How Can I Select the Most Recent Record in SQL?
In the world of data management, the ability to efficiently retrieve the most recent records from a database can be a game-changer for businesses and developers alike. Whether you’re analyzing user activity, tracking inventory, or generating reports, knowing how to select the latest entries is crucial for making informed decisions. SQL, or Structured Query Language, serves as the backbone for database interactions, providing powerful tools to sift through vast amounts of data with precision. In this article, we will delve into the techniques and best practices for selecting the most recent records in SQL, empowering you to harness your data effectively.
Selecting the most recent record in SQL is a fundamental task that can be approached in various ways, depending on the structure of your data and the specific requirements of your query. At its core, this process involves utilizing date or timestamp fields to filter results, ensuring that you are always working with the latest information available. Understanding the nuances of SQL functions and clauses, such as `ORDER BY`, `LIMIT`, and `GROUP BY`, can significantly enhance your ability to retrieve relevant records efficiently.
Moreover, the context in which you need the most recent data can vary widely, from real-time analytics to historical comparisons. This diversity necessitates a flexible approach to SQL queries, allowing you to adapt your methods based on the
Using SQL to Select the Most Recent Record
To select the most recent record from a dataset in SQL, you typically utilize the `ORDER BY` clause in conjunction with the `LIMIT` clause or window functions. The approach may vary slightly depending on the SQL dialect being used, but the general concepts remain consistent.
Basic Query Structure
The simplest method to retrieve the most recent record is to sort the results by the date field in descending order and limit the output to one record. Here’s a basic example using a hypothetical `orders` table:
“`sql
SELECT *
FROM orders
ORDER BY order_date DESC
LIMIT 1;
“`
In this example:
- `order_date` is the column representing the date of the order.
- The results are sorted in descending order, meaning the latest order appears first.
- The `LIMIT 1` clause restricts the output to only the most recent record.
Using Window Functions
In databases that support window functions, you can use the `ROW_NUMBER()` function to achieve more complex queries, especially if you want to retrieve the most recent record alongside other records from the same group. Here’s how it can be done:
“`sql
SELECT *
FROM (
SELECT *,
ROW_NUMBER() OVER (PARTITION BY customer_id ORDER BY order_date DESC) AS rn
FROM orders
) AS ranked_orders
WHERE rn = 1;
“`
In this query:
- `PARTITION BY customer_id` allows you to group results by customer, so each customer’s most recent order is identified.
- The `ORDER BY order_date DESC` ensures the most recent order is ranked first within each partition.
- The outer query filters to return only the records where `rn` (row number) equals 1.
Example Table Structure
To illustrate this with a sample table, consider the following structure for the `orders` table:
order_id | customer_id | order_date | amount |
---|---|---|---|
1 | A1 | 2023-01-15 | 100 |
2 | A2 | 2023-01-20 | 150 |
3 | A1 | 2023-02-10 | 200 |
4 | A2 | 2023-01-25 | 300 |
In this example:
- For customer `A1`, the most recent order is order ID `3` dated `2023-02-10`.
- For customer `A2`, the most recent order is order ID `2` dated `2023-01-20`.
Considerations
When selecting the most recent record, consider the following:
- Performance: Large datasets may require indexing on the date column to optimize query performance.
- Data Types: Ensure the date field is stored in a date or datetime format for accurate sorting.
- Null Values: Be aware of how your SQL dialect handles null values in date columns, as they can affect the results.
By applying these techniques, you can efficiently retrieve the most recent records from your SQL database.
Selecting the Most Recent Record in SQL
To retrieve the most recent record from a database table in SQL, you typically utilize the `ORDER BY` clause in conjunction with `LIMIT` or a similar function depending on the SQL dialect. The following are common approaches to achieve this across different database systems.
Using ORDER BY with LIMIT
This method is widely used in SQL databases such as MySQL and PostgreSQL. By sorting the records based on a timestamp or date column in descending order, you can easily limit the results to the most recent entry.
“`sql
SELECT *
FROM your_table
ORDER BY date_column DESC
LIMIT 1;
“`
- date_column: The column containing the date or timestamp.
- your_table: The name of the table from which you want to select records.
Using Common Table Expressions (CTEs)
Common Table Expressions can provide a more readable approach, especially when dealing with more complex queries. This method is particularly useful in SQL Server and PostgreSQL.
“`sql
WITH RecentRecords AS (
SELECT *,
ROW_NUMBER() OVER (ORDER BY date_column DESC) AS RowNum
FROM your_table
)
SELECT *
FROM RecentRecords
WHERE RowNum = 1;
“`
- ROW_NUMBER(): This function assigns a unique sequential integer to rows within a partition of a result set.
Using Subqueries
Subqueries can also be employed to select the most recent record. This is a versatile approach compatible with various SQL databases.
“`sql
SELECT *
FROM your_table
WHERE date_column = (
SELECT MAX(date_column)
FROM your_table
);
“`
- MAX(): This aggregate function returns the maximum value in the specified column.
