘Poor’
END
WHEN sales BETWEEN 50000 AND 100000 THEN ‘Average’
ELSE ‘Poor’
END AS performance_rating
FROM employees;
“`
In this query:
- The outer CASE evaluates the `sales` figure.
- If sales exceed 100,000, the inner CASE checks the `feedback_score` to determine if the performance is “Excellent,” “Average,” or “Poor.”
- For sales between 50,000 and 100,000, the performance is directly rated as “Average.”
- If sales fall below 50,000, the performance is rated as “Poor.”
Advantages of Using Nested CASE Statements
Utilizing nested CASE statements in SQL offers several advantages:
- Enhanced Logic: Complex decision-making can be implemented directly within SQL queries.
- Readability: When structured correctly, nested CASE statements can make queries more intuitive.
- Flexibility: Allows for multiple levels of evaluation and categorization, accommodating varied business rules.
Common Use Cases
Nested CASE statements can be applied in various scenarios, including but not limited to:
- Data Classification: Classifying numerical values into different categories based on specific thresholds.
- Conditional Formatting: Adjusting output formats based on certain criteria, such as displaying different messages or formats in reports.
- Aggregating Data: Summarizing data points based on complex conditions for reporting purposes.
Performance Level | Sales Threshold | Feedback Score |
---|---|---|
Excellent | Sales > 100,000 | Score >= 4 |
Average | Sales > 100,000 | Score = 3 |
Poor | Sales <= 100,000 | Any Score |
By employing nested CASE statements appropriately, SQL users can efficiently handle intricate logic within their queries, leading to more powerful data manipulation and analysis capabilities.
Understanding Nested CASE Statements
Nested CASE statements in SQL allow for more complex conditional logic by embedding one CASE statement within another. This approach enables the handling of multiple conditions and scenarios efficiently within a single query.
Structure of a Nested CASE Statement
The basic syntax for a nested CASE statement is as follows:
“`sql
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN
CASE
WHEN nested_condition1 THEN nested_result1
WHEN nested_condition2 THEN nested_result2
ELSE nested_default_result
END
ELSE default_result
END
“`
Example of a Nested CASE Statement
Consider a scenario where you need to categorize employees based on their performance ratings and years of service. A nested CASE statement can be structured as follows:
“`sql
SELECT employee_id,
performance_rating,
years_of_service,
CASE
WHEN performance_rating = ‘Excellent’ THEN
CASE
WHEN years_of_service > 5 THEN ‘Top Performer’
ELSE ‘Valuable Contributor’
END
WHEN performance_rating = ‘Good’ THEN ‘Meets Expectations’
ELSE ‘Needs Improvement’
END AS performance_category
FROM employees;
“`
Key Points to Remember
- Readability: While nested CASE statements can simplify complex logic, they may reduce readability if overused. Aim for clarity.
- Performance: Excessive nesting may impact performance. Always consider the execution plan when using complex structures.
- Limitations: SQL Server limits the number of nested CASE statements, which is generally around 10 levels deep. Be mindful of this constraint.
Practical Use Cases
Nested CASE statements are particularly useful in:
- Dynamic Reporting: Adjusting output based on various criteria for dashboards or reports.
- Data Transformation: Modifying data during selection based on multiple conditions.
- Conditional Aggregation: Aggregating data in various ways based on conditions.
Advantages of Nested CASE Statements
- Flexibility: They provide the ability to handle multiple layers of conditions in one statement.
- Conciseness: Reduces the need for multiple separate queries or joins for conditional logic.
- Clarity: In some cases, they can condense complex logic into a single, manageable expression.
Example Table for Clarity
Performance Rating | Years of Service | Performance Category |
---|---|---|
Excellent | 6 | Top Performer |
Excellent | 3 | Valuable Contributor |
Good | 4 | Meets Expectations |
Poor | 2 | Needs Improvement |
By leveraging nested CASE statements effectively, SQL developers can streamline their queries and enhance the logic within their database operations.
Expert Insights on Nested Case Statements in SQL
Dr. Emily Carter (Database Architect, Data Solutions Inc.). “Nested case statements in SQL are powerful tools for handling complex conditional logic within queries. They allow for multiple layers of decision-making, which can simplify the overall structure of your SQL code and enhance readability when used judiciously.”
James Liu (Senior SQL Developer, Tech Innovations Group). “Utilizing nested case statements can significantly improve data manipulation capabilities. However, developers must be cautious about performance implications, especially with large datasets, as excessive nesting can lead to slower query execution times.”
Maria Gonzalez (Business Intelligence Analyst, Insight Analytics). “Incorporating nested case statements is essential for generating complex reports that require multi-dimensional analysis. It enables analysts to derive insights from data by applying various conditions and aggregations within a single query.”
Frequently Asked Questions (FAQs)
What is a nested case statement in SQL?
A nested case statement in SQL refers to the use of one CASE statement inside another CASE statement. This allows for more complex conditional logic and enables the evaluation of multiple conditions in a hierarchical manner.
How do you structure a nested case statement?
A nested case statement is structured by placing one CASE statement inside the result expression of another CASE statement. The syntax involves defining the outer CASE first, followed by the inner CASE within one of its WHEN clauses.
Can you provide an example of a nested case statement?
Certainly. An example would be:
“`sql
SELECT
employee_id,
CASE
WHEN department = ‘Sales’ THEN
CASE
WHEN sales_amount > 100000 THEN ‘High Performer’
ELSE ‘Regular Performer’
END
ELSE ‘Non-Sales Department’
END AS performance_category
FROM employees;
“`
What are the benefits of using nested case statements?
The benefits include enhanced readability and organization of complex conditional logic, allowing for more granular control over the evaluation of conditions and the ability to return different results based on multiple criteria.
Are there any performance considerations with nested case statements?
Yes, performance can be affected if nested case statements become too complex or deep, as they may require more processing time. It is advisable to keep the logic as simple as possible and consider alternative approaches if performance issues arise.
Can nested case statements be used in all SQL databases?
Most modern SQL databases, including MySQL, SQL Server, Oracle, and PostgreSQL, support nested case statements. However, it’s essential to check the specific syntax and limitations of the database you are using.
A nested case statement in SQL is a powerful construct that allows for complex conditional logic within queries. By using multiple levels of CASE statements, developers can evaluate various conditions and return different results based on those evaluations. This capability is particularly useful in scenarios where multiple criteria must be assessed to determine the outcome, enabling more sophisticated data manipulation and reporting.
One of the key advantages of nested case statements is their flexibility. They can be used to handle intricate business rules directly within SQL queries, reducing the need for additional processing in application code. This can lead to improved performance and easier maintenance, as the logic is encapsulated within the database layer. Additionally, nested case statements can simplify queries by consolidating multiple conditional checks into a single structure, enhancing readability and organization.
However, it is essential to use nested case statements judiciously. Overly complex nesting can lead to queries that are difficult to read and maintain. Developers should strive for clarity and simplicity, ensuring that the logic remains understandable. Proper indentation and formatting can significantly improve the readability of nested case statements, making it easier for others to follow the logic and for future developers to make necessary adjustments.
In summary, nested case statements are a valuable tool in SQL for implementing complex conditional logic
Author Profile
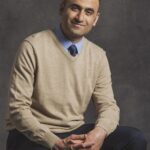
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?