Handling Ties in Date Values
When the most recent date may have multiple entries, deciding how to handle ties is essential. Here are options to consider:
- Select All Tied Records: If you want all records that share the maximum date:
“`sql
SELECT *
FROM your_table
WHERE date_column = (
SELECT MAX(date_column)
FROM your_table
);
“`
- Select One with Additional Criteria: If you prefer a single record, you can add further sorting criteria:
“`sql
SELECT *
FROM your_table
ORDER BY date_column DESC, another_column ASC
LIMIT 1;
“`
Database-Specific Functions
Certain databases offer specialized functions to simplify retrieving recent records. For example:
- SQL Server: You can use `TOP` instead of `LIMIT`.
“`sql
SELECT TOP 1 *
FROM your_table
ORDER BY date_column DESC;
“`
- Oracle: Utilize the `ROWNUM` function.
“`sql
SELECT *
FROM (
SELECT *
FROM your_table
ORDER BY date_column DESC
)
WHERE ROWNUM = 1;
“`
Example Table Structure
Here’s an example table structure to visualize the concepts discussed:
id | name | date_column |
---|---|---|
1 | Record A | 2023-10-01 10:00:00 |
2 | Record B | 2023-10-01 12:00:00 |
3 | Record C | 2023-10-02 09:00:00 |
4 | Record D | 2023-10-02 09:00:00 |
Using the provided SQL examples, you can effectively retrieve the most recent records from this structure.
Expert Insights on Selecting the Most Recent Record in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When selecting the most recent record in SQL, it is essential to utilize the `ORDER BY` clause in conjunction with the `LIMIT` statement. This approach ensures that you retrieve the latest entry based on a timestamp or an auto-incremented ID, providing accuracy and efficiency in data retrieval.”
James Patel (Senior SQL Developer, Data Solutions Group). “A common method for fetching the most recent record is to use a subquery that identifies the maximum value of the relevant date or timestamp column. This technique allows for precise targeting of the latest entry without unnecessary data processing, which is crucial in large datasets.”
Lisa Tran (Data Analyst, Insightful Analytics). “In scenarios where multiple records may share the same timestamp, employing the `ROW_NUMBER()` window function can be particularly advantageous. This function allows for the ranking of records, enabling you to select the most recent one while maintaining control over ties in the data.”
Frequently Asked Questions (FAQs)
How can I select the most recent record in SQL?
To select the most recent record in SQL, you can use the `ORDER BY` clause in combination with the `LIMIT` clause. For example:
“`sql
SELECT * FROM your_table ORDER BY date_column DESC LIMIT 1;
“`
What SQL function can I use to find the latest date?
You can use the `MAX()` function to find the latest date in a column. For example:
“`sql
SELECT MAX(date_column) FROM your_table;
“`
Can I select multiple recent records in SQL?
Yes, you can select multiple recent records by adjusting the `LIMIT` value. For instance, to select the top 5 recent records:
“`sql
SELECT * FROM your_table ORDER BY date_column DESC LIMIT 5;
“`
What if I need the most recent record for each group?
To obtain the most recent record for each group, you can use a subquery or the `ROW_NUMBER()` window function. For example:
“`sql
SELECT * FROM (
SELECT *, ROW_NUMBER() OVER (PARTITION BY group_column ORDER BY date_column DESC) as rn
FROM your_table
) AS subquery
WHERE rn = 1;
“`
Is there a difference between using `TOP` and `LIMIT` in SQL?
Yes, `TOP` is used in SQL Server to limit the number of records returned, while `LIMIT` is used in MySQL and PostgreSQL. The syntax differs, so ensure you use the appropriate one for your SQL dialect.
How do I handle ties when selecting the most recent record?
To handle ties, you can use additional criteria in your `ORDER BY` clause. For example:
“`sql
SELECT * FROM your_table ORDER BY date_column DESC, another_column DESC LIMIT 1;
“`
This ensures a consistent selection in case of identical dates.
In SQL, selecting the most recent record from a dataset is a common requirement that can be efficiently achieved using various techniques. The primary approach involves utilizing the `ORDER BY` clause alongside the `LIMIT` or `TOP` keywords, depending on the specific SQL dialect being used. By ordering the results based on a timestamp or date column in descending order, one can easily retrieve the latest entry. This method is straightforward and widely applicable across different database systems.
Another effective technique is to use subqueries or Common Table Expressions (CTEs) to isolate the most recent record based on specific criteria. This approach allows for more complex queries where additional filtering or aggregation is necessary. By leveraging these advanced SQL features, users can ensure they are accurately pinpointing the most relevant data while maintaining clarity in their queries.
It is also important to consider the implications of data integrity and consistency when retrieving recent records. In scenarios where multiple records share the same timestamp, additional criteria may be needed to determine which record should be selected. This can involve incorporating additional columns into the `ORDER BY` clause or using distinct identifiers to ensure the selection process is robust and reliable.
mastering the techniques for selecting the most recent record in SQL is essential
Author Profile
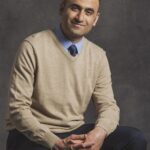
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?